filmov
tv
3 Ways To Flatten Any List In Python
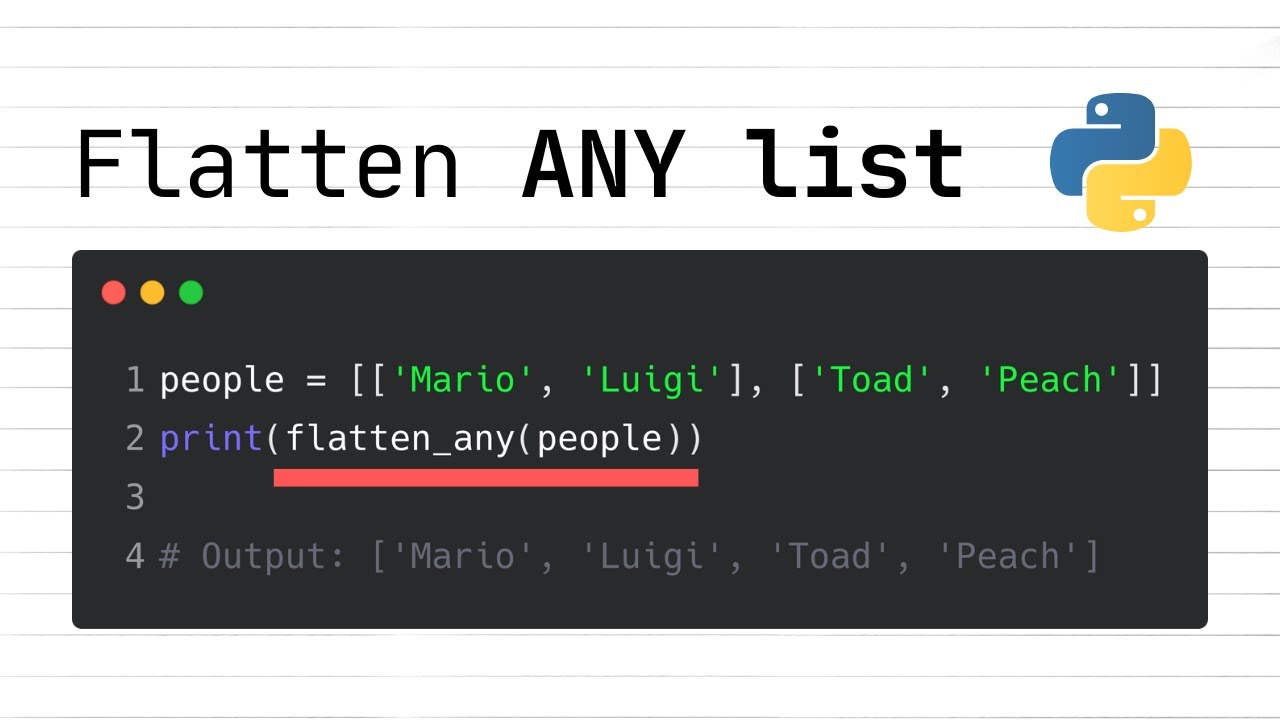
Показать описание
Learn how you can flatten any list in Python. We will be exploring 3 different ways to do this. First will be through vanilla Python with the for loop, then we will use the faster list comprehension approach, and finally we will use the easiest approach with numpy.
3 Ways To Flatten Any List In Python
Daily Blender Secrets - 3 ways to Flatten Faces
FLATTEN YOUR STOMACH with 1 easy trick! 🤯 #diy #satisfying #shorts
Three Ways To Flatten Beer And Soda Cans Into Metal Sheets
How To Flatten Autocad Drawing
3 WAYS TO FLATTEN A BOARD | Dave Stanton Live!
Flatten Your Stomach in 24 Hours! Dr. Mandell
Flatten Your Belly in 2 Moves! Dr. Mandell
How to flatten a board without a planer - A new different method for straightening boards
How to Flatten Cupped and Twisted Wood | ToolsToday
How to Flatten a Bench Top or Any Slab With Only Hand Tools
🧊 Skincare tip: How to flatten a cystic acne breakout for faster healing #shorts
Basic Ways to Flatten an Opponent Out by Gordon Ryan
Flatten Your Stomach For All Ages | Dr. Mandell
How to Flatten a Live Edge Wood Slab
How I flatten my knife sharpening stones | Knife sharpening tips 4
How to Flatten a Whetstone - Easiest/ Cheapest Method
How to Flatten Your Belly (FOR GOOD)
3 weeks to flatten the curve… 2 years later… what have we learnt??????
028 Cheap Way to Flatten a Board Without a Planer
3 Tips to Flatten Rug Corners | JONATHAN Y
How to flatten a board without a planer / jointer / thicknesser
3 Belly Fat Loss Drinks to Flatten Your Stomach #shorts
How To Flatten Your Stomach With 3 Exercises
Комментарии