filmov
tv
Solving the list index out of range Error in Python: A Detailed Guide
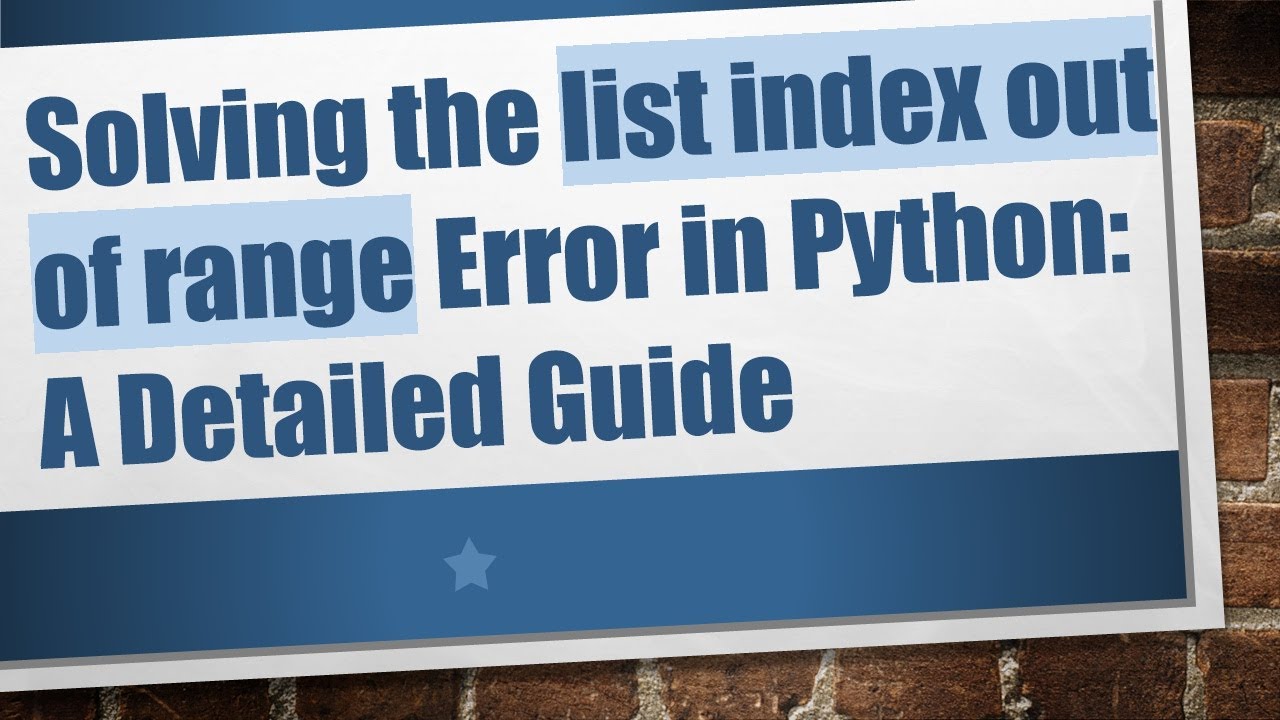
Показать описание
Learn how to resolve the common "list index out of range" error in Python, particularly when dealing with list manipulations like deleting occurrences of elements.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: I have an error " list index out of range" that I can't solve
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding and Resolving the list index out of range Error
When working with lists in Python, encountering errors can be frustrating, especially when they seem to arise out of nowhere. One common error that many coders face is the "list index out of range" error. This occurs when the code attempts to access an index in a list that does not exist. Let’s break down this problem and its solution using a practical example, particularly one that involves controlling occurrences of elements in a list.
The Problem
You’re tasked with solving a challenge on CodeWars that requires you to delete occurrences of an element if it appears more than N times in a given list. Here’s the requirement again:
Function: delete_nth(lst, N)
Behavior: Create a new list that contains each number from lst at most N times without changing the order of elements.
For instance:
delete_nth([1,1,1,1], 2) should return [1, 1]
delete_nth([20,37,20,21], 1) should return [20, 37, 21]
However, when running the function with an empty list as input, you encounter a list index out of range error. This can happen with an input like delete_nth([], 5).
Why the Error Occurs
Let's analyze the provided code snippet that leads to this error:
[[See Video to Reveal this Text or Code Snippet]]
The problem lies within your while loop, specifically this line:
[[See Video to Reveal this Text or Code Snippet]]
If the input list order is empty, o will also be empty. Trying to access o[x] will result in an IndexError since there are no elements in o to access.
The Solution: Handling Edge Cases
To prevent this error, you can add a simple check at the beginning of your function to handle the edge case of an empty list. Here's how you can adjust your function:
[[See Video to Reveal this Text or Code Snippet]]
Key Changes Made:
Edge Case Check: Before proceeding with any operations, the code now checks if order is empty and returns an empty list immediately if true.
Conclusion
By implementing this simple check, we can effectively avoid the list index out of range error when an empty list is passed to the function. This highlights the importance of validating inputs in programming, especially when working with lists and other data structures.
If you apply these adjustments, you should no longer encounter issues with your function when handling edge cases. Keep coding, and don’t hesitate to reach out for help whenever you hit a snag! Remember, debugging is part of the learning process.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: I have an error " list index out of range" that I can't solve
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding and Resolving the list index out of range Error
When working with lists in Python, encountering errors can be frustrating, especially when they seem to arise out of nowhere. One common error that many coders face is the "list index out of range" error. This occurs when the code attempts to access an index in a list that does not exist. Let’s break down this problem and its solution using a practical example, particularly one that involves controlling occurrences of elements in a list.
The Problem
You’re tasked with solving a challenge on CodeWars that requires you to delete occurrences of an element if it appears more than N times in a given list. Here’s the requirement again:
Function: delete_nth(lst, N)
Behavior: Create a new list that contains each number from lst at most N times without changing the order of elements.
For instance:
delete_nth([1,1,1,1], 2) should return [1, 1]
delete_nth([20,37,20,21], 1) should return [20, 37, 21]
However, when running the function with an empty list as input, you encounter a list index out of range error. This can happen with an input like delete_nth([], 5).
Why the Error Occurs
Let's analyze the provided code snippet that leads to this error:
[[See Video to Reveal this Text or Code Snippet]]
The problem lies within your while loop, specifically this line:
[[See Video to Reveal this Text or Code Snippet]]
If the input list order is empty, o will also be empty. Trying to access o[x] will result in an IndexError since there are no elements in o to access.
The Solution: Handling Edge Cases
To prevent this error, you can add a simple check at the beginning of your function to handle the edge case of an empty list. Here's how you can adjust your function:
[[See Video to Reveal this Text or Code Snippet]]
Key Changes Made:
Edge Case Check: Before proceeding with any operations, the code now checks if order is empty and returns an empty list immediately if true.
Conclusion
By implementing this simple check, we can effectively avoid the list index out of range error when an empty list is passed to the function. This highlights the importance of validating inputs in programming, especially when working with lists and other data structures.
If you apply these adjustments, you should no longer encounter issues with your function when handling edge cases. Keep coding, and don’t hesitate to reach out for help whenever you hit a snag! Remember, debugging is part of the learning process.