filmov
tv
Find All Anagrams in a String | Sliding window | Leetcode #438
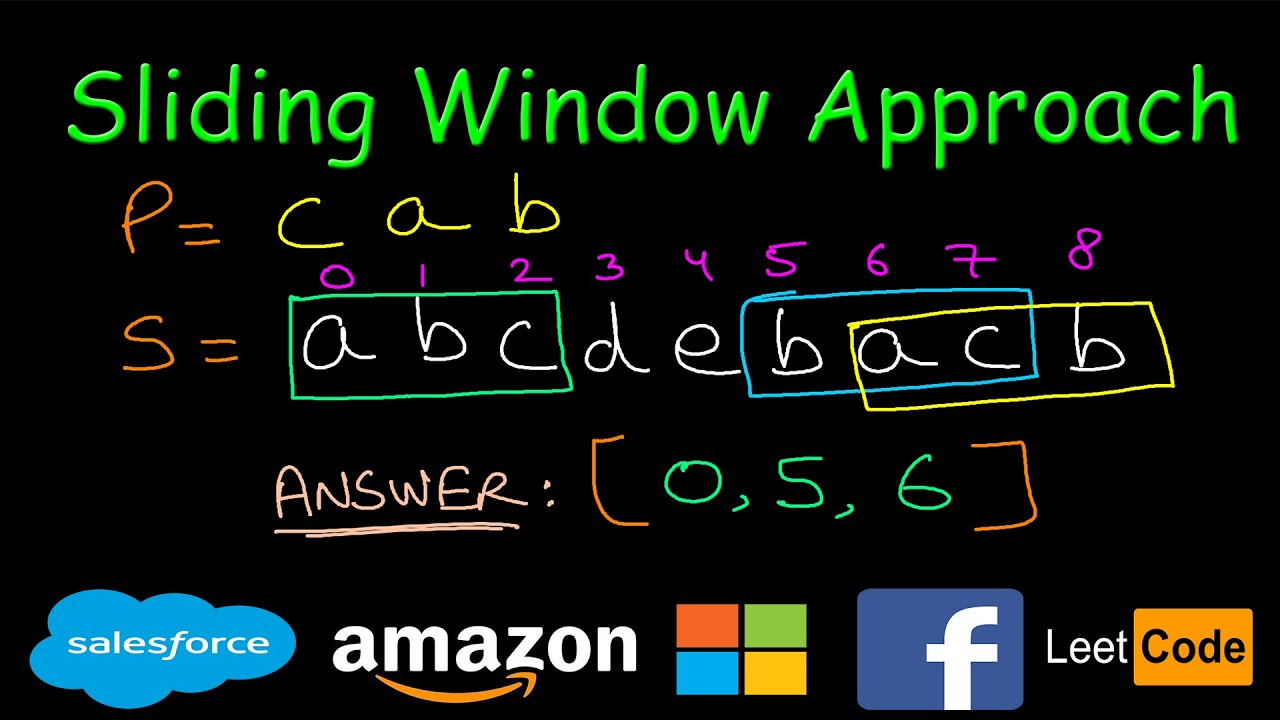
Показать описание
This video explains a very important programming interview problem which is to find all anagrams of a string P in another string S. We need to record the starting indices of all anagrams of string P in string S and then return the recorded array of indices. The most intuitive approach of solving this problem is by using sliding window technique and i have explained the same using examples. At the end of the video, i have explained the code for the sliding window algorithm. CODE LINK is present below as usual. If you find any difficulty or have any query then do COMMENT below. PLEASE help our channel by SUBSCRIBING and LIKE our video if you found it helpful...CYA :)
Find All Anagrams in a String - Leetcode 438 - Python
LeetCode 438. Find All Anagrams in a String (Algorithm Explained)
Find All Anagrams in a String | Sliding window | Leetcode #438
Find All Anagrams in a String | Leetcode - 438
LeetCode | 438. Find All Anagrams in a String | Sliding Window Technique Explained
Leetcode - Find All Anagrams in a String / Permutation in String (Python)
Find all anagrams problem (LeetCode #438) - Inside code
Leetcode Find All Anagrams in A String | Solving AlgoPrep 151 | Nishant Chahar
Find All Anagrams in a String | Leetcode - 438 | Python
438. Find All Anagrams in a String | JavaScript | Hash Map | Sliding Window | Detailed Explanation
Leetcode 438 - Find All Anagrams in a String (JAVA Solution Explained!)
Leetcode Solution - 438 Find All Anagrams in a String
#438: Find All Anagrams in a String - LeetCode Solutions
LeetCode 438 | Find All Anagrams in a String | Medium | C# Solution |
Разбор задачи 438 leetcode.com Find All Anagrams in a String. Решение на C++
Find All Anagrams in a String | Find All Anagrams in a String leetcode | leetcode 438
Find All Anagrams in a String
Leetcode 438. Find All Anagrams in a String Daily Challenge | Sliding Window ,Hashing C++ Full Code
Find All Anagrams in a String | Leetcode 438 | Array
Find All Anagrams In A String | Module : HashMap & Heap | In English | Java | Video_19
Find All Anagrams in a String
Find All Anagrams in a String (Leetcode Medium) | Hashmap Interview Questions Playlist
Find All Anagrams in a String (python) - Day 17/31 Leetcode May Challenge
438. Find All Anagrams in a String - Day 5/28 Leetcode February Challenge
Комментарии