filmov
tv
How to Implement Debounce in Fetch Function with useQuery in React JS
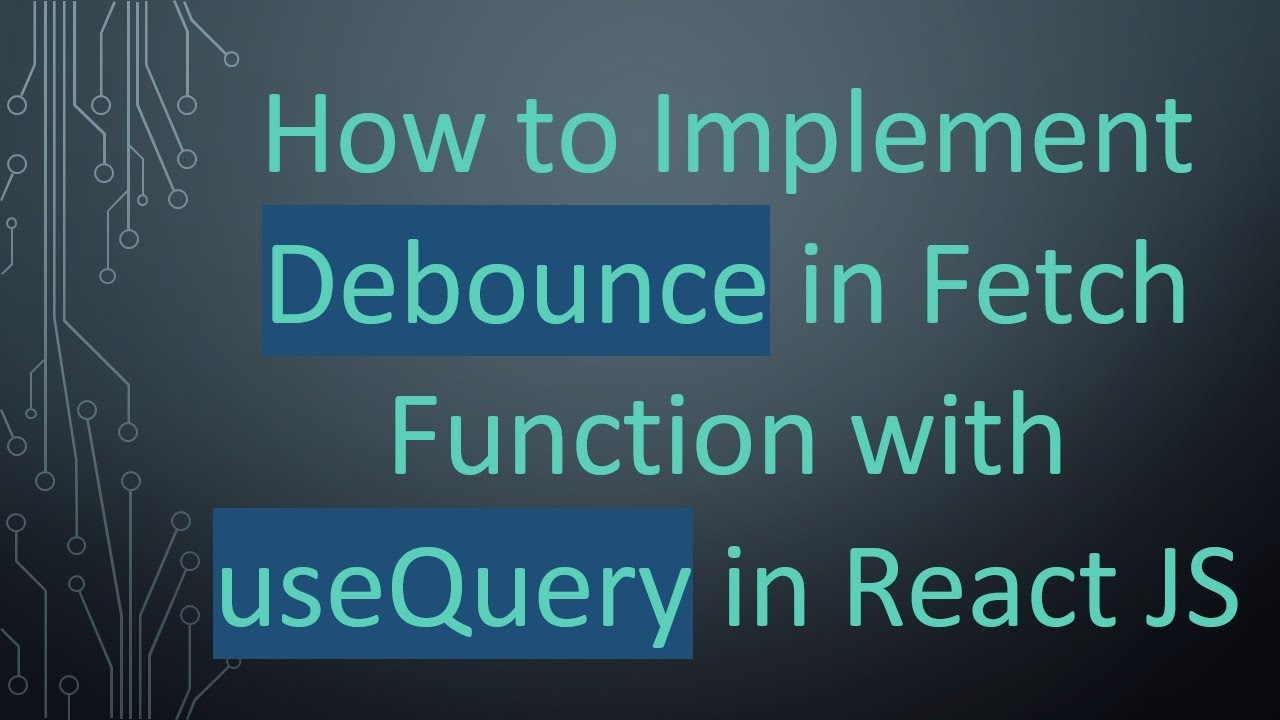
Показать описание
Learn how to effectively implement `debounce` in your fetch function using `useQuery` in React JS, enhancing your API data retrieval with controlled delays.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to debounce in fetch function in useQuery?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mastering Debounce in Fetch Function with useQuery in React JS
When building applications with React JS, especially those that consume APIs, managing data fetching efficiently is crucial. One common challenge developers face is handling API requests in response to user input. If you're looking to implement debounce in your fetch function using React Query's useQuery, you’re in the right place! This guide will walk you through the steps needed to achieve this with ease.
Understanding the Problem
In your application, you may have a data fetching function that retrieves data from an API as the user types in a search query. However, frequent changes to this query can lead to multiple rapid requests, which might overwhelm the API or lead to an unpleasant user experience. The solution? Debouncing the input so that the fetch function only triggers after a specified delay.
Your Current Setup
You already have a basic setup for fetching data using useQuery. Here’s a quick overview:
[[See Video to Reveal this Text or Code Snippet]]
In this code, you're fetching data when the search query changes. However, to optimize this, we need to implement debouncing on the searchQuery to minimize unnecessary fetch calls.
Implementing Debounce in useQuery
Step 1: Create a Custom Hook
To debounce the search query effectively, we can create a custom hook. This hook will use useDebounce to delay the updates to the searchQuery, allowing us to control when the fetch function is called.
Here’s an example of how you can structure the custom hook:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Use the Custom Hook in Your Component
Now that you have your custom hook ready, it's time to use it in your components. You can specify the debounce duration as an argument, allowing you to fine-tune the delay to your needs.
Here's how to implement it in a component:
[[See Video to Reveal this Text or Code Snippet]]
Key Benefits of Debouncing
Reduced API Calls: By minimizing the number of requests, we reduce the load on the server and enhance app performance.
Improved User Experience: Users see updates only after they've stopped typing, which can lead to a smoother experience.
Efficient Resource Management: This approach helps in managing resources effectively while working with APIs.
Conclusion
Incorporating debounce into your data fetching with useQuery can greatly enhance your React applications, providing a better experience for users while ensuring efficient resource usage. By following the steps outlined above, you can set up a system that only fetches data when necessary, keeping your application responsive and user-friendly.
If you're interested in learning more about React Query or need help with other topics, feel free to reach out in the comments! Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to debounce in fetch function in useQuery?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mastering Debounce in Fetch Function with useQuery in React JS
When building applications with React JS, especially those that consume APIs, managing data fetching efficiently is crucial. One common challenge developers face is handling API requests in response to user input. If you're looking to implement debounce in your fetch function using React Query's useQuery, you’re in the right place! This guide will walk you through the steps needed to achieve this with ease.
Understanding the Problem
In your application, you may have a data fetching function that retrieves data from an API as the user types in a search query. However, frequent changes to this query can lead to multiple rapid requests, which might overwhelm the API or lead to an unpleasant user experience. The solution? Debouncing the input so that the fetch function only triggers after a specified delay.
Your Current Setup
You already have a basic setup for fetching data using useQuery. Here’s a quick overview:
[[See Video to Reveal this Text or Code Snippet]]
In this code, you're fetching data when the search query changes. However, to optimize this, we need to implement debouncing on the searchQuery to minimize unnecessary fetch calls.
Implementing Debounce in useQuery
Step 1: Create a Custom Hook
To debounce the search query effectively, we can create a custom hook. This hook will use useDebounce to delay the updates to the searchQuery, allowing us to control when the fetch function is called.
Here’s an example of how you can structure the custom hook:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Use the Custom Hook in Your Component
Now that you have your custom hook ready, it's time to use it in your components. You can specify the debounce duration as an argument, allowing you to fine-tune the delay to your needs.
Here's how to implement it in a component:
[[See Video to Reveal this Text or Code Snippet]]
Key Benefits of Debouncing
Reduced API Calls: By minimizing the number of requests, we reduce the load on the server and enhance app performance.
Improved User Experience: Users see updates only after they've stopped typing, which can lead to a smoother experience.
Efficient Resource Management: This approach helps in managing resources effectively while working with APIs.
Conclusion
Incorporating debounce into your data fetching with useQuery can greatly enhance your React applications, providing a better experience for users while ensuring efficient resource usage. By following the steps outlined above, you can set up a system that only fetches data when necessary, keeping your application responsive and user-friendly.
If you're interested in learning more about React Query or need help with other topics, feel free to reach out in the comments! Happy coding!