filmov
tv
Java Programming All-in-One Tutorial Series (6 HOURS!)
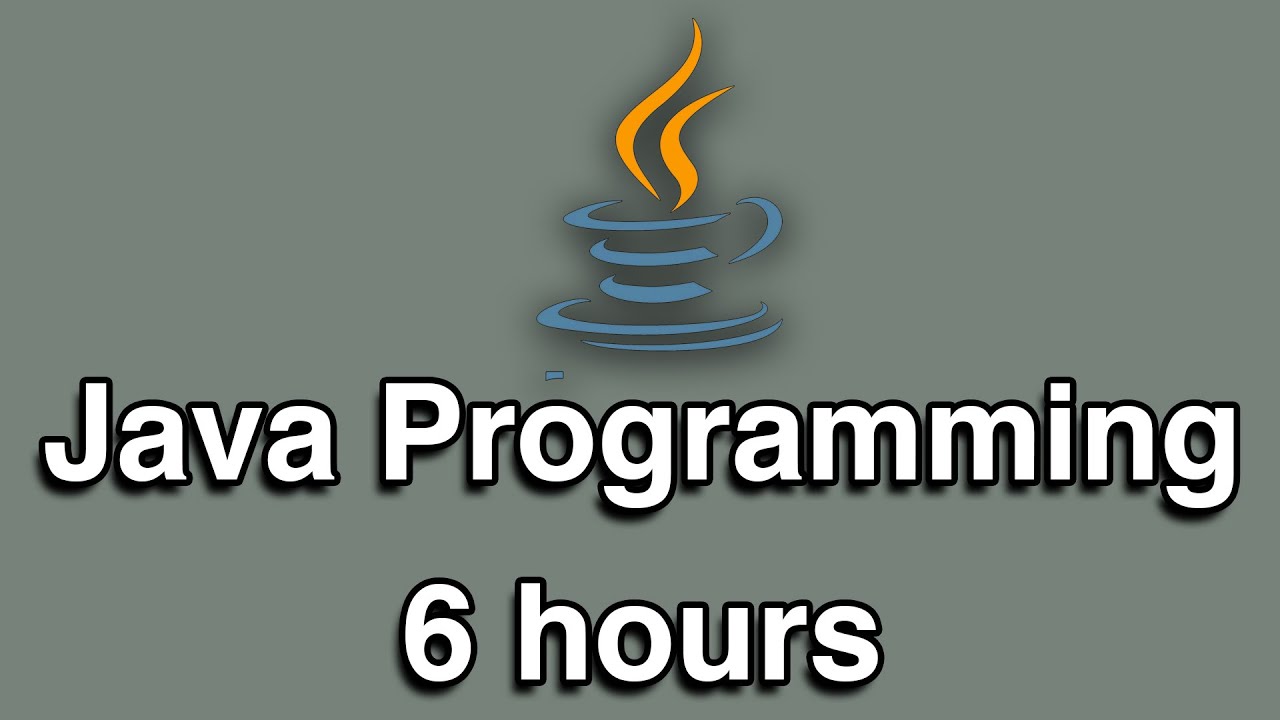
Показать описание
Timestamps:
00:00:00 - Introduction
00:07:17 - Installation and Hello World
00:11:49 - Understanding Java Foundations
00:22:20 - Arguments and Parameters
00:32:58 - Input and Output
00:41:09 - Variables
00:49:02 - Primitives and Objects
00:56:38 - Variable Declaration and Initialization
01:03:24 - Primitive Data Types
01:13:22 - Scanner Input
01:20:48 - Numeric Data Types and Properties (Infinity, NaN)
01:26:53 - Numeric Expressions and Operators
01:36:19 - Numeric Methods (max, compare, valueOf, parseInt, etc)
01:49:33 - String Methods (charAt, concat, contains, indexOf, lastIndexOf)
01:55:33 - More String Methods (toLowerCase, strip, substring, repeat, equals)
02:02:05 - Creating Basic Classes, Methods, and Properties
02:08:34 - String Comparison and Interning
02:15:14 - if, else if, else
02:22:36 - Comparison and Logical Operators
02:29:05 - Switch Statement
02:36:13 - Ternary Conditional Operator
02:41:13 - Single line if Statement
02:44:50 - Intro to Loops (While loops)
02:51:41 - Do While Loop
02:56:29 - for Loops
03:01:32 - Nested Blocks (Nested if)
03:04:44 - Nested for Loops (Triangles and Pyramids)
03:09:37 - Nested While Loops
03:13:44 - Variable Scope with Nested Control Flow
03:18:55 - break
03:22:34 - continue
03:24:45 - Intro to Arrays
03:29:58 - Working with Arrays
03:34:42 - Arrays toString and Arrays deeptoString
03:36:45 - Array Values from Input and for Loop
03:40:48 - Search an Array with for Loop
03:51:19 - 2d Arrays
03:54:49 - Working with 2D Arrays
03:57:52 - Iterate through 2D Structures with for Loop
04:01:39 - ArrayList Introduction
04:05:06 - List Interface and ArrayList Implementation
04:09:52 - Working with Lists (List Methods)
04:15:25 - Quickly Initialize a List with Elements and How to Print List
04:18:50 - for Loops with Lists & How to Modify Each Element
04:21:27 - for each Loop in Java
04:22:54 - Nested for each Loop
04:25:46 - Convert List to an Array
04:28:58 - Intro to Object Oriented Programming (OOP)
04:32:21 - Class vs Object
04:36:29 - Fields
04:39:18 - Public vs Private
04:43:31 - Methods
04:47:04 - Basics of Creating a Class and Object
04:49:19 - Adding Fields to a Class
04:51:11 - Creating Our First Method
04:52:31 - Arguments and Parameters in Methods
04:54:31 - Return Statement
04:57:42 - Encapsulation
5:00:33 - Create a Getter
05:03:16 - Create a Setter
05:06:20 - Custom Getter and Setter
05:10:18 - ArrayList f Custom Type
05:12:50 - Creating Custom Type in Loop
05:15:27 - Taking Custom Types as Arguments
05:18:04 - Intro to Static Methods
05:19:32 - Creating a Static Method
05:21:54 - Method to take an ArrayList of Custom Type
05:24:49 - Intro to Method Overloading and Optional Parameters
05:27:14 - Working with overloads to Print a User
05:29:54 - Searching a List for Custom Objects
05:35:15 - Method Overriding
05:37:21 - Override toString
05:39:13 - Override Equals
05:41:24 - Overload the Search to Take in a User Object
05:44:05 - Returning Custom Objects
05:47:43 - Passing by Value or Reference
05:51:16 - Intro to Inheritance
05:52:58 - Working with Inheritance
05:55:51 - Virtual in Java
05:58:10 - Creating a Method in User Class and Overriding in a Derived Class
06:00:35 - abstract Class
06:02:21 - abstract Method
06:03:41 - Polymorphism
06:06:09 - Polymorphism in Practice
06:09:29 - Intro to Constructors
06:12:49 - Creating the Default Constructor
06:14:57 - Custom Constructors
06:17:07 - Invoke Parent Class Methods with super keyword
06:19:04 - Readonly Fields Assigned with Constructor
06:21:40 - Intro to Interfaces
06:25:57 - Creating an Interface for Functionality
06:29:20 - Final Methods
06:30:25 - Final Classes
06:31:39 - Intro to enum
06:33:29 - enum in switch
06:34:34 - Conclusion
~~~~~~~~~~~~~~~ CONNECT ~~~~~~~~~~~~~~~
~~~~~~~~~~~~~~ SUPPORT ME ~~~~~~~~~~~~~~
🅑 Bitcoin - 3HnF1SWTzo1dCU7RwFLhgk7SYiVfV37Pbq
🅔 Eth - 0x350139af84b60d075a3a0379716040b63f6D3853
#java
Комментарии