filmov
tv
Finding the Sum of Surrounding Digits Around 1's in a Matrix Using Python
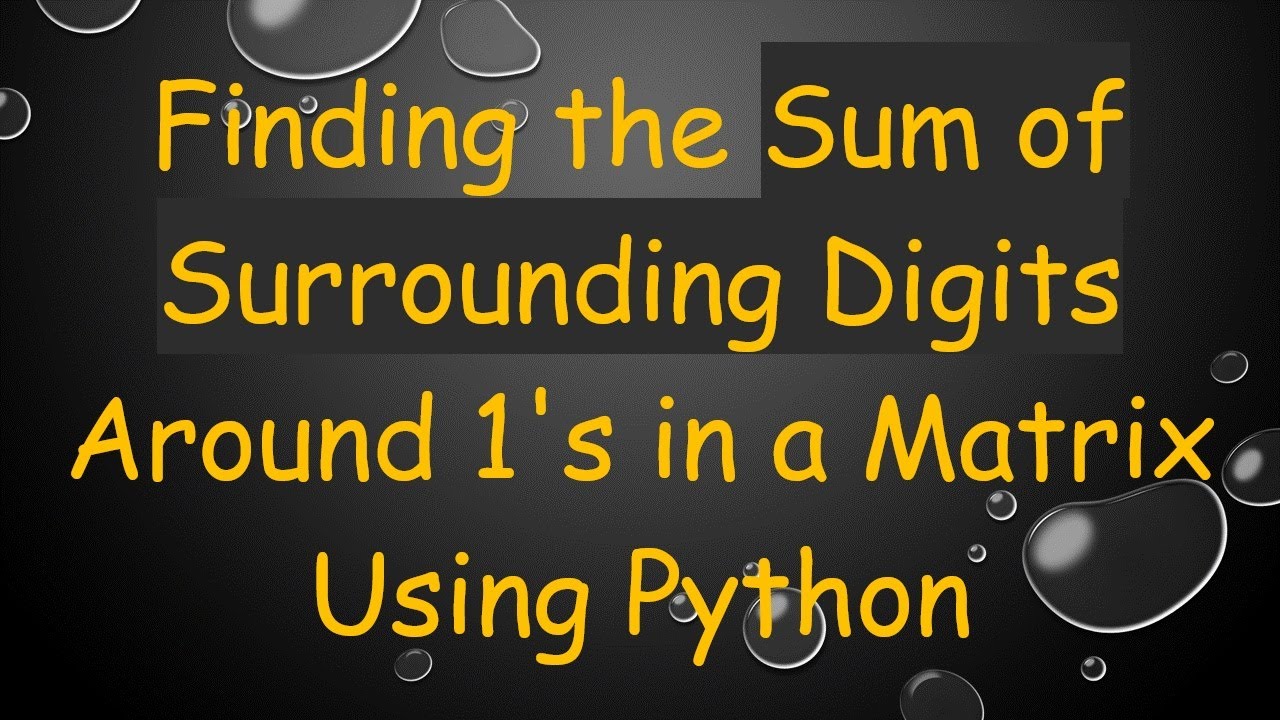
Показать описание
Learn how to sum the surrounding elements of consecutive `1's` in a matrix with this comprehensive Python guide using NumPy and SciPy.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Python - Method to get the array around an slice in a matrix
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Finding the Sum of Surrounding Digits Around 1's in a Matrix Using Python
In the realm of data processing and analysis, matrix manipulations are a fundamental task, especially when it comes to numeric data. One common challenge that many programmers face is extracting meaningful information from matrices. In this guide, we'll explore a fascinating problem: how to compute the sum of digits that surround consecutive 1's in a binary matrix.
The Problem at Hand
Imagine you have a binary matrix filled with 0's and 1's, similar to the example below:
[[See Video to Reveal this Text or Code Snippet]]
In this matrix, your goal is to find consecutive 1's and calculate the sum of any 1's that are positioned directly around these groups. For example, surrounding the 111 found in the fifth row results in a sum of 1.
The Solution Breakdown
To solve this problem, we can employ the power of two popular Python libraries: NumPy and SciPy. Together, they will allow us to efficiently manipulate the matrix and extract the desired data.
Step 1: Set Up Your Environment
Start by importing the necessary libraries:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Create a Random Matrix
We can create a random binary matrix as follows:
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Label the Regions in the Matrix
Using ndimage from SciPy, we can label connected regions in our matrix:
[[See Video to Reveal this Text or Code Snippet]]
This step assigns a unique label to each group of consecutive 1's, making it easier to analyze them individually.
Step 4: Calculate the Surrounding Sums
Now that we have a labeled matrix, we want to identify the number of 1's surrounding each labeled region. We'll do this using binary dilation and logical operations:
[[See Video to Reveal this Text or Code Snippet]]
Summary of the Process
Import Libraries: We utilize NumPy for numerical operations and SciPy for image processing functions.
Create a Matrix: Generate a random binary matrix.
Label Regions: Use a structuring element to label connected components.
Sum Surrounding 1's: For each labeled group, calculate how many 1's surround it and print the result.
Conclusion
This approach demonstrates how powerful NumPy and SciPy can be in handling matrix-related operations. By breaking down the problem into smaller parts, we were able to derive the surrounding sum of 1's in an efficient manner.
We hope you found this guide helpful for your matrix manipulation needs in Python! Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Python - Method to get the array around an slice in a matrix
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Finding the Sum of Surrounding Digits Around 1's in a Matrix Using Python
In the realm of data processing and analysis, matrix manipulations are a fundamental task, especially when it comes to numeric data. One common challenge that many programmers face is extracting meaningful information from matrices. In this guide, we'll explore a fascinating problem: how to compute the sum of digits that surround consecutive 1's in a binary matrix.
The Problem at Hand
Imagine you have a binary matrix filled with 0's and 1's, similar to the example below:
[[See Video to Reveal this Text or Code Snippet]]
In this matrix, your goal is to find consecutive 1's and calculate the sum of any 1's that are positioned directly around these groups. For example, surrounding the 111 found in the fifth row results in a sum of 1.
The Solution Breakdown
To solve this problem, we can employ the power of two popular Python libraries: NumPy and SciPy. Together, they will allow us to efficiently manipulate the matrix and extract the desired data.
Step 1: Set Up Your Environment
Start by importing the necessary libraries:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Create a Random Matrix
We can create a random binary matrix as follows:
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Label the Regions in the Matrix
Using ndimage from SciPy, we can label connected regions in our matrix:
[[See Video to Reveal this Text or Code Snippet]]
This step assigns a unique label to each group of consecutive 1's, making it easier to analyze them individually.
Step 4: Calculate the Surrounding Sums
Now that we have a labeled matrix, we want to identify the number of 1's surrounding each labeled region. We'll do this using binary dilation and logical operations:
[[See Video to Reveal this Text or Code Snippet]]
Summary of the Process
Import Libraries: We utilize NumPy for numerical operations and SciPy for image processing functions.
Create a Matrix: Generate a random binary matrix.
Label Regions: Use a structuring element to label connected components.
Sum Surrounding 1's: For each labeled group, calculate how many 1's surround it and print the result.
Conclusion
This approach demonstrates how powerful NumPy and SciPy can be in handling matrix-related operations. By breaking down the problem into smaller parts, we were able to derive the surrounding sum of 1's in an efficient manner.
We hope you found this guide helpful for your matrix manipulation needs in Python! Happy coding!