filmov
tv
L46. Check if a tree is a BST or BT | Validate a BST
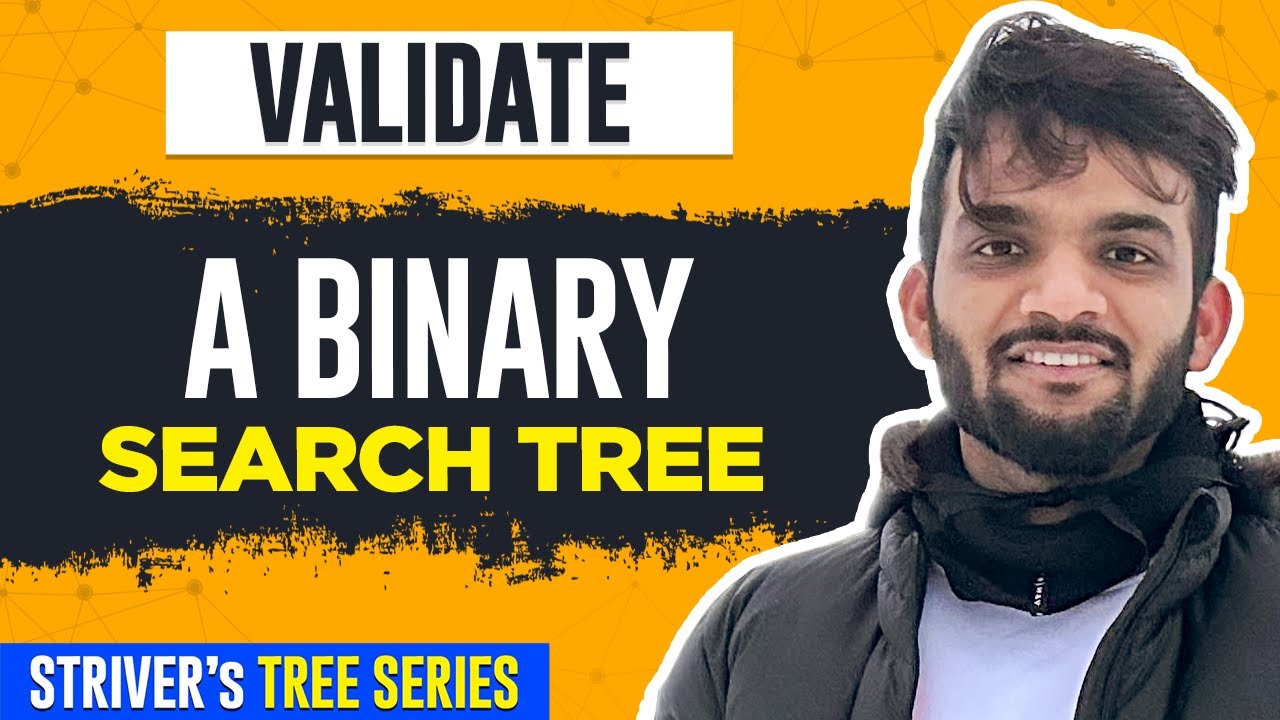
Показать описание
#treeSeries #striver #placements
L46. Check if a tree is a BST or BT | Validate a BST
Validate Binary Search Tree - Depth First Search - Leetcode 98
Check Completeness of a Binary Tree - Leetcode 958 - Python
Leetcode - Validate Binary Search Tree (Python)
Validate Binary Search Tree - LeetCode 98 - Coding Interview Questions
Validate Binary Search Tree (LeetCode 98) | Full solution with animations and visuals
Validate Binary Search Tree | Leetcode 98
BST - 20: Check if given Binary Tree is BST
Check for BST | Validate Binary Search Tree | Validate BST | Check BST | DSA-One Course #69
L15. Check for Balanced Binary Tree | C++ | Java
Validate Binary Search Tree - LeetCode 98 - JavaScript
Check Completeness of a Binary Tree (BFS) - (Meta, Amazon) : Explanation ➕ Live Coding
Validate Binary Tree Nodes - Leetcode 1361 - Python
[Java] Leetcode 98. Validate Binary Search Tree [Binary Search Tree #3]
Leetcode 98 Valide Binary Search Tree
Valid BST from Preorder | Verify Preorder Sequence in Binary Search Tree
#42 Check if a Tree is BST or Not | Data Structures and Algorithms in Java | V. Imp 🔥
LeetCode 98. Validate Binary Search Tree [Algorithm + Code Explained ] Best Solution
GFG POTD: 11/10/2023 | Check for Balanced Tree | Problem of the Day GeeksforGeeks
How To Validate If A Binary Tree Is A Binary Search Tree implemented in C#
Validate Binary Search Tree | Live Coding with Explanation | Leetcode - 98
Validate Binary Search Tree | Java | LeetCode - Medium - 98 | The Code Mate
132 - Is This a Binary Search Tree? | Trees | Hackerrank Solution | Python
Checking if a binary tree is a binary search tree or not!
Комментарии