filmov
tv
GameMaker Studio 2: Tile Collisions
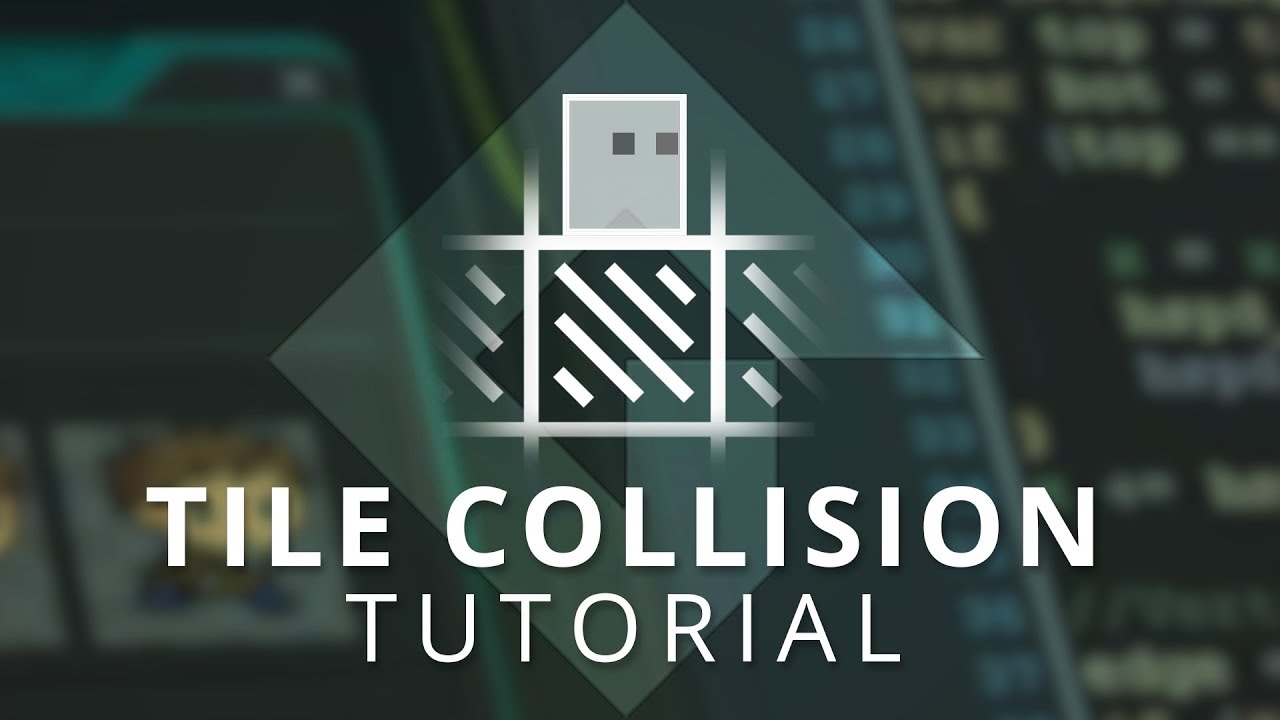
Показать описание
How to collide with a tilemap layer, pixel perfect. Less than 20 lines of code. Slopes and more advanced tile collisions are likely to be covered in a future video.
Easy Tile Collisions | GameMaker
Easy to do tileset collision [Game Maker Studio 2 | Basics]
GameMaker Studio 2 - Super Simple Tile Collisions
GameMaker Studio 2: Tile Collisions
Easily Create Collisions with Tile Maps in this GameMaker Tutorial
GameMaker Studio 2: Tile Collisions
2D sonic engine gamemaker studio 2 precise tile collisions sonic physics guide
Big change to COLLISIONS in GameMaker Studio 2 - v2022.1
How to Move and Collide in GameMaker
Aria Disconnect | Dev Progress | Game Maker | Tile Collisions
GameMaker Studio 2 - TILE COLLISIONS
GameMaker Studio 2: Easy Tile Collision System
TANK - Movement and tile collision - Gamemaker Studio 2
[GameMaker Studio 2] Tiles and Tile Collision - Pac Man (Part Two)
The Ultimate Guide to Collisions in GameMaker
Platformer test in Gamemaker with tile based collision
[Gamemaker] Sloped Tile Collision using Polygons
[Gamemaker] Capsule Collider with Sloped Tile Collisions
How Collisions Work in GameMaker Studio 2
[Gamemaker] Sloped Tile Collisions using Polygons (with rotation!)
Absolute Beginner's Guide Part 12 - Collisions In GameMaker Studio 2
Move And Collide with Slopes - Gamemaker Tutorial
Tile Based Moving Platform Engine for Gamemaker Studio 2 Trailer 1
GameMaker Studio 2 collision display
Комментарии