filmov
tv
Solving the List index out of range Error When Reading XYZ Files in Python
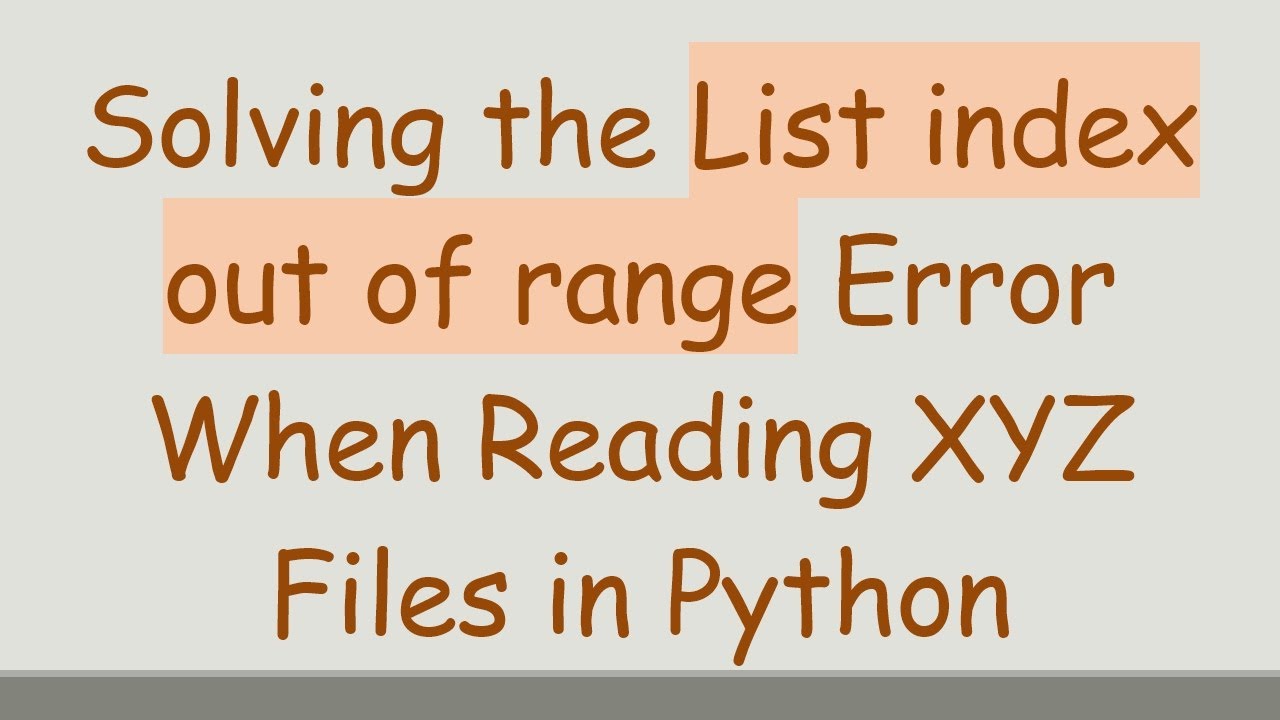
Показать описание
Discover how to efficiently read XYZ files in Python without encountering the `list index out of range` error. Learn practical coding techniques and best practices to streamline your data processing.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: List index out range while ready xyz file
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Tackling the List Index Out of Range Error When Reading XYZ Files in Python
When working with data files in Python, especially those formatted in XYZ for scientific or analytical purposes, you may run into a common error: the dreaded list index out of range. This issue can derail your data processing and leave you scratching your head. In this post, we'll explore the problem of reading XYZ files and provide a step-by-step solution.
Understanding the Problem
The error typically arises when the code attempts to access an index in a list that does not exist. In the context of reading a file, this often happens when the file pointer has moved to the end of the file (EOF) and there are no lines left to read.
Example Scenario
Consider the following XYZ file structure (this is a simplified example based on your needs):
[[See Video to Reveal this Text or Code Snippet]]
When you try to read these lines into a Particle3D object, you might encounter the list index out of range error if you're not careful about how you handle the file reading.
The Solution
Let's break down the solution into clear steps that can help you avoid this error while reading your XYZ file.
Step 1: Read File Line by Line
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Modify the new_particle Function
To accommodate this change, you need to modify the new_particle function to accept a line instead of a file handle. Here’s the updated function definition:
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Testing Your Code
After making these adjustments, it’s time to test the code. Make sure that your XYZ file follows the expected format for successful parsing. If every particle is on a new line and the values align correctly, your data should load without issue.
Conclusion
By restructuring how files are read and adapting the new_particle method, you can efficiently read XYZ files in Python without encountering the list index out of range error. This approach not only prevents errors but also helps to maintain cleaner, more efficient code.
Now you’re one step closer to seamless data processing in your Python projects. If you have more questions, feel free to ask!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: List index out range while ready xyz file
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Tackling the List Index Out of Range Error When Reading XYZ Files in Python
When working with data files in Python, especially those formatted in XYZ for scientific or analytical purposes, you may run into a common error: the dreaded list index out of range. This issue can derail your data processing and leave you scratching your head. In this post, we'll explore the problem of reading XYZ files and provide a step-by-step solution.
Understanding the Problem
The error typically arises when the code attempts to access an index in a list that does not exist. In the context of reading a file, this often happens when the file pointer has moved to the end of the file (EOF) and there are no lines left to read.
Example Scenario
Consider the following XYZ file structure (this is a simplified example based on your needs):
[[See Video to Reveal this Text or Code Snippet]]
When you try to read these lines into a Particle3D object, you might encounter the list index out of range error if you're not careful about how you handle the file reading.
The Solution
Let's break down the solution into clear steps that can help you avoid this error while reading your XYZ file.
Step 1: Read File Line by Line
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Modify the new_particle Function
To accommodate this change, you need to modify the new_particle function to accept a line instead of a file handle. Here’s the updated function definition:
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Testing Your Code
After making these adjustments, it’s time to test the code. Make sure that your XYZ file follows the expected format for successful parsing. If every particle is on a new line and the values align correctly, your data should load without issue.
Conclusion
By restructuring how files are read and adapting the new_particle method, you can efficiently read XYZ files in Python without encountering the list index out of range error. This approach not only prevents errors but also helps to maintain cleaner, more efficient code.
Now you’re one step closer to seamless data processing in your Python projects. If you have more questions, feel free to ask!