filmov
tv
Check if Rectangles Overlap
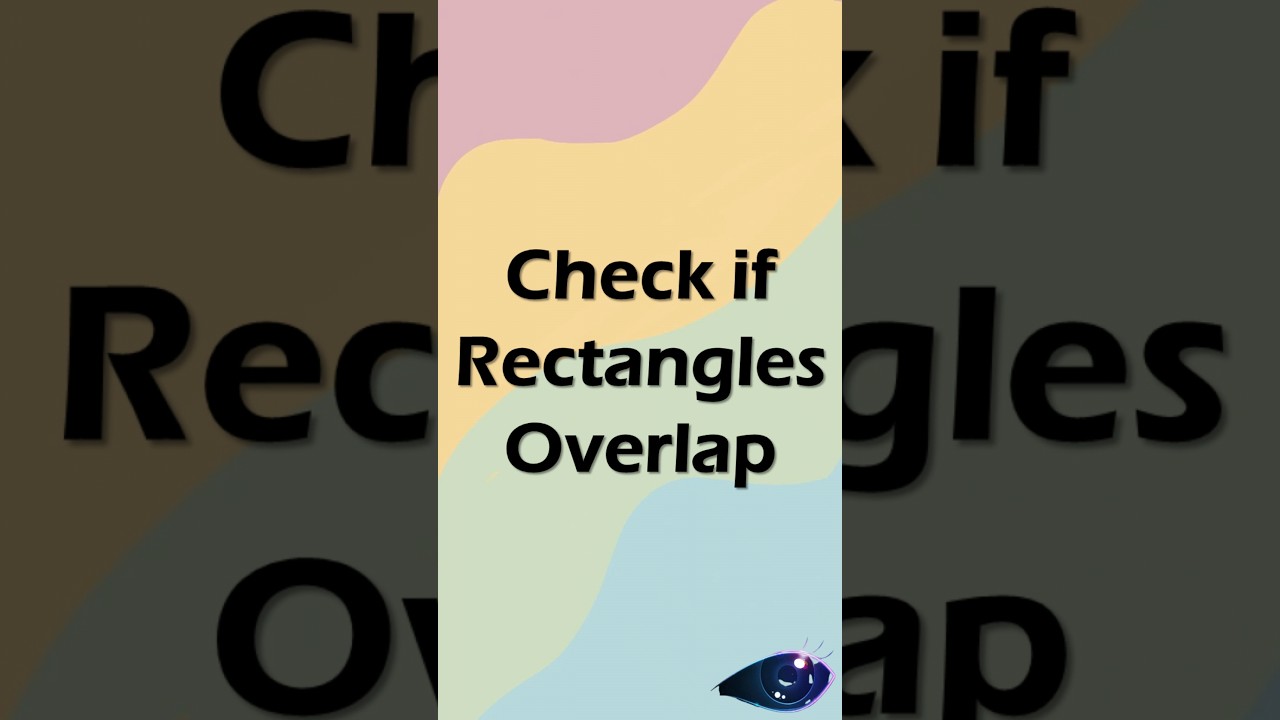
Показать описание
Given two rectangles, find if the given two rectangles overlap or not. A rectangle is denoted by providing the x and y coordinates of two points: the left top corner and the right bottom corner of the rectangle. Two rectangles sharing a side are considered overlapping. (L1 and R1 are the extreme points of the first rectangle and L2 and R2 are the extreme points of the second rectangle).
Note: It may be assumed that the rectangles are parallel to the coordinate axis. using python without def function
Here's a Python code snippet that checks if two rectangles overlap:
python
Copy code
# Input the coordinates of the first rectangle
L1 = tuple(map(int, input().split()))
R1 = tuple(map(int, input().split()))
# Input the coordinates of the second rectangle
L2 = tuple(map(int, input().split()))
R2 = tuple(map(int, input().split()))
# Check if the rectangles overlap
if R1[0] less than L2[0] or R2[0] less than L1[0]:
print("The rectangles do not overlap")
elif R1[1] less than L2[1] or R2[1] less than L1[1]:
print("The rectangles do not overlap")
else:
print("The rectangles overlap")
The code reads the coordinates of the two rectangles from the user input, and then checks if they overlap. The condition for overlap is that the two rectangles must not be disjoint along either the x-axis or the y-axis. If the rectangles overlap, the code prints a message indicating that they overlap. Otherwise, it prints a message indicating that they do not overlap
Note: It may be assumed that the rectangles are parallel to the coordinate axis. using python without def function
Here's a Python code snippet that checks if two rectangles overlap:
python
Copy code
# Input the coordinates of the first rectangle
L1 = tuple(map(int, input().split()))
R1 = tuple(map(int, input().split()))
# Input the coordinates of the second rectangle
L2 = tuple(map(int, input().split()))
R2 = tuple(map(int, input().split()))
# Check if the rectangles overlap
if R1[0] less than L2[0] or R2[0] less than L1[0]:
print("The rectangles do not overlap")
elif R1[1] less than L2[1] or R2[1] less than L1[1]:
print("The rectangles do not overlap")
else:
print("The rectangles overlap")
The code reads the coordinates of the two rectangles from the user input, and then checks if they overlap. The condition for overlap is that the two rectangles must not be disjoint along either the x-axis or the y-axis. If the rectangles overlap, the code prints a message indicating that they overlap. Otherwise, it prints a message indicating that they do not overlap