filmov
tv
How to compare values of multiple fields on button click using jQuery
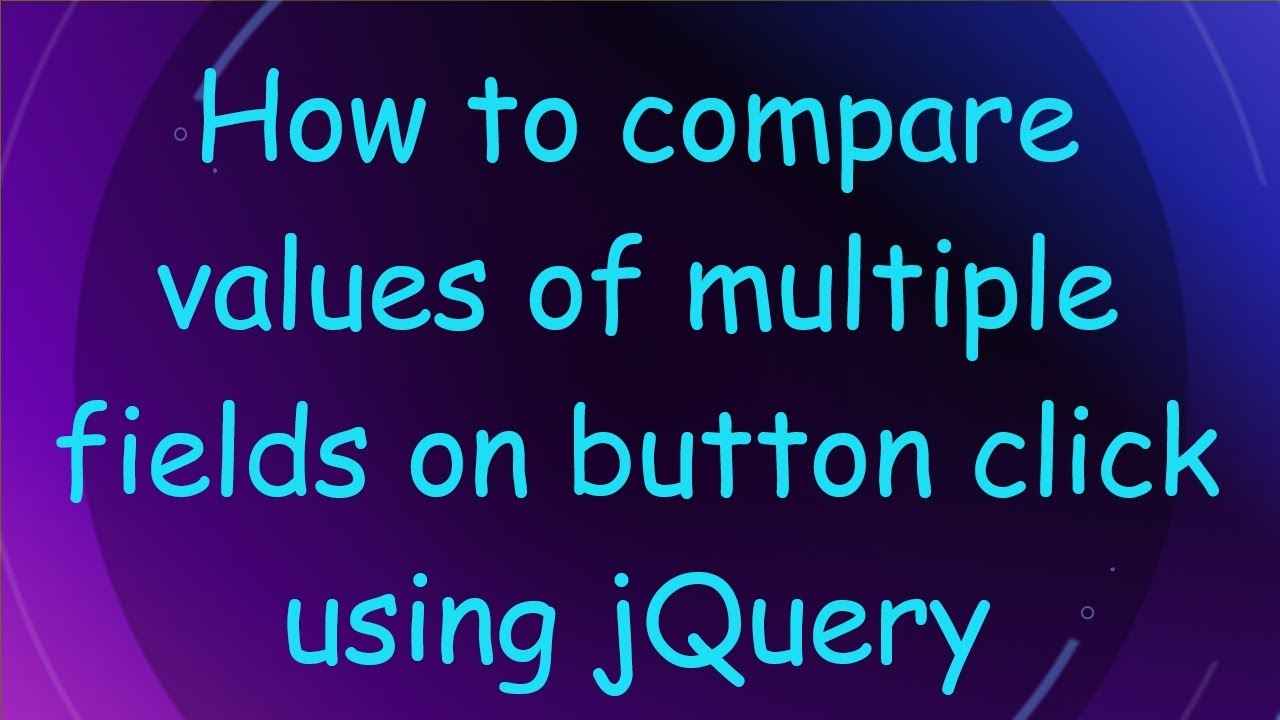
Показать описание
Learn how to efficiently compare the values of multiple fields in a form when a button is clicked, using jQuery. This guide walks you through step-by-step implementation.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to compare the values of all field at a time on button click?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Comparing Multiple Field Values with jQuery
If you're building a web form that allows users to input zip codes, you may want to ensure that the value provided in the first zip code field is always less than the value in the corresponding last zip code field. This functionality not only prevents user errors but also enhances the overall user experience. In this guide, we'll explore how to achieve this using jQuery when a save button is clicked.
The Problem
When you have multiple rows in a form, such as those for entering zip codes, it can become tedious to ensure validations happen for each row. You might be able to validate the first row easily, but how do you handle all of them at once upon clicking a button? This was the challenge posed by a developer, who sought a solution to loop through multiple table rows and compare the values of their fields.
The Solution
To tackle the problem effectively, we rely on jQuery's ability to loop through DOM elements. Here’s how we can implement the comparison logic efficiently:
HTML Structure
Start by structuring your HTML form. We will have a table containing the input fields for zip codes along with a save button:
[[See Video to Reveal this Text or Code Snippet]]
jQuery Logic
Next, add a jQuery script to handle the button click event and loop through each row to compare the field values:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
Button Click Event: The $(".zip-save").on('click', ...) event is triggered when the Save button is clicked. This will initiate the validation process.
Looping Through Rows: $('.zip-table tr').each(function() {...}) allows you to iterate through each row within the table.
Value Extraction: The values of the input fields are extracted using $(this).find('.zip-first').val() and converted to numbers with the + operator.
Validation Logic: A simple if condition checks if the start zip is greater than the end zip. If the condition is true, we provide visual feedback by changing the border color and displaying an error message.
Error Handling: An error message will display if the validation fails.
Conclusion
By following the steps outlined in this post, you can effectively compare values of multiple inputs in your form when a button is clicked. This approach enhances data integrity and improves the overall user experience. With just a few lines of jQuery, you have built a robust solution that handles form validation dynamically across multiple rows.
Remember, user experience is critical in web development, and proper validation is key to achieving that. Enjoy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to compare the values of all field at a time on button click?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Comparing Multiple Field Values with jQuery
If you're building a web form that allows users to input zip codes, you may want to ensure that the value provided in the first zip code field is always less than the value in the corresponding last zip code field. This functionality not only prevents user errors but also enhances the overall user experience. In this guide, we'll explore how to achieve this using jQuery when a save button is clicked.
The Problem
When you have multiple rows in a form, such as those for entering zip codes, it can become tedious to ensure validations happen for each row. You might be able to validate the first row easily, but how do you handle all of them at once upon clicking a button? This was the challenge posed by a developer, who sought a solution to loop through multiple table rows and compare the values of their fields.
The Solution
To tackle the problem effectively, we rely on jQuery's ability to loop through DOM elements. Here’s how we can implement the comparison logic efficiently:
HTML Structure
Start by structuring your HTML form. We will have a table containing the input fields for zip codes along with a save button:
[[See Video to Reveal this Text or Code Snippet]]
jQuery Logic
Next, add a jQuery script to handle the button click event and loop through each row to compare the field values:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
Button Click Event: The $(".zip-save").on('click', ...) event is triggered when the Save button is clicked. This will initiate the validation process.
Looping Through Rows: $('.zip-table tr').each(function() {...}) allows you to iterate through each row within the table.
Value Extraction: The values of the input fields are extracted using $(this).find('.zip-first').val() and converted to numbers with the + operator.
Validation Logic: A simple if condition checks if the start zip is greater than the end zip. If the condition is true, we provide visual feedback by changing the border color and displaying an error message.
Error Handling: An error message will display if the validation fails.
Conclusion
By following the steps outlined in this post, you can effectively compare values of multiple inputs in your form when a button is clicked. This approach enhances data integrity and improves the overall user experience. With just a few lines of jQuery, you have built a robust solution that handles form validation dynamically across multiple rows.
Remember, user experience is critical in web development, and proper validation is key to achieving that. Enjoy coding!