filmov
tv
Solving the IndexError: list index out of range in Your Python Code
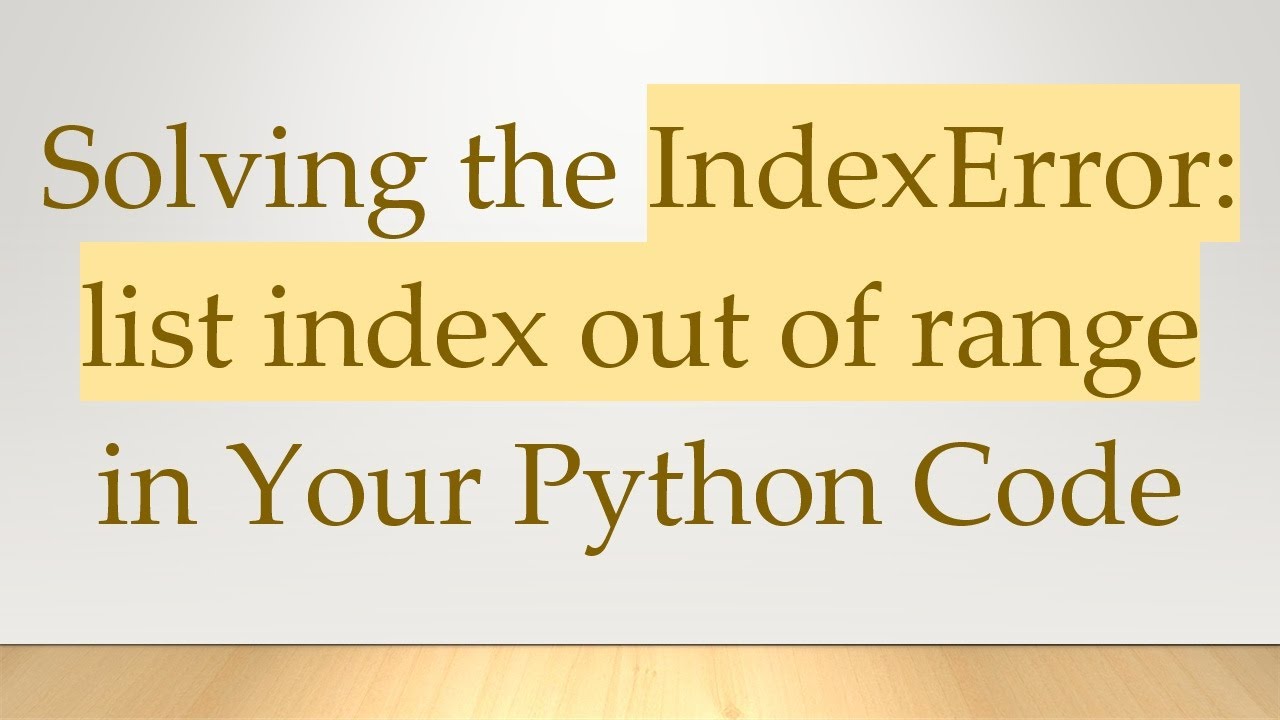
Показать описание
Discover the reasons behind the `IndexError` in your Python script and learn effective solutions for reading lines from a file correctly.
---
Visit these links for original content and any more details, such as alternate solutions, comments, revision history etc. For example, the original title of the Question was: Why am I getting an IndexError on this program?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the IndexError: list index out of range
If you’re diving into Python programming, you've likely encountered various errors while running your code. One common error that can leave you scratching your head is the dreaded IndexError: list index out of range. This error can be especially frustrating when you’re simply trying to perform straightforward calculations, such as subtracting numbers from a text file.
In this post, we will explore a specific problem that causes this error, and I'll guide you through the solution step by step. So let’s get started!
The Problem: Code Overview
You have a snippet of code that aims to read two numbers from a text file and subtract the second from the first. Here’s a simplified version of the code you might be running:
[[See Video to Reveal this Text or Code Snippet]]
Your Input Data
[[See Video to Reveal this Text or Code Snippet]]
You expect the output to be 250. However, when you run your code, you encounter this error:
[[See Video to Reveal this Text or Code Snippet]]
The Cause of the Error
The key issue here lies in how you are using the readlines() method. When you call readlines() the first time, it reads all the lines from the file into a list and moves the file pointer to the end of the file. Consequently, when you call readlines() a second time, there's nothing left to read, resulting in an empty list.
Analyzing the Flow
First call to readlines(): Reads all lines and returns them.
Second call to readlines(): Returns an empty list because the end of the file has been reached.
Thus, attempting to access the second line of the list (index 1) leads to the IndexError.
The Solutions
Fortunately, there are straightforward fixes for this error. Here are two effective methods you can adopt:
Method 1: Read All Lines Once
Instead of calling readlines() twice, you can store the result in a variable and access the lines from that variable. Here’s how:
[[See Video to Reveal this Text or Code Snippet]]
Method 2: Read One Line at a Time
Another approach is to read the file line by line using the readline() method. This way, each call will read the next line in the file:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
The IndexError: list index out of range is a common hurdle for many Python beginners, especially when reading from files. By understanding the cause of the error and applying one of the solutions provided, you can effectively resolve the issue and run your code successfully.
Remember, handling file I/O correctly is crucial in Python, and these methods will make your code cleaner and less prone to errors. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, comments, revision history etc. For example, the original title of the Question was: Why am I getting an IndexError on this program?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the IndexError: list index out of range
If you’re diving into Python programming, you've likely encountered various errors while running your code. One common error that can leave you scratching your head is the dreaded IndexError: list index out of range. This error can be especially frustrating when you’re simply trying to perform straightforward calculations, such as subtracting numbers from a text file.
In this post, we will explore a specific problem that causes this error, and I'll guide you through the solution step by step. So let’s get started!
The Problem: Code Overview
You have a snippet of code that aims to read two numbers from a text file and subtract the second from the first. Here’s a simplified version of the code you might be running:
[[See Video to Reveal this Text or Code Snippet]]
Your Input Data
[[See Video to Reveal this Text or Code Snippet]]
You expect the output to be 250. However, when you run your code, you encounter this error:
[[See Video to Reveal this Text or Code Snippet]]
The Cause of the Error
The key issue here lies in how you are using the readlines() method. When you call readlines() the first time, it reads all the lines from the file into a list and moves the file pointer to the end of the file. Consequently, when you call readlines() a second time, there's nothing left to read, resulting in an empty list.
Analyzing the Flow
First call to readlines(): Reads all lines and returns them.
Second call to readlines(): Returns an empty list because the end of the file has been reached.
Thus, attempting to access the second line of the list (index 1) leads to the IndexError.
The Solutions
Fortunately, there are straightforward fixes for this error. Here are two effective methods you can adopt:
Method 1: Read All Lines Once
Instead of calling readlines() twice, you can store the result in a variable and access the lines from that variable. Here’s how:
[[See Video to Reveal this Text or Code Snippet]]
Method 2: Read One Line at a Time
Another approach is to read the file line by line using the readline() method. This way, each call will read the next line in the file:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
The IndexError: list index out of range is a common hurdle for many Python beginners, especially when reading from files. By understanding the cause of the error and applying one of the solutions provided, you can effectively resolve the issue and run your code successfully.
Remember, handling file I/O correctly is crucial in Python, and these methods will make your code cleaner and less prone to errors. Happy coding!