filmov
tv
Determine if two strings/phrases are valid Anagrams | Study Algorithms
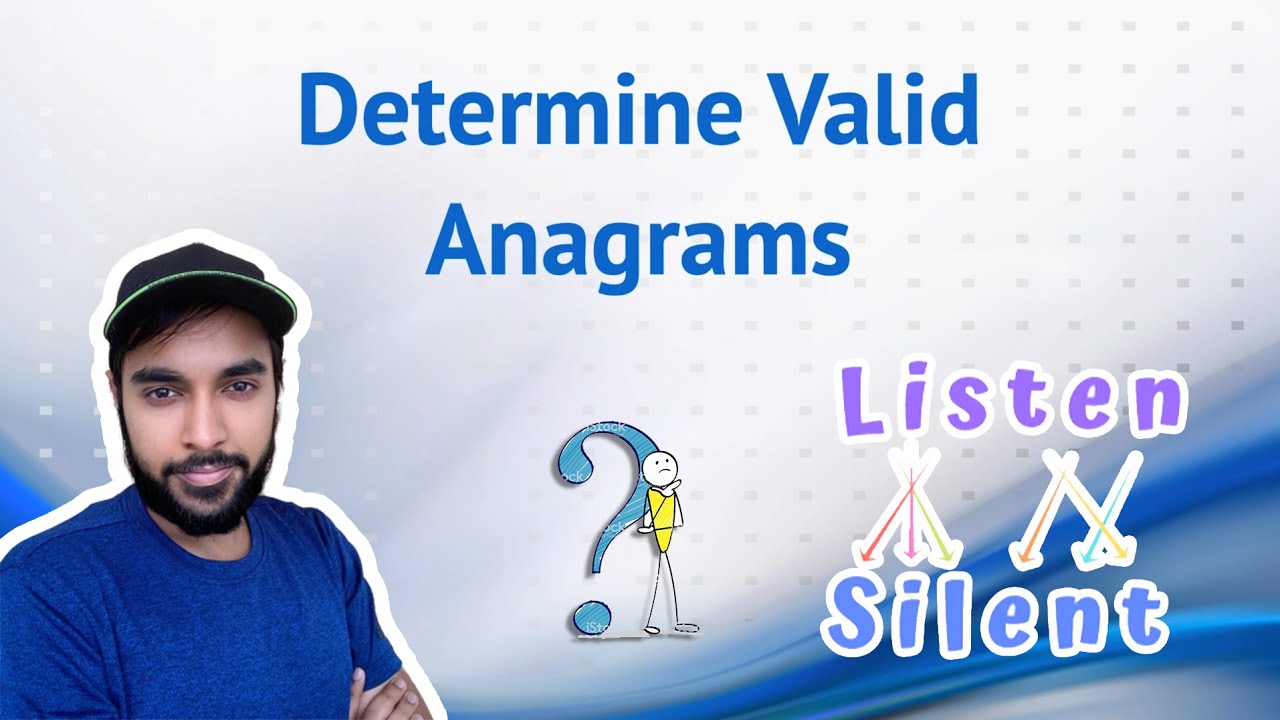
Показать описание
Two words or phrases are said to be anagrams of each other if they can be formed by re-shuffling of characters in one of them. So if you are given two strings str1 and str2. Write a function to determine if str1 is an anagram of str2.
00:00 - Intro
00:26 - Problem description and test cases
01:57 - Brute Force Solution
03:42 - Optimized approach (Solving in O(n))
06:26 - Dry run of the code
Read More:
💻 Get Social 💻
#studyAlgorithms #programming #interview
00:00 - Intro
00:26 - Problem description and test cases
01:57 - Brute Force Solution
03:42 - Optimized approach (Solving in O(n))
06:26 - Dry run of the code
Read More:
💻 Get Social 💻
#studyAlgorithms #programming #interview
Determine if two strings/phrases are valid Anagrams | Study Algorithms
Check if two strings are anagrams
Check if two strings are anagrams
Valid Anagram - Leetcode 242 - Python
Valid Anagram | LeetCode problem 242
Anagram | Check whether two strings are Anagram of each other | Java
Program to Check Whether the Two Strings are Anagram of Each Other or Not - Letsfindcourse
Find All Anagrams in a String - Leetcode 438 - Python
Check If Strings Are Anagrams | C++ Example
Building Search Strings, Part 2: Nesting, Phrase Searching, Truncation, & Wildcards
Check If Two Strings Are Anagrams | Python Example
LeetCode 242. Valid Anagram Solution Explained - Java
An Algorithm A Day - Check If Two Strings Are Anagrams Of Each Other Part 2
Check if two Strings are K Anagrams | Hashmap Interview Questions Playlist
Matching multiline strings between two strings, or how to match across lines
How to check if two strings are anagrams - Java Programming
Check if Two Strings are Anagrams in Python | Step-by-Step Guide
Determine if two Strings are Anagram of each other by counting || java solution
this is the hardest guitar solo ever
1657. Determine if Two Strings Are Close - Day 2/31 Leetcode December Challenge
Check Whether Two Strings Are Anagram Of Each Other | C++ Program
This Bible Verse Could Change Your Entire Day
Fingers still HURT from practising chords? Try THIS
Find All Anagrams in a String | Sliding window | Leetcode #438
Комментарии