filmov
tv
How to Convert JSON UTF-8 Encoded Strings in an NSArray to Characters in iOS
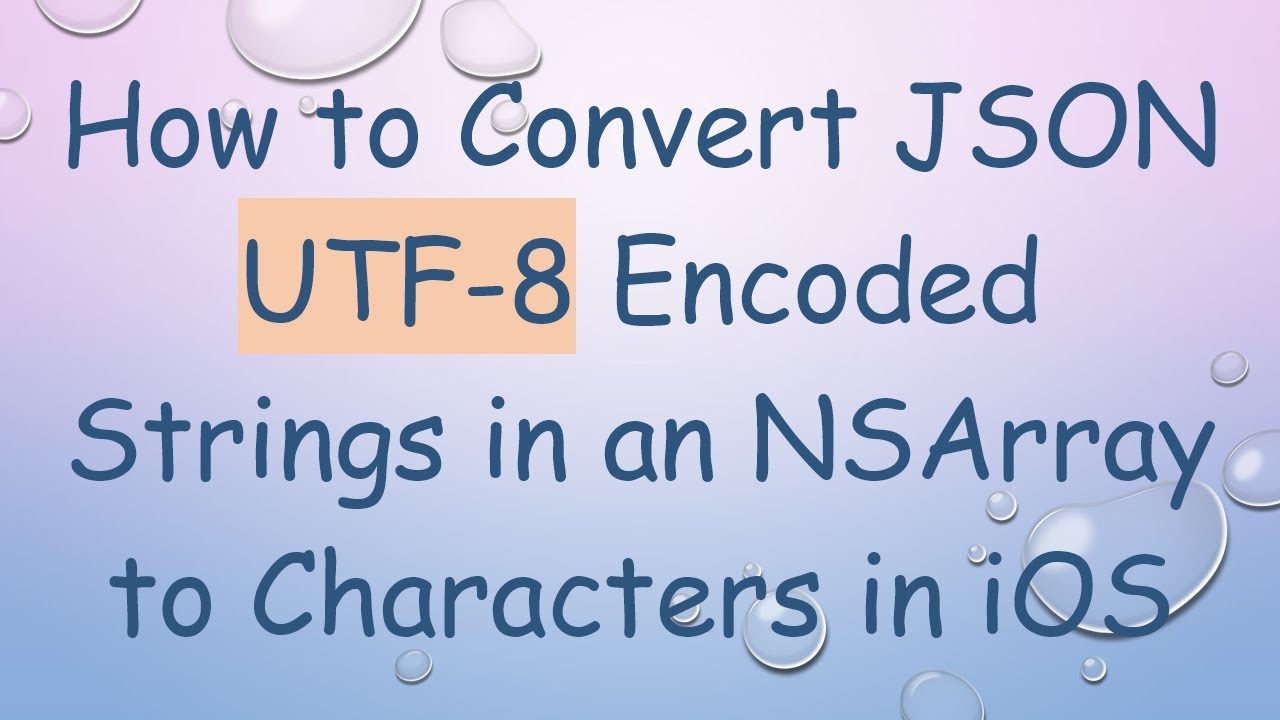
Показать описание
Summary: Learn how to efficiently convert JSON `UTF-8` encoded strings contained in an NSArray to readable characters in iOS using native Swift or Objective-C features.
---
Disclaimer/Disclosure - Portions of this content were created using Generative AI tools, which may result in inaccuracies or misleading information in the video. Please keep this in mind before making any decisions or taking any actions based on the content. If you have any concerns, don't hesitate to leave a comment. Thanks.
---
JSON, short for JavaScript Object Notation, is a lightweight format commonly used for data interchange. While working with JSON data in iOS applications, developers frequently encounter UTF-8 encoded strings. When dealing with arrays, especially NSArrays in iOS, the task of converting these encoded strings to actual characters can initially seem daunting, but it is quite manageable with the right approach.
Understanding UTF-8 Encoding
UTF-8 is a character encoding capable of encoding all possible characters in Unicode. It avoids the pitfalls of older encoding formats by being both space-efficient and consistent in how it handles bytes across various platforms. The challenge arises when you need to transform those seemingly jumbled sequences into user-readable characters.
Converting UTF-8 Strings in an NSArray
When processing JSON within an iOS application, JSON data is typically received as UTF-8 encoded text. To provide a seamless experience, you should convert these strings into a format that displays correctly within your app.
Using Swift
Swift offers a robust set of tools to handle string conversions effectively:
[[See Video to Reveal this Text or Code Snippet]]
In this approach:
JSONSerialization is used to parse JSON data received from a remote server or file, transforming it into a native array.
We utilize compactMap to process each string individually by first converting it into Data using UTF-8.
The String initializer helps convert the Data object back to a readable string.
Utilizing Objective-C
For those who prefer Objective-C, the procedure involves slightly different syntax:
[[See Video to Reveal this Text or Code Snippet]]
Here, you:
Parse the JSON data to an NSArray instance using NSJSONSerialization.
Loop through the array, converting each string using NSData and creating a new decoded string using NSString.
Considerations
Error Handling: Always ensure you have appropriate error handling, especially when dealing with JSON parsing, to avoid crashes in your application.
Data Integrity: Validate the NSArray content to confirm it comprises strings before conversion, preventing unexpected behaviors.
Conclusion
Converting JSON UTF-8 encoded strings within an NSArray to readable characters in iOS can be straightforward with the application of Swift or Objective-C built-in capabilities. By following the patterns above, you can render JSON data into a format that users can easily comprehend, thereby enhancing your app's interactivity and user experience.
---
Disclaimer/Disclosure - Portions of this content were created using Generative AI tools, which may result in inaccuracies or misleading information in the video. Please keep this in mind before making any decisions or taking any actions based on the content. If you have any concerns, don't hesitate to leave a comment. Thanks.
---
JSON, short for JavaScript Object Notation, is a lightweight format commonly used for data interchange. While working with JSON data in iOS applications, developers frequently encounter UTF-8 encoded strings. When dealing with arrays, especially NSArrays in iOS, the task of converting these encoded strings to actual characters can initially seem daunting, but it is quite manageable with the right approach.
Understanding UTF-8 Encoding
UTF-8 is a character encoding capable of encoding all possible characters in Unicode. It avoids the pitfalls of older encoding formats by being both space-efficient and consistent in how it handles bytes across various platforms. The challenge arises when you need to transform those seemingly jumbled sequences into user-readable characters.
Converting UTF-8 Strings in an NSArray
When processing JSON within an iOS application, JSON data is typically received as UTF-8 encoded text. To provide a seamless experience, you should convert these strings into a format that displays correctly within your app.
Using Swift
Swift offers a robust set of tools to handle string conversions effectively:
[[See Video to Reveal this Text or Code Snippet]]
In this approach:
JSONSerialization is used to parse JSON data received from a remote server or file, transforming it into a native array.
We utilize compactMap to process each string individually by first converting it into Data using UTF-8.
The String initializer helps convert the Data object back to a readable string.
Utilizing Objective-C
For those who prefer Objective-C, the procedure involves slightly different syntax:
[[See Video to Reveal this Text or Code Snippet]]
Here, you:
Parse the JSON data to an NSArray instance using NSJSONSerialization.
Loop through the array, converting each string using NSData and creating a new decoded string using NSString.
Considerations
Error Handling: Always ensure you have appropriate error handling, especially when dealing with JSON parsing, to avoid crashes in your application.
Data Integrity: Validate the NSArray content to confirm it comprises strings before conversion, preventing unexpected behaviors.
Conclusion
Converting JSON UTF-8 encoded strings within an NSArray to readable characters in iOS can be straightforward with the application of Swift or Objective-C built-in capabilities. By following the patterns above, you can render JSON data into a format that users can easily comprehend, thereby enhancing your app's interactivity and user experience.