filmov
tv
encoding text with binary
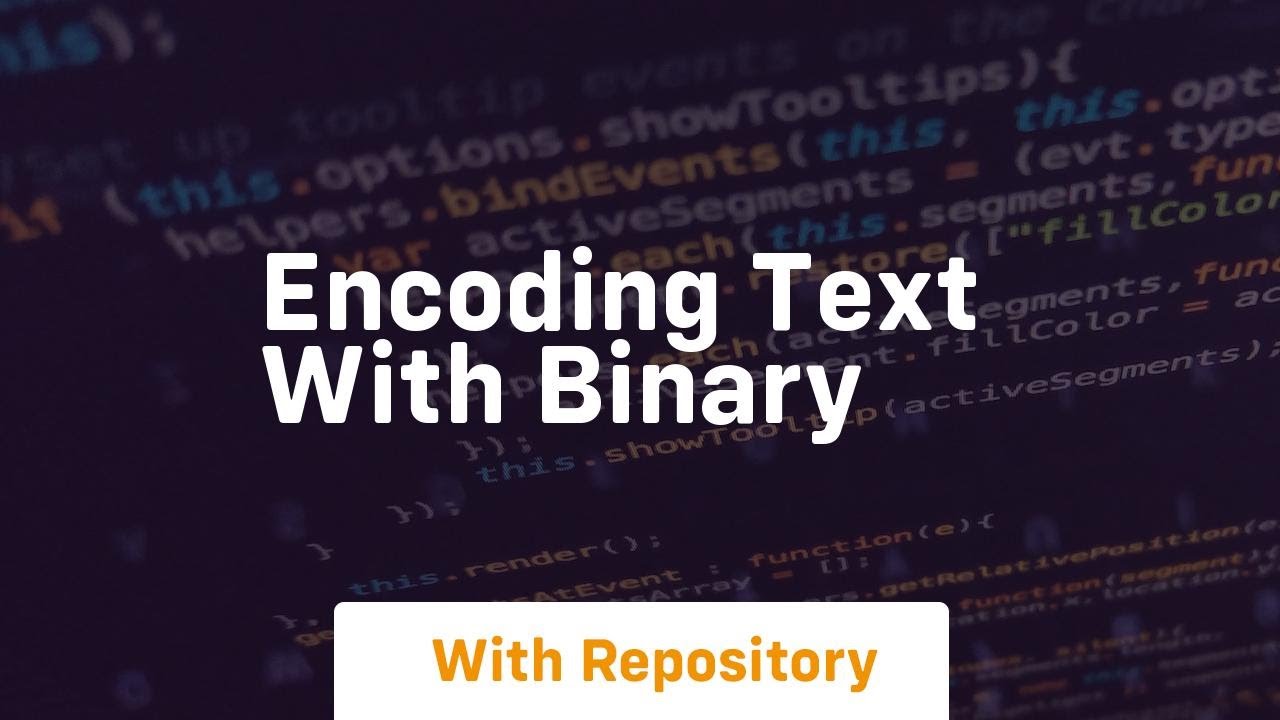
Показать описание
### tutorial: encoding text to binary
encoding text to binary involves converting characters into a format that a computer can understand and process. each character is represented by a binary number, typically using encoding standards like ascii or utf-8. in this tutorial, we’ll focus on ascii encoding and provide a python code example to demonstrate the process.
#### understanding ascii encoding
ascii (american standard code for information interchange) is one of the most common character encoding schemes. each character in the ascii table is represented by a 7-bit binary number, allowing for 128 unique characters, including:
- uppercase letters (a-z)
- lowercase letters (a-z)
- digits (0-9)
- punctuation marks
- control characters (e.g., newline, carriage return)
for example:
- the character 'a' is represented by the decimal number 65, which is `01000001` in binary.
- the character 'a' is represented by the decimal number 97, which is `01100001` in binary.
### encoding text to binary in python
below is a step-by-step guide to encoding a string of text into its binary representation using python.
#### step 1: define the function
we will create a function called `text_to_binary` that takes a string as input and returns its binary representation.
#### step 2: testing the function
now, we can test our function with a sample text:
#### complete code example
here’s the complete code that includes both the function and the test:
### explanation of the code
1. **function definition**: the `text_to_binary` function takes a string as input.
2. **character loop**: it iterates through each character in the string.
3. **ascii conversion**: for each character, it uses `ord(char)` to get the ascii value and `format(..., '08b')` to convert it to an 8-bit binary string.
4. **list of binary strings**: each binary string is appended to a list.
5. **joining the list**: finally, the list of binary strings is joined into a single string with spaces in between for ...
#python binary search
#python binary to string
#python binary search tree
#python binary to decimal
#python binary tree
python binary search
python binary to string
python binary search tree
python binary to decimal
python binary tree
python binary
python binary to int
python binary operators
python binary to hex
python binary number
python encoding errors
python encoding ascii
python encoding types
python encoding cp1252
python encodings module
python encoding list
python encoding utf-8
python encoding
encoding text to binary involves converting characters into a format that a computer can understand and process. each character is represented by a binary number, typically using encoding standards like ascii or utf-8. in this tutorial, we’ll focus on ascii encoding and provide a python code example to demonstrate the process.
#### understanding ascii encoding
ascii (american standard code for information interchange) is one of the most common character encoding schemes. each character in the ascii table is represented by a 7-bit binary number, allowing for 128 unique characters, including:
- uppercase letters (a-z)
- lowercase letters (a-z)
- digits (0-9)
- punctuation marks
- control characters (e.g., newline, carriage return)
for example:
- the character 'a' is represented by the decimal number 65, which is `01000001` in binary.
- the character 'a' is represented by the decimal number 97, which is `01100001` in binary.
### encoding text to binary in python
below is a step-by-step guide to encoding a string of text into its binary representation using python.
#### step 1: define the function
we will create a function called `text_to_binary` that takes a string as input and returns its binary representation.
#### step 2: testing the function
now, we can test our function with a sample text:
#### complete code example
here’s the complete code that includes both the function and the test:
### explanation of the code
1. **function definition**: the `text_to_binary` function takes a string as input.
2. **character loop**: it iterates through each character in the string.
3. **ascii conversion**: for each character, it uses `ord(char)` to get the ascii value and `format(..., '08b')` to convert it to an 8-bit binary string.
4. **list of binary strings**: each binary string is appended to a list.
5. **joining the list**: finally, the list of binary strings is joined into a single string with spaces in between for ...
#python binary search
#python binary to string
#python binary search tree
#python binary to decimal
#python binary tree
python binary search
python binary to string
python binary search tree
python binary to decimal
python binary tree
python binary
python binary to int
python binary operators
python binary to hex
python binary number
python encoding errors
python encoding ascii
python encoding types
python encoding cp1252
python encodings module
python encoding list
python encoding utf-8
python encoding