filmov
tv
LeetCode 98. Validate Binary Search Tree [Algorithm + Code Explained ] Best Solution
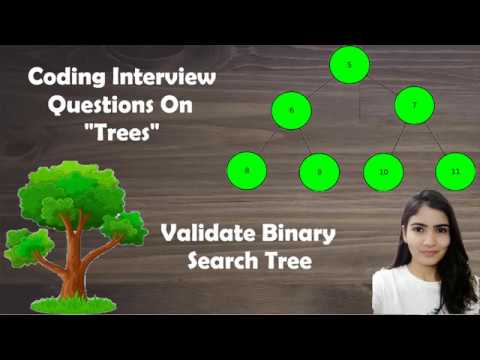
Показать описание
One of the most frequently asked coding interview questions on Trees in companies like Google, Facebook, Amazon, LinkedIn, Microsoft, Uber, Apple, Adobe etc.
LeetCode : Validate Binary Search Tree
Question : Given a binary tree, determine if it is a valid binary search tree (BST).
Assume a BST is defined as follows:
The left subtree of a node contains only nodes with keys less than the node's key.
The right subtree of a node contains only nodes with keys greater than the node's key.
Both the left and right subtrees must also be binary search trees.
Example 1:
2
/ \
1 3
Input: [2,1,3]
Output: true
Example 2:
5
/ \
1 4
/ \
3 6
Input: [5,1,4,null,null,3,6]
Output: false
Explanation: The root node's value is 5 but its right child's value is 4.
LeetCode : Validate Binary Search Tree
Question : Given a binary tree, determine if it is a valid binary search tree (BST).
Assume a BST is defined as follows:
The left subtree of a node contains only nodes with keys less than the node's key.
The right subtree of a node contains only nodes with keys greater than the node's key.
Both the left and right subtrees must also be binary search trees.
Example 1:
2
/ \
1 3
Input: [2,1,3]
Output: true
Example 2:
5
/ \
1 4
/ \
3 6
Input: [5,1,4,null,null,3,6]
Output: false
Explanation: The root node's value is 5 but its right child's value is 4.
Validate Binary Search Tree - Depth First Search - Leetcode 98
Validate Binary Search Tree - Leetcode 98
LeetCode 98. Validate Binary Search Tree (Algorithm Explained)
LeetCode 98. Validate Binary Search Tree - Interview Prep Ep 69
Validate Binary Search Tree - Leetcode 98
Validate Binary Search Tree (LeetCode 98) | Full solution with animations and visuals
Validate Binary Search Tree - Leetcode 98
Validate Binary Search Tree | Live Coding with Explanation | Leetcode - 98
[Java] Leetcode 98. Validate Binary Search Tree [Binary Search Tree #3]
Leetcode - Validate Binary Search Tree (Python)
Validate Binary Search Tree - Leetcode 98 - Trees (Python)
Validate Binary Search Tree - LeetCode 98 - JavaScript
Leetcode 98: Validate Binary Search Tree VISUALIZED
Validate Binary Search Tree | Leetcode 98
FAANG BST QUESTION! | Validate Binary Search Tree - Leetcode 98
Leetcode Q98: Validate Binary Search Tree Solution VISUALIZED
LeetCode Interview Problem | Validate Binary Search Tree
Validate Binary Search Tree
Leetcode 98: Validate Binary Search Tree
Leetcode 98 Validate Binary Search Tree (Java)
Leetcode 98 - Validate Binary Search Tree (JAVA Solution Explained!)
Validate Binary Search Tree - LeetCode 98 - Coding Interview Questions
Validate Binary Search Tree Leetcode Recursion 3 line solution
Software Engineer/Developer Interview Question: 98. Validate Binary Search Tree
Комментарии