filmov
tv
Java Tutorial - 6 | Edureka
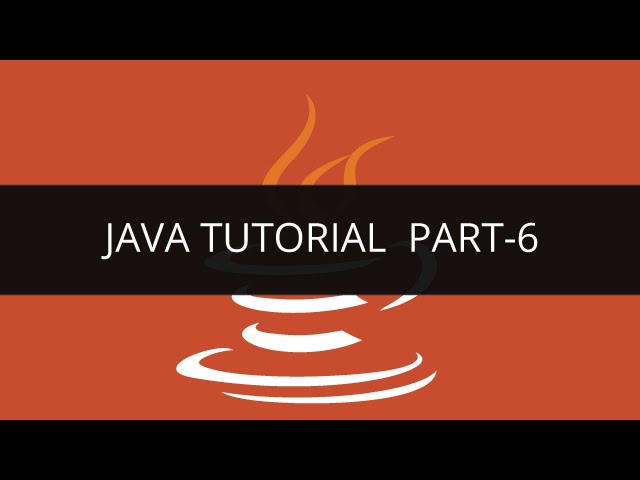
Показать описание
Take instructor-led Live class on Java Tutorial at :
The following topics were covered in this Java Tutorial:
Threads, Thread Lifecycle, Runnable, Thread Class, Synchronization, Deadlock, Examples
Thread:
A thread is a part of execution in a program. The JVM (Java Virtual Machine) allows an application to have multiple threads execution (running) concurrently. So that you can get the multi tasking feature available in your system. Hence multiple threads will be working in the back ground. Threads are lighter than processes i.e threads consume less memory as well as execution is faster.
E.g. of thread in our day to day life in computer: suppose you have a computer. Now in that computer you are playing game and got other tasks like notepad opened up, playing music, downloading files, browse internet and so on. You are using all these things simultaneously i.e. performing multitask all together. This is done with the help of threads. All these tasks are treated as threads and executing simultaneously.
Why do we use threads?
Thread is a light weight process as it shares the same memory and the resources of the process in which it is created. It shares the memory and resources
It promotes multi threading also.
Life --Cycle of a Thread:
When we start a program create a thread it enters into NEW state. The thread will enter into RUNNABLE state when we start the thread. You can make the thread WAIT using wait() available and later thread will be unlocked. You can make the thread SLEEP i.e thread will sleep for some time and again start executing. So you can stop executing by using sleep() available. Finally when the task is completed it gets TERMINATED and ends after that.
Thread -- Methods:
What is the difference between sleep() and wait()? -- both are used to block the threads. But the difference is that consider there is a thread using a resource and another thread is waiting for that resource. So the second thread has to wait until the resource gets free. When resource is free the first thread will notify the other threads which are waiting for the resource where as sleep() will wait for a particular time but do not get notify.
Thread Priorities:
When multiple threads are running, the order of execution of threads depend on priority given to thread. Priority values range from 1 to 10. If you do not set the priority to any thread then its priority will taken as 5 which is default priority. We use setPriority(priority_value) to set the priority to thread.
Threads can be created in two ways:
1. Implementing Runnable interface
2. Extending Thread class
Thread t= new Class1();
These statements will also work when you are creating a thread for a class that extends Thread class.
Synchronization:
E.g: consider I have two friends and a resource like pen. Both of them wants the same pen. So what I have to do is to synchronise the object(pen) i.e when first one is using, the second one cannot use it until the pen is released by first one and when the second one is using the pen then first one cannot use it until the pen is released by second one.
Inter-Thread Communication:
e.g.: suppose you went to buy a movie ticket and stand in the queue. The first person standing in the queue will get the ticket and ask the second person to wait. When the first person comes out he sends notify to second. Now the second will get the ticket and ask the third person to wait. This process will continue until either the tickets are finished or no persons left in the queue.The methods used for communication between threads are: wait(), notify(), notifyAll()
The following topics were covered in this Java Tutorial:
Threads, Thread Lifecycle, Runnable, Thread Class, Synchronization, Deadlock, Examples
Thread:
A thread is a part of execution in a program. The JVM (Java Virtual Machine) allows an application to have multiple threads execution (running) concurrently. So that you can get the multi tasking feature available in your system. Hence multiple threads will be working in the back ground. Threads are lighter than processes i.e threads consume less memory as well as execution is faster.
E.g. of thread in our day to day life in computer: suppose you have a computer. Now in that computer you are playing game and got other tasks like notepad opened up, playing music, downloading files, browse internet and so on. You are using all these things simultaneously i.e. performing multitask all together. This is done with the help of threads. All these tasks are treated as threads and executing simultaneously.
Why do we use threads?
Thread is a light weight process as it shares the same memory and the resources of the process in which it is created. It shares the memory and resources
It promotes multi threading also.
Life --Cycle of a Thread:
When we start a program create a thread it enters into NEW state. The thread will enter into RUNNABLE state when we start the thread. You can make the thread WAIT using wait() available and later thread will be unlocked. You can make the thread SLEEP i.e thread will sleep for some time and again start executing. So you can stop executing by using sleep() available. Finally when the task is completed it gets TERMINATED and ends after that.
Thread -- Methods:
What is the difference between sleep() and wait()? -- both are used to block the threads. But the difference is that consider there is a thread using a resource and another thread is waiting for that resource. So the second thread has to wait until the resource gets free. When resource is free the first thread will notify the other threads which are waiting for the resource where as sleep() will wait for a particular time but do not get notify.
Thread Priorities:
When multiple threads are running, the order of execution of threads depend on priority given to thread. Priority values range from 1 to 10. If you do not set the priority to any thread then its priority will taken as 5 which is default priority. We use setPriority(priority_value) to set the priority to thread.
Threads can be created in two ways:
1. Implementing Runnable interface
2. Extending Thread class
Thread t= new Class1();
These statements will also work when you are creating a thread for a class that extends Thread class.
Synchronization:
E.g: consider I have two friends and a resource like pen. Both of them wants the same pen. So what I have to do is to synchronise the object(pen) i.e when first one is using, the second one cannot use it until the pen is released by first one and when the second one is using the pen then first one cannot use it until the pen is released by second one.
Inter-Thread Communication:
e.g.: suppose you went to buy a movie ticket and stand in the queue. The first person standing in the queue will get the ticket and ask the second person to wait. When the first person comes out he sends notify to second. Now the second will get the ticket and ask the third person to wait. This process will continue until either the tickets are finished or no persons left in the queue.The methods used for communication between threads are: wait(), notify(), notifyAll()
Комментарии