filmov
tv
Why We Should Never Use gets()... And Why To Use fgets() Instead | C Programming Tutorial
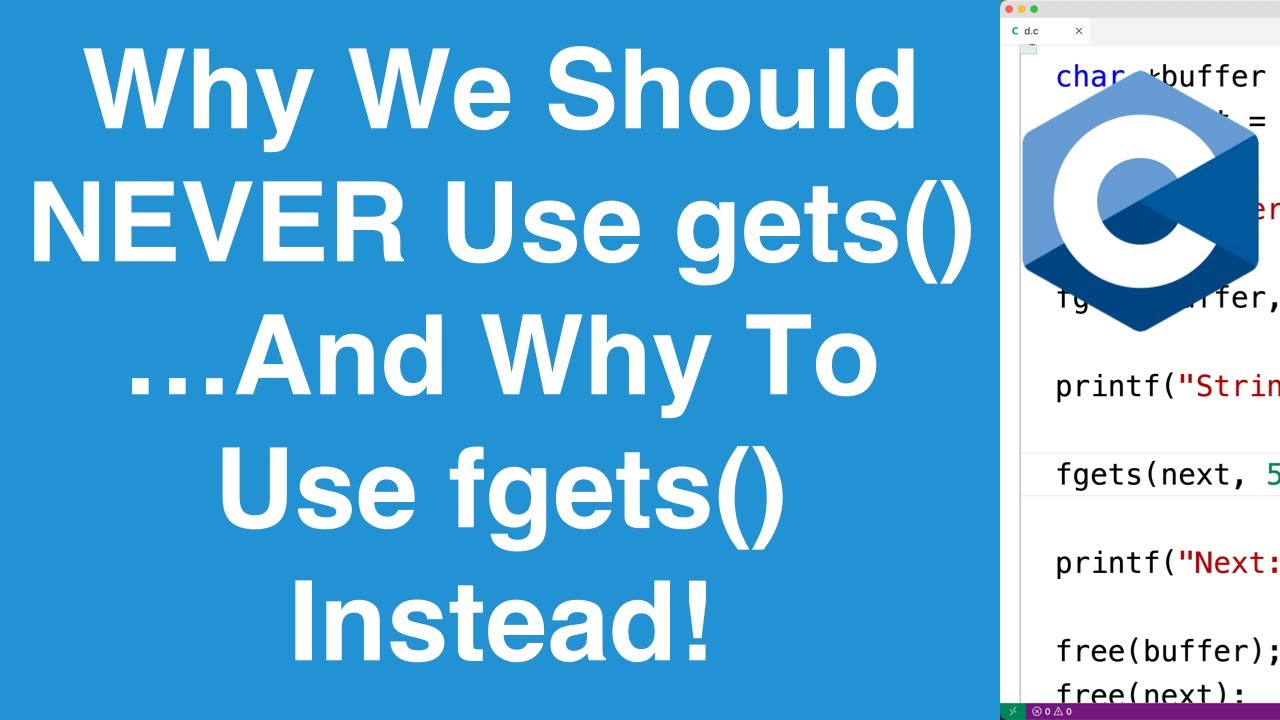
Показать описание
Things You Should NEVER Do In A Hospital
7 Things Parents Should NEVER DO To a Child
5 Things You Should Never Say To Someone With Depression
THAT'S WHY you should NEVER use Short Wash Programs💥(99% don't know it)🤯
8 Things You Should NEVER Say To Someone In Pain
7 Foods You Should Never Eat – Dr. Berg
This Is Why YOU Should Never Ever Eat Your Boogers (Animation)!
NEUROSCIENTIST: You Will NEVER Be Stressed Again | Andrew Huberman
7 Things You Should Never Say to Children
Why You Should Never Say 'It's Just A Theory'
The Part Of Your Body You Should NEVER Shave | RTC
Why You Should Never Use Bathroom Air Hand Dryers Again
10 Things You Should NEVER Say to Anyone - Matthew Kelly - 60 Second Wisdom
4 words you should never say to a narcissist
5 Pokemon You Should Never Use
12 Things You Should Never Flush Down the Toilet
Why You Should NEVER Check CAMERAS in Among Us 😨
Why You Should Never Visit The Dark Web
Most Dangerous Trees You Should NEVER Touch
This Is Why You Should Never Cut Your Pills in Half Unless They're Scored
10 Things You Should Never Say to Teens
Why We Should Never Stop Learning by Jay Shetty - Motivational Video
THIS Is Why You Should Never Use TikTok
Food for Life: 5 foods you should never eat
Комментарии