filmov
tv
Something Is Weird About Rust's Threading and Concurrency | Rust Multi-Threading Tutorial
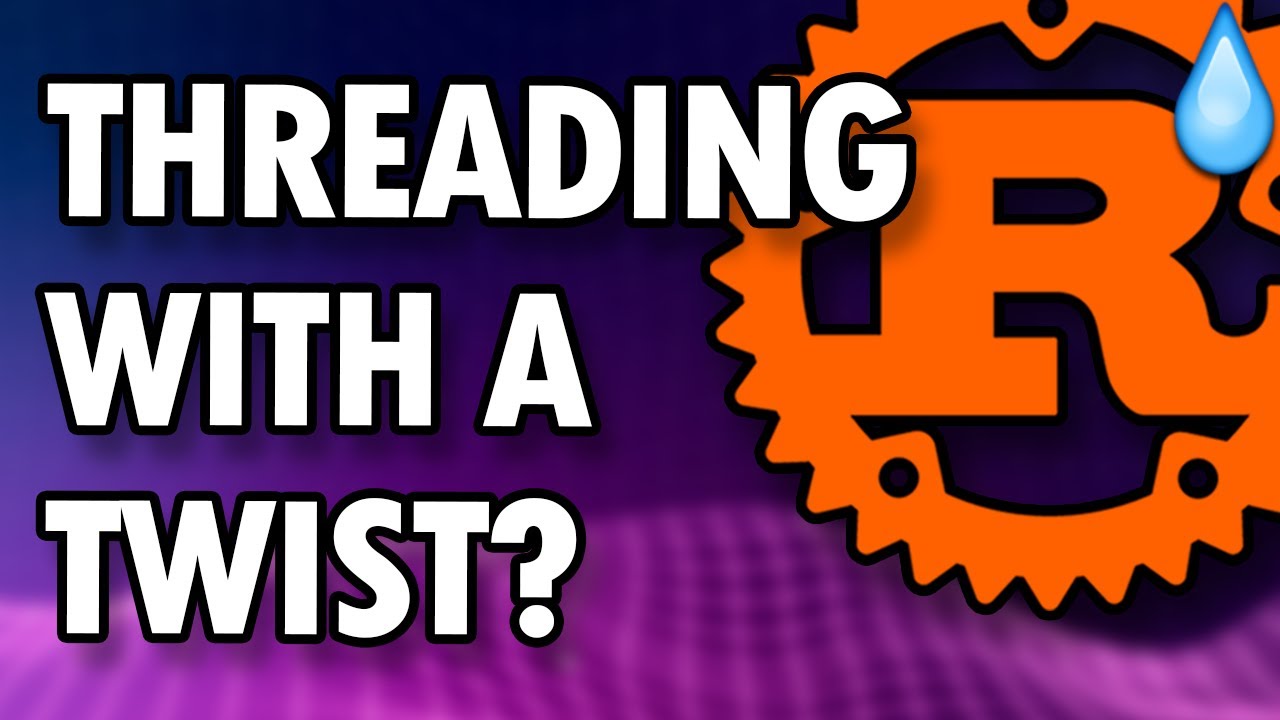
Показать описание
Concurrency and synchronization is an extremely important topic in computer programming. How can I use multi threaded code to execute computationally intensive algorithms? While it's easy to do in most languages, designing a system that allows systems to access the same data at the same time is dangerous and easier said than done.
In this video I teach Multi Threaded Rust Programming, and use a more complicated example to describe how to provide multiple threads access to the same data.
In this video I teach Multi Threaded Rust Programming, and use a more complicated example to describe how to provide multiple threads access to the same data.
Something Is Weird About Rust's Threading and Concurrency | Rust Multi-Threading Tutorial
Weird bug with lockers on rust #shorts
Rust - When Roleplay Gets Weird
RUST WEIRD SINKING & CLASSICAL MUSIC
Strange Guy I Met In Rust #shorts
The Most Modded Servers in Rust
RUST Tutorial - Let´s learn it together - DATA TYPES (video 2)
'Rusty Runtimes: Building Languages In Rust' by Aditya Siram
'Type-Driven API Design in Rust' by Will Crichton
Solving distributed systems challenges in Rust
Rust.into() by Michal Kulagowski
Crust of Rust: std::collections
Weird glitch on rust (PS5) console
Rust Tutorial #8 - Functions, Expressions & Statements
#cs #csgo #steam #кс #ксго #стим #лето #cs2 #rust #steam #cs2strategy #rustclips #cs2skins...
Trying Rust
Rust: Bad for your Car, Good for your Code Base - BSides Winnipeg 2015
Converting `Population` from a Rust type to a trait! Episode 55 of Unhindered by Coding
Rust Traits vs C++ Concepts
Python/C/C++ developer learns Rust from scratch - Chapter 2
RUST Tutorial - Let´s learn it together - Getting started (video 1)
Evening Rust: Ep.21: Onto Super-Tac-Toe
Rust in the Linux Kernel, Uber Security Verdict, Prototype Pollution, PHP Composer - ASW #215
One symptom of a dying Hard Disk Drive (Spinning Rust)
Комментарии