filmov
tv
Understanding Matrix Multiplication in Python: The Role of range(len(B[0]))
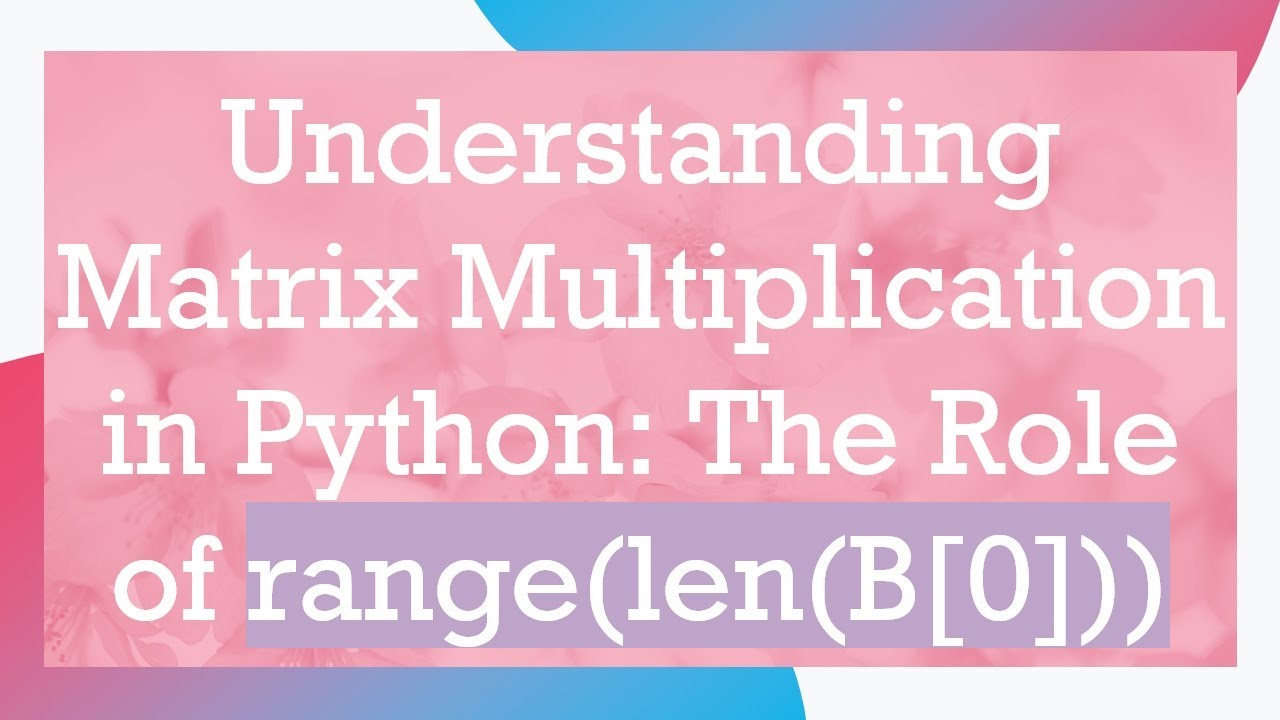
Показать описание
Summary: Dive into the specifics of why `range(len(B[0]))` is used in the second 'for' loop during matrix multiplication in Python, catering to intermediate and advanced users.
---
Understanding Matrix Multiplication in Python: The Role of range(len(B[0]))
Matrix multiplication is a fundamental operation in various fields such as data science, engineering, and computer graphics. If you’re working with Python, particularly with mathematical models or algorithms, understanding the mechanics behind matrix multiplication is crucial. In this guide, we’ll break down why the second 'for' loop in matrix multiplication uses range(len(B[0])).
Matrix multiplication involves taking two matrices, A and B, and producing a third matrix, C. Given two matrices:
A with dimensions m x n
B with dimensions n x p
The resulting matrix C will have dimensions m x p.
The For Loops in Matrix Multiplication
In Python, you can implement matrix multiplication using nested for loops. Let’s consider the following code snippet for clarity:
[[See Video to Reveal this Text or Code Snippet]]
The Crucial Role of range(len(B[0]))
Understanding why we use range(len(B[0])) in the second 'for' loop requires a closer look at the structure of matrix B.
len(B) gives the number of rows in matrix B.
len(B[0]) gives the number of columns in matrix B.
In the context of matrix multiplication, the second for loop iterates over the number of columns in matrix B. Here’s why:
Column Handling:
To compute the elements of the new matrix C, we need to multiply and accumulate the products of corresponding elements from a row in A and a column in B. This necessitates iteration over each column in B.
Resultant Matrix Dimensions:
Since the resultant matrix C should have p columns (the same number of columns as B), the loop must iterate over that number, which is obtained using len(B[0]).
Element Positioning in Matrix C:
Correctly placing the result of the dot product of row i from matrix A and column j from matrix B into the position C[i][j] in the resultant matrix.
Visualizing the Process
Consider the first element of matrix C, C[0][0]:
First Iteration (i=0, j=0):
Loop over k=0 to k=2 (since range(len(B)) translates to range(3)).
Compute and accumulate:
C[0][0] += A[0][0]*B[0][0] + A[0][1]*B[1][0] + A[0][2]*B[2][0].
Each element C[i][j] is then computed similarly, with the inner loop managing the summation of products of the row elements from A and column elements from B.
Conclusion
Understanding the use of range(len(B[0])) in the second 'for' loop in matrix multiplication is crucial for correctly implementing the algorithm in Python. This indexing ensures that the resulting matrix C has dimensions m x p, as intended. Whether you’re delving into data processing or advanced algorithms, this fundamental concept will prove indispensable.
Happy coding!
---
Understanding Matrix Multiplication in Python: The Role of range(len(B[0]))
Matrix multiplication is a fundamental operation in various fields such as data science, engineering, and computer graphics. If you’re working with Python, particularly with mathematical models or algorithms, understanding the mechanics behind matrix multiplication is crucial. In this guide, we’ll break down why the second 'for' loop in matrix multiplication uses range(len(B[0])).
Matrix multiplication involves taking two matrices, A and B, and producing a third matrix, C. Given two matrices:
A with dimensions m x n
B with dimensions n x p
The resulting matrix C will have dimensions m x p.
The For Loops in Matrix Multiplication
In Python, you can implement matrix multiplication using nested for loops. Let’s consider the following code snippet for clarity:
[[See Video to Reveal this Text or Code Snippet]]
The Crucial Role of range(len(B[0]))
Understanding why we use range(len(B[0])) in the second 'for' loop requires a closer look at the structure of matrix B.
len(B) gives the number of rows in matrix B.
len(B[0]) gives the number of columns in matrix B.
In the context of matrix multiplication, the second for loop iterates over the number of columns in matrix B. Here’s why:
Column Handling:
To compute the elements of the new matrix C, we need to multiply and accumulate the products of corresponding elements from a row in A and a column in B. This necessitates iteration over each column in B.
Resultant Matrix Dimensions:
Since the resultant matrix C should have p columns (the same number of columns as B), the loop must iterate over that number, which is obtained using len(B[0]).
Element Positioning in Matrix C:
Correctly placing the result of the dot product of row i from matrix A and column j from matrix B into the position C[i][j] in the resultant matrix.
Visualizing the Process
Consider the first element of matrix C, C[0][0]:
First Iteration (i=0, j=0):
Loop over k=0 to k=2 (since range(len(B)) translates to range(3)).
Compute and accumulate:
C[0][0] += A[0][0]*B[0][0] + A[0][1]*B[1][0] + A[0][2]*B[2][0].
Each element C[i][j] is then computed similarly, with the inner loop managing the summation of products of the row elements from A and column elements from B.
Conclusion
Understanding the use of range(len(B[0])) in the second 'for' loop in matrix multiplication is crucial for correctly implementing the algorithm in Python. This indexing ensures that the resulting matrix C has dimensions m x p, as intended. Whether you’re delving into data processing or advanced algorithms, this fundamental concept will prove indispensable.
Happy coding!