filmov
tv
Java 2D OpenGL RPG Tutorial 3: Creating Items
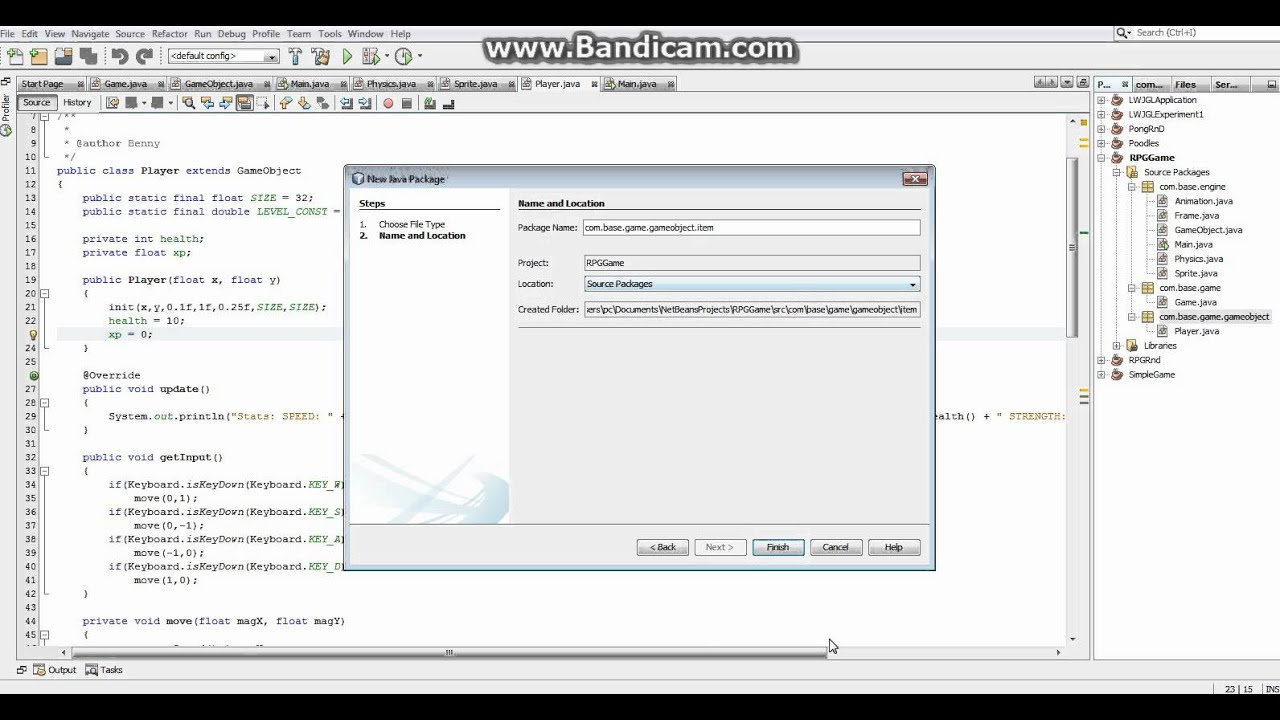
Показать описание
In this video we create a new leveling algorithm and create basic items
Java 2D OpenGL RPG Tutorial 1: Starting off
Java 2D OpenGL RPG Tutorial 2: Setting up the player
Java 2D OpenGL RPG Tutorial 3: Creating Items
Java 2D OpenGL RPG Tutorial 11: Test Level
Java 2D OpenGL RPG Tutorial 7: Getting the player to attack.
Java 2D OpenGL RPG Tutorial 5: Creating Enemies
Java 2D OpenGL RPG Tutorial 4: Inventory
Java 2D OpenGL RPG Tutorial 8: Equipment and Cleanup
Java 2D OpenGL RPG Tutorial 6: Basic Combat and Time Keeping
Java 2D OpenGL RPG Tutorial 9: RPG Mechanics and final Stat System
Java 2D OpenGL RPG Tutorial 10: Random Number Generator Improvements
FPS using ASCII chars test
Best Programming Languages #programming #coding #javascript
What Is The WORST Game Engine?
Start Making Games
The WORST Programming Languages EVER #shorts
How Gamers Think Video Games Are Made...
Code a 2D Game Engine using Java - Full Course for Beginners
How stairs work in top down 2D game worlds
Programming Language Tier List
3D OpenGL RPG Game
#20 Java und OpenGL Tutorial - Einrichtung von Jogl
How to fix your diagonal movement!
programming language, speed compilation #c++ #golang #rust
Комментарии