filmov
tv
Converting UTF-8 Strings to JSON in Python
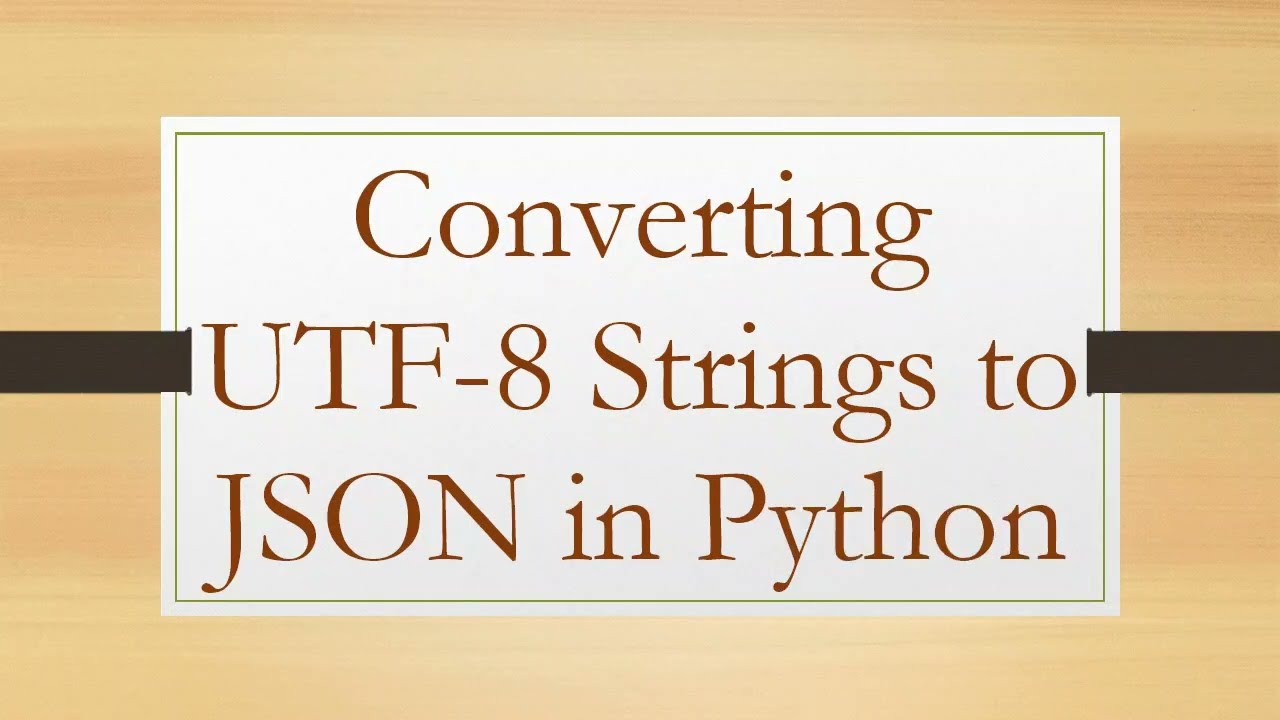
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Learn how to efficiently convert UTF-8 encoded strings to JSON in Python. Explore methods and best practices for handling character encodings in your data transformations.
---
When working with data in Python, you may come across situations where you need to convert UTF-8 encoded strings to JSON format. This process involves decoding the UTF-8 strings and then parsing them into a JSON structure. In this guide, we'll explore the steps to achieve this and discuss some best practices.
Understanding UTF-8 Encoding
UTF-8 is a widely-used character encoding that represents each character in the Unicode character set using a variable number of bytes. When dealing with UTF-8 encoded strings, it's important to decode them properly to work with the actual text data.
Decoding UTF-8 Strings in Python
Python provides a straightforward way to decode UTF-8 strings using the decode method. Here's a simple example:
[[See Video to Reveal this Text or Code Snippet]]
In this example, the decode method is called on the UTF-8 encoded string, specifying the encoding as 'utf-8'. The result, decoded_string, now contains the text representation of the original UTF-8 encoded data.
Converting to JSON
Once you have decoded the UTF-8 string, the next step is to convert it to a JSON format. Python's json module comes in handy for this task. Here's an example:
[[See Video to Reveal this Text or Code Snippet]]
In this snippet, the loads function from the json module is used to parse the decoded string into a JSON object. Now, json_data contains the data in a structured JSON format.
Handling Potential Issues
When working with character encodings, it's crucial to handle potential exceptions that may arise during decoding. You can use a try-except block to catch and address such issues:
[[See Video to Reveal this Text or Code Snippet]]
This approach helps you identify and handle decoding or JSON parsing errors gracefully.
Conclusion
Converting UTF-8 strings to JSON in Python involves decoding the strings using the appropriate method and then parsing them into a JSON format using the json module. Understanding character encodings and handling potential issues is crucial for robust data transformations.
In your Python projects, be mindful of the data encoding, and follow these steps to seamlessly convert UTF-8 encoded strings to JSON, ensuring that your data processing is accurate and reliable.
---
Summary: Learn how to efficiently convert UTF-8 encoded strings to JSON in Python. Explore methods and best practices for handling character encodings in your data transformations.
---
When working with data in Python, you may come across situations where you need to convert UTF-8 encoded strings to JSON format. This process involves decoding the UTF-8 strings and then parsing them into a JSON structure. In this guide, we'll explore the steps to achieve this and discuss some best practices.
Understanding UTF-8 Encoding
UTF-8 is a widely-used character encoding that represents each character in the Unicode character set using a variable number of bytes. When dealing with UTF-8 encoded strings, it's important to decode them properly to work with the actual text data.
Decoding UTF-8 Strings in Python
Python provides a straightforward way to decode UTF-8 strings using the decode method. Here's a simple example:
[[See Video to Reveal this Text or Code Snippet]]
In this example, the decode method is called on the UTF-8 encoded string, specifying the encoding as 'utf-8'. The result, decoded_string, now contains the text representation of the original UTF-8 encoded data.
Converting to JSON
Once you have decoded the UTF-8 string, the next step is to convert it to a JSON format. Python's json module comes in handy for this task. Here's an example:
[[See Video to Reveal this Text or Code Snippet]]
In this snippet, the loads function from the json module is used to parse the decoded string into a JSON object. Now, json_data contains the data in a structured JSON format.
Handling Potential Issues
When working with character encodings, it's crucial to handle potential exceptions that may arise during decoding. You can use a try-except block to catch and address such issues:
[[See Video to Reveal this Text or Code Snippet]]
This approach helps you identify and handle decoding or JSON parsing errors gracefully.
Conclusion
Converting UTF-8 strings to JSON in Python involves decoding the strings using the appropriate method and then parsing them into a JSON format using the json module. Understanding character encodings and handling potential issues is crucial for robust data transformations.
In your Python projects, be mindful of the data encoding, and follow these steps to seamlessly convert UTF-8 encoded strings to JSON, ensuring that your data processing is accurate and reliable.