filmov
tv
Beginner Python Programming All-in-One Tutorial Series (6 HOURS!)
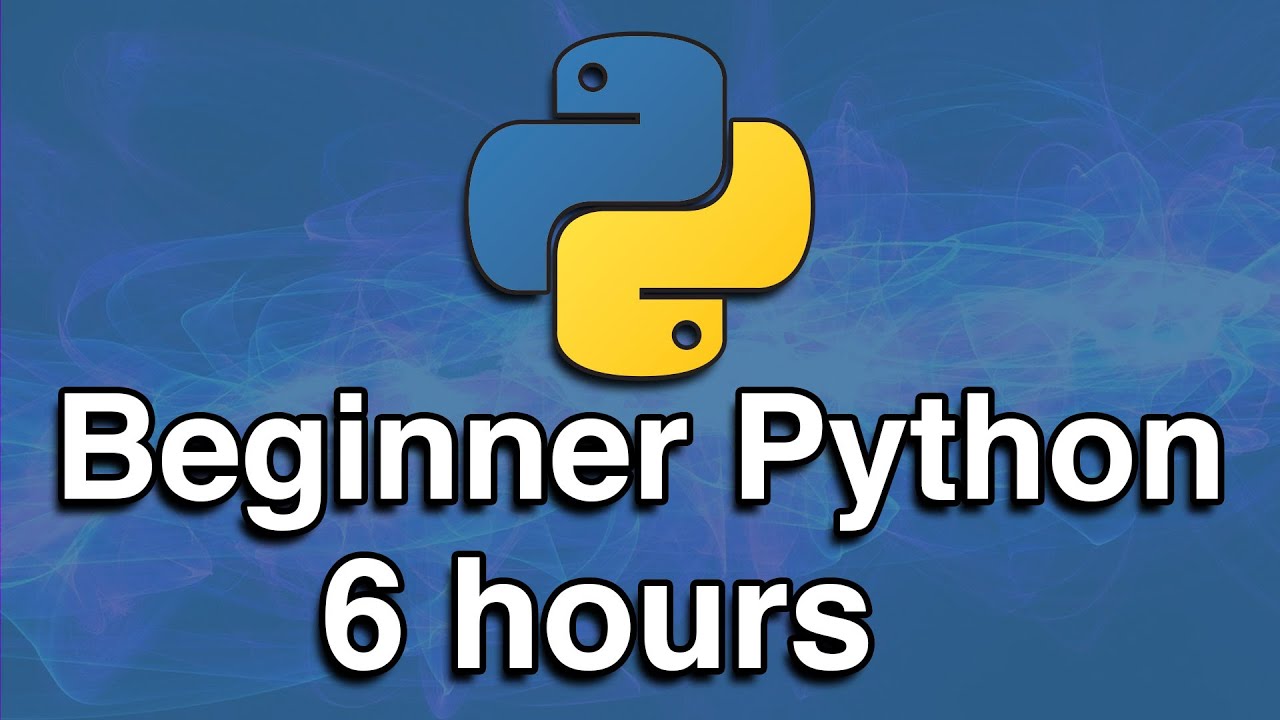
Показать описание
Timestamps
00:00:00 - Introduction
00:05:59 - Interactive Mode
00:10:24 - Working with Numbers
00:14:47 - Parenthesis and Precedence Rules
00:20:35 - Floor Division and Round
00:26:20 - Creating and Executing Python Files
00:31:22 - Installing and Setting up Visual Studio Code
00:35:33 - Print Function
00:40:46 - Interactive Mode in Visual Studio Code
00:46:21 - Creating a Number Variable
00:53:25 - Comments
00:57:15 - Variable Naming Rules
01:02:33 - Floor Division (Double Forward Slash)
01:07:15 - Modulus
01:10:48 - Power
01:14:06 - Review 01
01:19:53 - Creating a String
01:21:49 - Escape Characters
01:26:07 - Double vs Single Quotes
01:30:44 - Concatenation
01:34:22 - Concatenation with Literals
01:37:59 - Multiline Strings
01:42:03 - Indexes
01:45:05 - Slicing Strings
01:47:50 - Negatives with String Slicing
01:52:49 - Slicing with Two Numbers
01:58:52 - Strings are Immutable
02:04:49 - len() Function
02:07:54 - Convert Integer to String
02:10:31 - Nested Function Calls
02:14:06 - Review of Strings and Slicing
02:24:40 - Creating a List and Indexing
02:26:58 - Changing and Slicing a List
02:29:36 - How to Copy a List
02:33:07 - Intro to Nested Lists (2D Lists)
02:40:39 - Combining Lists
02:42:38 - Review of List Basics
02:48:59 - Getting User Input
02:53:12 - Type Casting
03:00:58 - Review of Lists and User Input
03:05:29 - Boolean and Comparison Operators
03:09:17 - if Statement
03:12:27 - Tabs vs Spaces
03:15:38 - if, elif, else
03:20:00 - if Statement with Boolean variables
03:22:51 - or Operator (Intro to Logical Operators)
03:25:47 - and Operator
03:28:02 - not Operator
03:30:15 - Control Flow and Logic Review
03:36:03 - For Loop
03:37:35 - Print without Newline using end Parameter
03:39:06 - range() Function
03:41:00 - Range Starting Position
03:42:54 - Step in Range Explained
03:44:45 - Range Sum
03:46:14 - Create a List from Range
03:48:18 - For Loop with Index
03:51:31 - Loop Basics Review
03:56:49 - break
04:00:32 - continue
04:03:26 - else Instead of Continue
04:05:36 - Pass
04:08:25 - else with for
04:11:19 - while Loop
04:14:15 - Converting Between while and for Loops
04:16:40 - Else with while
04:21:31 - Flag Variable Example
04:24:12 - do while Loop in Python
04:27:39 - Indefinite Loop
04:32:17 - lower and upper Functions
04:37:03 - isupper and islower Functions
04:39:43 - Review of Loop Variations
04:50:25 - Nested if
04:54:25 - Complex Conditional vs Nested if
05:00:52 - Nested for Loops
05:04:01 - Review of Nested if Statements
05:10:19 - Nested for Loop Variations
05:13:23 - Loop Variable Within range (Triangles)
05:15:48 - Nested while Loops
05:18:54 - Nested While Loops to Calculate Sums
05:23:40 - Review of Nested Loops
05:27:46 - Creating a Function
05:31:51 - Arguments and Parameters
05:34:20 - return
05:35:57 - return vs else to Exit Function
05:37:23 - How to Return a Value from Function
05:41:28 - Default Parameters
05:43:21 - Multiple Arguments / Parameters
05:45:11 - Keyword Arguments
05:47:00 - Passing Arguments by Keyword or Position
05:49:23 - Positional Only Parameter
05:51:18 - Keyword Only Arguments
05:52:20 - Position and Keyword Summary
05:55:12 - Function to Work with Lists
05:57:53 - Function Taking Unlimited Arguments
05:59:37 - Unpacking Data
06:02:29 - Functions Calling Functions
06:06:38 - Review of Functions
06:14:08 - Conclusion and Up Next
~~~~~~~~~~~~~~~ CONNECT ~~~~~~~~~~~~~~~
~~~~~~~~~~~~~~ SUPPORT ME ~~~~~~~~~~~~~~
🅑 Bitcoin - 3HnF1SWTzo1dCU7RwFLhgk7SYiVfV37Pbq
🅔 Eth - 0x350139af84b60d075a3a0379716040b63f6D3853
00:00:00 - Introduction
00:05:59 - Interactive Mode
00:10:24 - Working with Numbers
00:14:47 - Parenthesis and Precedence Rules
00:20:35 - Floor Division and Round
00:26:20 - Creating and Executing Python Files
00:31:22 - Installing and Setting up Visual Studio Code
00:35:33 - Print Function
00:40:46 - Interactive Mode in Visual Studio Code
00:46:21 - Creating a Number Variable
00:53:25 - Comments
00:57:15 - Variable Naming Rules
01:02:33 - Floor Division (Double Forward Slash)
01:07:15 - Modulus
01:10:48 - Power
01:14:06 - Review 01
01:19:53 - Creating a String
01:21:49 - Escape Characters
01:26:07 - Double vs Single Quotes
01:30:44 - Concatenation
01:34:22 - Concatenation with Literals
01:37:59 - Multiline Strings
01:42:03 - Indexes
01:45:05 - Slicing Strings
01:47:50 - Negatives with String Slicing
01:52:49 - Slicing with Two Numbers
01:58:52 - Strings are Immutable
02:04:49 - len() Function
02:07:54 - Convert Integer to String
02:10:31 - Nested Function Calls
02:14:06 - Review of Strings and Slicing
02:24:40 - Creating a List and Indexing
02:26:58 - Changing and Slicing a List
02:29:36 - How to Copy a List
02:33:07 - Intro to Nested Lists (2D Lists)
02:40:39 - Combining Lists
02:42:38 - Review of List Basics
02:48:59 - Getting User Input
02:53:12 - Type Casting
03:00:58 - Review of Lists and User Input
03:05:29 - Boolean and Comparison Operators
03:09:17 - if Statement
03:12:27 - Tabs vs Spaces
03:15:38 - if, elif, else
03:20:00 - if Statement with Boolean variables
03:22:51 - or Operator (Intro to Logical Operators)
03:25:47 - and Operator
03:28:02 - not Operator
03:30:15 - Control Flow and Logic Review
03:36:03 - For Loop
03:37:35 - Print without Newline using end Parameter
03:39:06 - range() Function
03:41:00 - Range Starting Position
03:42:54 - Step in Range Explained
03:44:45 - Range Sum
03:46:14 - Create a List from Range
03:48:18 - For Loop with Index
03:51:31 - Loop Basics Review
03:56:49 - break
04:00:32 - continue
04:03:26 - else Instead of Continue
04:05:36 - Pass
04:08:25 - else with for
04:11:19 - while Loop
04:14:15 - Converting Between while and for Loops
04:16:40 - Else with while
04:21:31 - Flag Variable Example
04:24:12 - do while Loop in Python
04:27:39 - Indefinite Loop
04:32:17 - lower and upper Functions
04:37:03 - isupper and islower Functions
04:39:43 - Review of Loop Variations
04:50:25 - Nested if
04:54:25 - Complex Conditional vs Nested if
05:00:52 - Nested for Loops
05:04:01 - Review of Nested if Statements
05:10:19 - Nested for Loop Variations
05:13:23 - Loop Variable Within range (Triangles)
05:15:48 - Nested while Loops
05:18:54 - Nested While Loops to Calculate Sums
05:23:40 - Review of Nested Loops
05:27:46 - Creating a Function
05:31:51 - Arguments and Parameters
05:34:20 - return
05:35:57 - return vs else to Exit Function
05:37:23 - How to Return a Value from Function
05:41:28 - Default Parameters
05:43:21 - Multiple Arguments / Parameters
05:45:11 - Keyword Arguments
05:47:00 - Passing Arguments by Keyword or Position
05:49:23 - Positional Only Parameter
05:51:18 - Keyword Only Arguments
05:52:20 - Position and Keyword Summary
05:55:12 - Function to Work with Lists
05:57:53 - Function Taking Unlimited Arguments
05:59:37 - Unpacking Data
06:02:29 - Functions Calling Functions
06:06:38 - Review of Functions
06:14:08 - Conclusion and Up Next
~~~~~~~~~~~~~~~ CONNECT ~~~~~~~~~~~~~~~
~~~~~~~~~~~~~~ SUPPORT ME ~~~~~~~~~~~~~~
🅑 Bitcoin - 3HnF1SWTzo1dCU7RwFLhgk7SYiVfV37Pbq
🅔 Eth - 0x350139af84b60d075a3a0379716040b63f6D3853
Комментарии