filmov
tv
Top 10 C# Best Practices (plus bonuses)
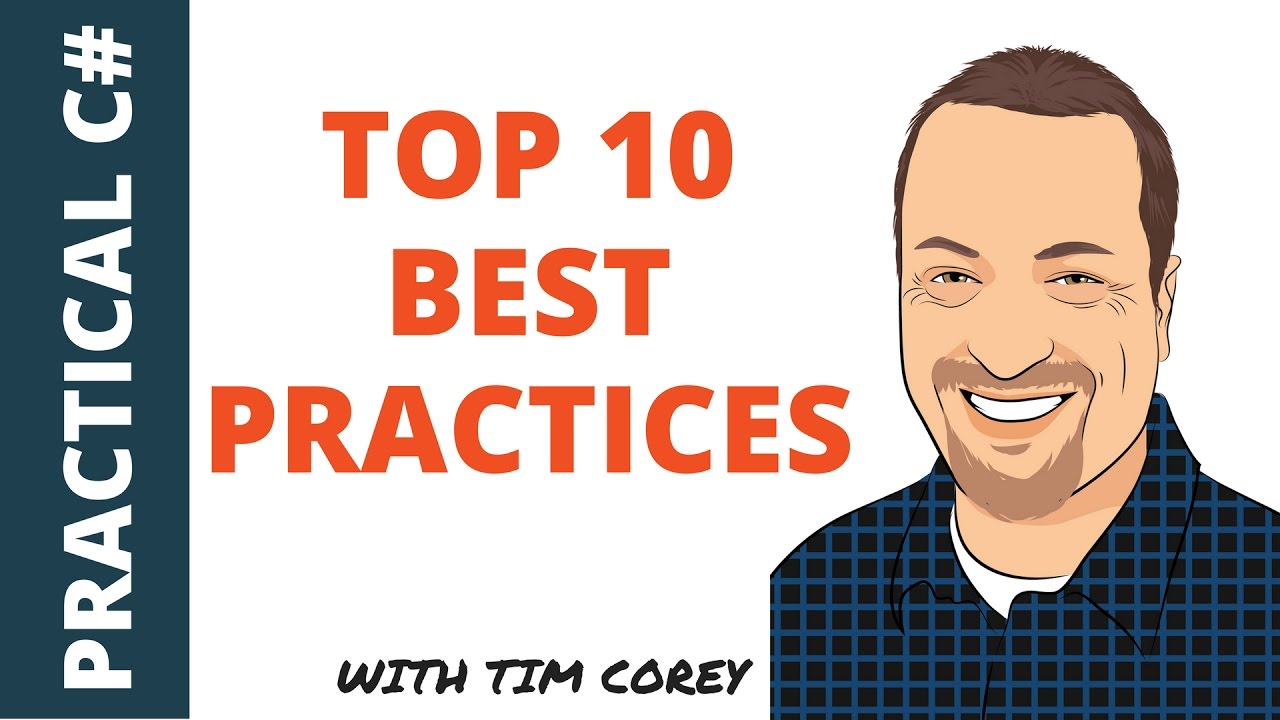
Показать описание
Learn what I believe are the top 10 best practices for C# developers. When you are programming in Visual Studio, I think these will help you do so better, more efficiently, and with less bugs. Once you see all 10 (plus the bonuses at the end), let me know what you think in the comments. Did I miss one? Do you disagree with one?
Thanks to Ralfs K and Julio M, here is a list of the best practices along with their video times:
0:00 - Intro
0:50 - Name things well
7:51 - One class per file
10:18 - Use properties not variables
12:57 - Methods should do one thing
23:27 - Keep it simple
28:21 - Be consistent
32:22 - Use curly braces for if statements
37:45 - Concatenate strings using $""
45:37 - Avoid global variables
48:39 - Use public modifier only when necessary
50:54 - Never trust the user (bonus)
52:24 - Plan before you build
55:31 - Concluding remarks
Top 10 C# Best Practices (plus bonuses)
coding in c until my program is unsafe
TOP 10 PRACTICES I HATE IN C++
C Programming Tutorial 48 - Good Coding Practices
31 nooby C++ habits you need to ditch
How Senior Programmers ACTUALLY Write Code
Tony Robbins One-On-One Coaching Session
The Simple Solution to Traffic
Build Top 10 Best HTML CSS JavaScript Projects for Your Portfolio | Perfect for Beginners in Hindi
Good practice for freeing memory in C
10 Design Patterns Explained in 10 Minutes
If Statement Best Practice | C Programming Tutorial
Go in 100 Seconds
How to Learn to Code FAST (Do This or Keep Struggling)
Simon Sinek’s guide to leadership | MotivationArk
Communication Hack for Connection & Influence | #shorts
If I could give advice to myself when starting as a software engineer
Why good leaders make you feel safe | Simon Sinek | TED
C++ Weekly - Ep 442 - Stop Using .h For C++ Header Files!
25 VS Code Productivity Tips and Speed Hacks
This is what makes employees happy at work | The Way We Work, a TED series
5 Common Mistakes When Installing Video Security System
The 3 Magic Ingredients of Amazing Presentations | Phil WAKNELL | TEDxSaclay
Top 5 Countries With the Highest Mass Attendance | Catholic Documentary | Religion Documentary
Комментарии