filmov
tv
ALL Python Programmers Should Know This!! #python #programming #coding
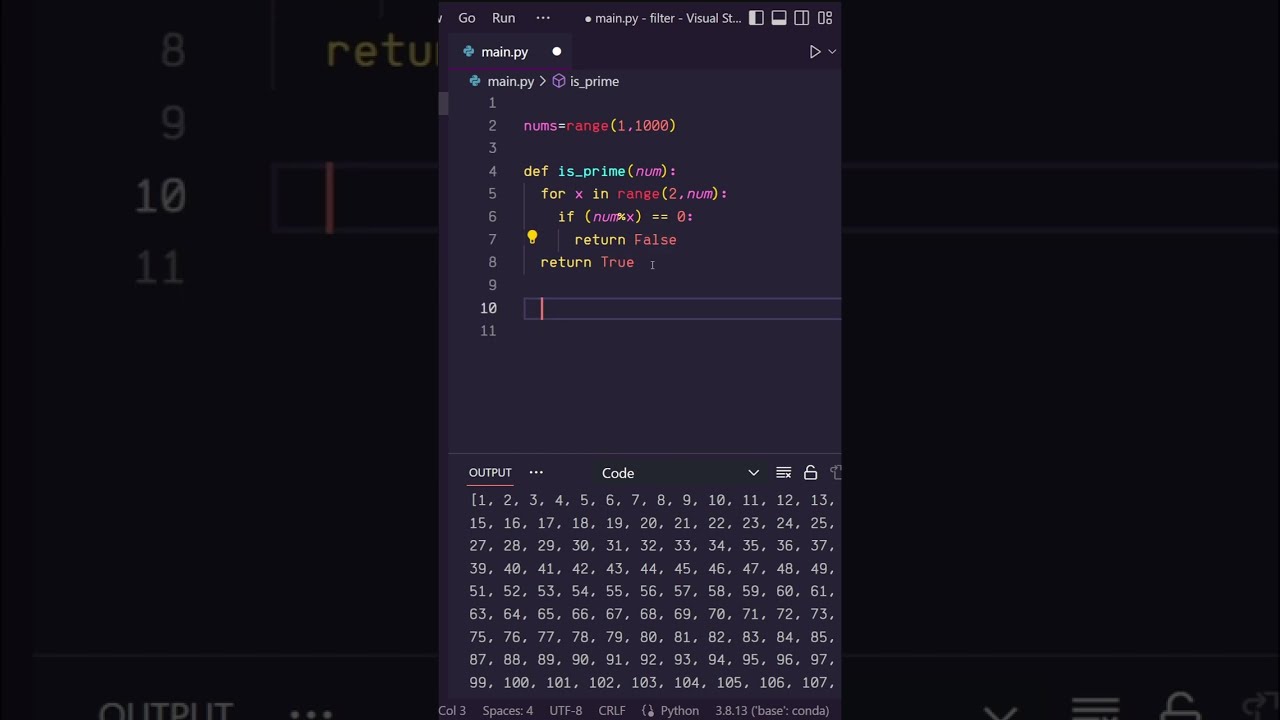
Показать описание
This quick demonstration shows you how powerful Python's filter() function can be!
Background Music:
Creative Commons Attribution-ShareAlike 3.0 Unported
ALL Python Programmers Should Know This!! #python #programming #coding
Mastering Python - Everything You Need To Know To Become a Python Master
25 Terms All Programmers NEED To Know!
All Python programmers should know this..!! #coding #programming #python
Subscribe for more Coding Tips! 🔥ALL Python Programmers should know this! #coder #programming #ai
Python for Beginners - Learn Python in 1 Hour
ALL Python Programmers Should Know This!! #python #programming #coding #shorts
Python 101: Learn the 5 Must-Know Concepts
New py Functions That Save Time! #python #programming #tipsandtricks #beginners #learnpython #coding
ALL Python Programmers should know this! #programmer
Subscribe for more Coding Tips!🔥ALL Python Programmers should know this!#python #pythonprogramming...
ALL Python Programmers should know this! #python
ALL Python Programmers Should Know This!! #python #coding #programming
ALL Python Programmers should know this! #pythoncoding
ALL Python Programmers should know this! #codinglife #frontenddevelopment
Normal People VS Programmers #coding #python #programming #easy #funny #short
If you're struggling to learn to code, you must watch this
ALL Python Programmers should know this! #pythonprogramming #pyhton
ALL Python Programmers should know this! #shortsvideo #codingtips
All Python Programmers Should Know This!! #python #programming #coding
How to Get Ahead of 99% of Programmers (in 99 seconds)
ALL Python Programmers should know this! #technology #tips #programming
ALL Python Programmers should know this! #codinglife #students
ALL Python Programmers Should Know This Function!! #python #programming #coding
Комментарии