filmov
tv
Worked Exercise 3.1
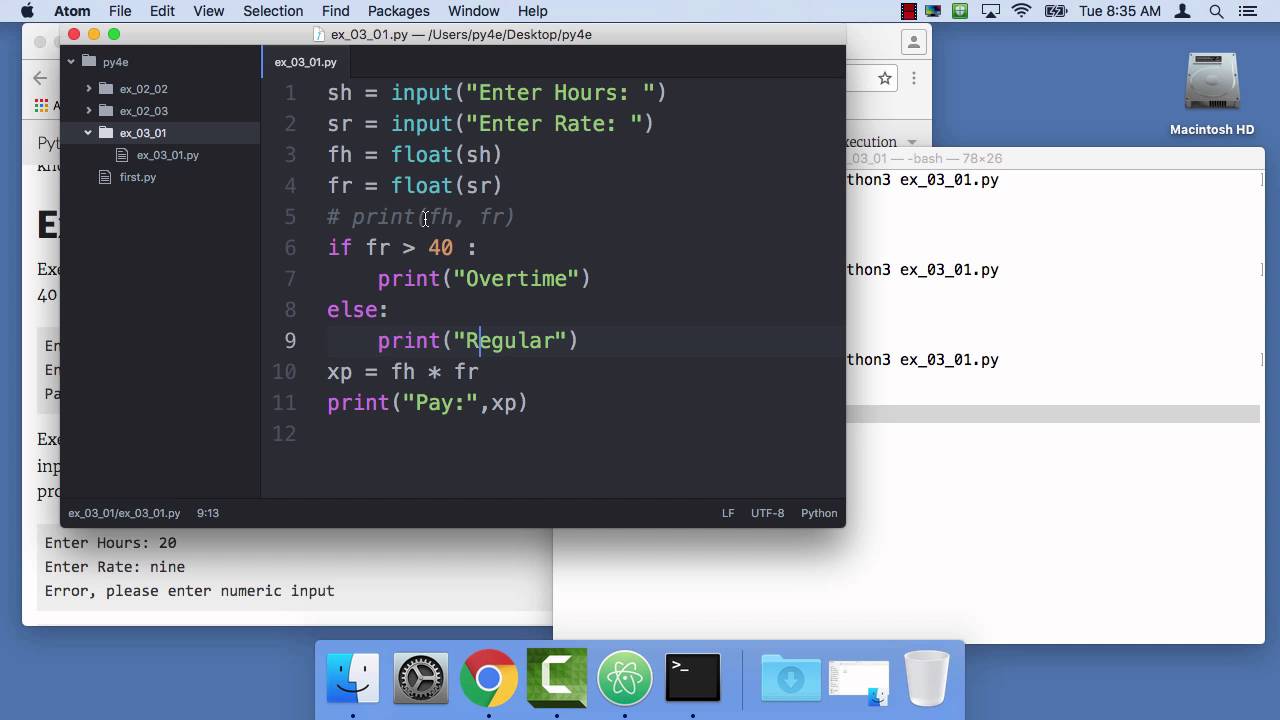
Показать описание
Please visit the web site to access a free textbook, free supporting materials, as well as interactive exercises.
Basic Jazz Conception for Saxophone by Lennie Niehaus (Vol. 1) - Exercise #3
The #1 Workout That BLEW UP My Back (3 Exercises)
Exercise 3.1 - Class 10 Math | Waqas Nasir
DAY 3 - 1 Month At Home Pilates Plan // 15MIN Hourglass Abs, Arms & Back // madeleineabeid
The 5-4-3-2-1 Method: A Grounding Exercise to Manage Anxiety
1 LIP EXERCISE 3 Levels of WEIGHT TRAINING your Upper Lip Area
DAY 1 OF 3 - BURN BELLY FAT - KIDS DAILY EXERCISE
Kids Beginner Exercise For Good Health
Abs Exercise Challenge: Partner-assisted Lower Abs Workout! Part 1
Kids Daily Exercise - Day 1
ONE JAW EXERCISE for THREE MINUTES per day to get a FIRM FACE
3-Step Piriformis Fix!
Class 9 Maths | Chapter 3 | Exercise 3.1 | Coordinate Geometry | NCERT
3 Day Challenge: Burn Fat and Calories - Kids Exercise
I wish I knew this before I started working out (3 things)
Kids Exercise - Kids Workout At Home
Post C-Section Exercise: WHEN To Start Exercising After C-Section (SERIES VIDEO 1)
The #1 Exercise That BLOWS UP Your Muscles - (FOLLOW ALONG)
3 ab exercises for a stronger core 💪 Exercise ball edition
30 Minute Morning Exercise Routine - Do This Every Day
5 Best Back Pain Exercises (for Quick and Long Lasting Relief)
3-Day Weight Loss Challenge: Daily Exercise To Burn Fat
Faça hoje mesmo 🤩 #atividadefisicaemcasa
21-Day Stand Only To Fix Hanging Belly!
Комментарии