filmov
tv
Understanding and Resolving the List Index Out of Range Error with Nested Lists in Python
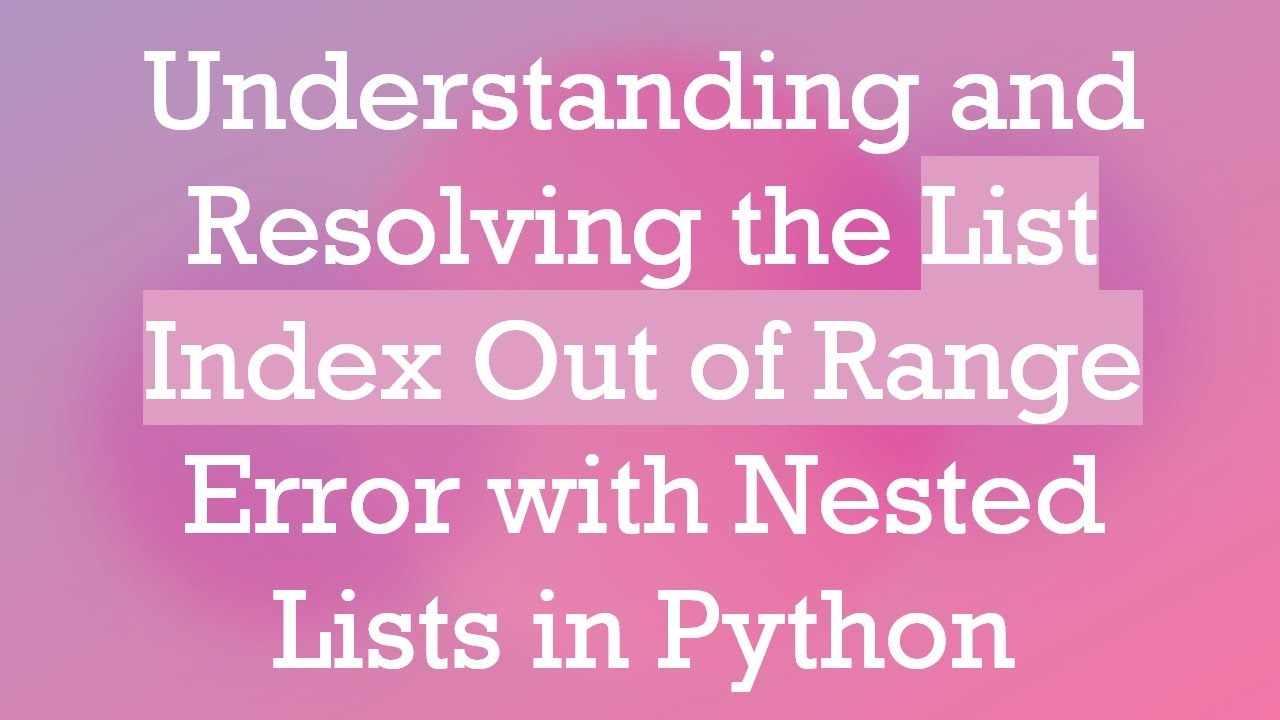
Показать описание
Summary: Uncover why your Python code is throwing a "list index out of range" error when working with nested lists and learn effective ways to resolve this issue.
---
Understanding and Resolving the List Index Out of Range Error with Nested Lists in Python
One of the common errors that Python developers encounter is the list index out of range error. It typically occurs when trying to access an index in a list that doesn’t exist. This error can become especially tricky when dealing with nested lists. In this guide, we will demystify why this error occurs and provide some practical ways to avoid it.
Why Does the Error Occur?
When you see a list index out of range error, it essentially means that you are trying to access a list element using an index that is either negative or greater than the current last index value. Nested lists add another layer of complexity since each element in the main list is itself a list, which can lead to multiple points of failure.
For example:
[[See Video to Reveal this Text or Code Snippet]]
In this code, nested_list[3][0] will produce an IndexError because nested_list only has three sublists (with indices 0, 1, and 2), and there is no index 3.
Identifying the Problem
To avoid or debug this issue, it is essential to:
Verify the List Length: Before accessing an index, ensure that it exists within the list.
Print Statements: Use debugging statements to print out lengths and indices you are working with.
Use Try-Except Blocks: Handle exceptions gracefully where feasible.
Practical Solutions
Verify the Index
Always verify the index before accessing it. You can use an if condition to check if the index is within the bounds of the list.
[[See Video to Reveal this Text or Code Snippet]]
Nested Loops
When dealing with nested lists, nested loops can help safely iterate through the elements.
[[See Video to Reveal this Text or Code Snippet]]
Use Try-Except Blocks
Using try-except blocks can help handle errors gracefully. This is useful for logging errors or providing feedback without crashing the program.
[[See Video to Reveal this Text or Code Snippet]]
Avoiding Common Pitfalls
Misalignment between the nested index and the sublist's length can often go unnoticed. Verify both the outer list index and the inner list length.
Assuming the presence of an index without checking its bounds can lead to frequent errors in dynamic or user-input-driven lists.
Conclusion
Understanding why the list index out of range error occurs, especially with nested lists, is crucial for writing robust Python code. By verifying indices, appropriately using loops, and handling exceptions, you can avoid these common pitfalls and ensure smoother program operation.
Happy coding!
---
Understanding and Resolving the List Index Out of Range Error with Nested Lists in Python
One of the common errors that Python developers encounter is the list index out of range error. It typically occurs when trying to access an index in a list that doesn’t exist. This error can become especially tricky when dealing with nested lists. In this guide, we will demystify why this error occurs and provide some practical ways to avoid it.
Why Does the Error Occur?
When you see a list index out of range error, it essentially means that you are trying to access a list element using an index that is either negative or greater than the current last index value. Nested lists add another layer of complexity since each element in the main list is itself a list, which can lead to multiple points of failure.
For example:
[[See Video to Reveal this Text or Code Snippet]]
In this code, nested_list[3][0] will produce an IndexError because nested_list only has three sublists (with indices 0, 1, and 2), and there is no index 3.
Identifying the Problem
To avoid or debug this issue, it is essential to:
Verify the List Length: Before accessing an index, ensure that it exists within the list.
Print Statements: Use debugging statements to print out lengths and indices you are working with.
Use Try-Except Blocks: Handle exceptions gracefully where feasible.
Practical Solutions
Verify the Index
Always verify the index before accessing it. You can use an if condition to check if the index is within the bounds of the list.
[[See Video to Reveal this Text or Code Snippet]]
Nested Loops
When dealing with nested lists, nested loops can help safely iterate through the elements.
[[See Video to Reveal this Text or Code Snippet]]
Use Try-Except Blocks
Using try-except blocks can help handle errors gracefully. This is useful for logging errors or providing feedback without crashing the program.
[[See Video to Reveal this Text or Code Snippet]]
Avoiding Common Pitfalls
Misalignment between the nested index and the sublist's length can often go unnoticed. Verify both the outer list index and the inner list length.
Assuming the presence of an index without checking its bounds can lead to frequent errors in dynamic or user-input-driven lists.
Conclusion
Understanding why the list index out of range error occurs, especially with nested lists, is crucial for writing robust Python code. By verifying indices, appropriately using loops, and handling exceptions, you can avoid these common pitfalls and ensure smoother program operation.
Happy coding!