filmov
tv
Find the Longest Continuous Increasing Subsequence in Array | Longest Increasing Subsequence Problem
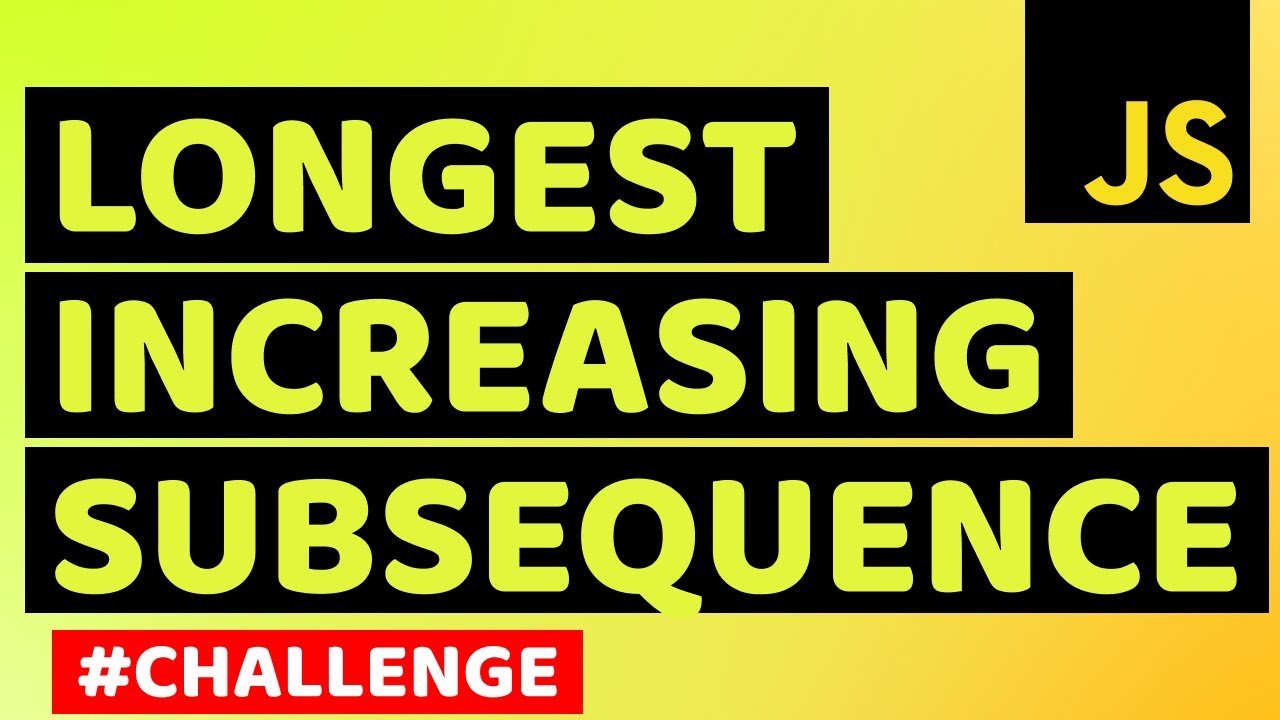
Показать описание
Find longest increasing subsequence in an array. The longest increasing subsequence (LIS) or longest increasing subsequence problem is the length of the longest subsequence of a given sequence such that all elements of the subsequence are sorted in increasing order. We have to write a function that finds the longest increasing subsequence in an array using javascript.
Here is one approach to find the longest increasing subsequence in an array using dynamic programming in JavaScript. Define a function that takes an array as an argument. If the length of the array is 0, return 0, as there is no increasing subsequence in an empty array.
Define a variable 'dp' that will be an array filled with the value 1, with the same length as the input array arr. This array will be used to store the length of the longest increasing subsequence ending at each index.
Initialize a variable 'maxLength' to 1 as a single element is always a valid increasing subsequence. Start a for loop that iterates through the input array from the second element to the last. Start a second for loop that iterates through the array from the first element.
If the current element 'arr[i]'' is greater than 'arr[j]', update the value of 'dp[i]' to the maximum of its current value and 'dp[j]+1'. This means the current element 'arr[i]' can be added to the end of an increasing subsequence ending at 'arr[j]'. Update the maxLength variable to the maximum of its current value and dp[i] after each iteration of the inner loop. This is to keep track of the maximum length of the increasing subsequence found so far.
After both loops end, return the maxLength which represents the length of the longest increasing subsequence in the input array. Define an array. Call the function with this array. Length of longest increasing subsequence in given array will be the output.
So this is how we can find the length of longest increasing subsequence in a given array using javascript.
* Full Playlist (Coding Challenge, Interview Questions & Leetcode) *
It can be a good javascript interview question or frontend interview question. You may not be required to solve it on paper or whiteboard but the interviewer may ask you to give an idea on how to approach this algorithm. If you have an understanding of how to solve this problem or approach this algorithm, you will be able to answer it and get your next job as a frontend developer or full-stack developer.
Our tutorials help you to improve your career growth, perform better in your job and make money online as a freelancer. Learn the skills to build and design professional websites, and create dynamic and interactive web applications using JavaScript, or WordPress. Our tutorials are tailored to help beginners and professionals alike. Whether you're just starting in the field or you're looking to expand your knowledge, we've got something for you. Join us on this journey to becoming a skilled web developer. Subscribe to our channel and let's get started!
Thank You!
👍 LIKE VIDEO
👊 SUBSCRIBE
🔔 PRESS BELL ICON
✍️ COMMENT
#js #javascript #challenge #codingchallenge #javascriptinterviewquestions #javascripttutorial #leetcode #coding #programming #computerscience #algorithm #WebStylePress #WebDevelopment
Here is one approach to find the longest increasing subsequence in an array using dynamic programming in JavaScript. Define a function that takes an array as an argument. If the length of the array is 0, return 0, as there is no increasing subsequence in an empty array.
Define a variable 'dp' that will be an array filled with the value 1, with the same length as the input array arr. This array will be used to store the length of the longest increasing subsequence ending at each index.
Initialize a variable 'maxLength' to 1 as a single element is always a valid increasing subsequence. Start a for loop that iterates through the input array from the second element to the last. Start a second for loop that iterates through the array from the first element.
If the current element 'arr[i]'' is greater than 'arr[j]', update the value of 'dp[i]' to the maximum of its current value and 'dp[j]+1'. This means the current element 'arr[i]' can be added to the end of an increasing subsequence ending at 'arr[j]'. Update the maxLength variable to the maximum of its current value and dp[i] after each iteration of the inner loop. This is to keep track of the maximum length of the increasing subsequence found so far.
After both loops end, return the maxLength which represents the length of the longest increasing subsequence in the input array. Define an array. Call the function with this array. Length of longest increasing subsequence in given array will be the output.
So this is how we can find the length of longest increasing subsequence in a given array using javascript.
* Full Playlist (Coding Challenge, Interview Questions & Leetcode) *
It can be a good javascript interview question or frontend interview question. You may not be required to solve it on paper or whiteboard but the interviewer may ask you to give an idea on how to approach this algorithm. If you have an understanding of how to solve this problem or approach this algorithm, you will be able to answer it and get your next job as a frontend developer or full-stack developer.
Our tutorials help you to improve your career growth, perform better in your job and make money online as a freelancer. Learn the skills to build and design professional websites, and create dynamic and interactive web applications using JavaScript, or WordPress. Our tutorials are tailored to help beginners and professionals alike. Whether you're just starting in the field or you're looking to expand your knowledge, we've got something for you. Join us on this journey to becoming a skilled web developer. Subscribe to our channel and let's get started!
Thank You!
👍 LIKE VIDEO
👊 SUBSCRIBE
🔔 PRESS BELL ICON
✍️ COMMENT
#js #javascript #challenge #codingchallenge #javascriptinterviewquestions #javascripttutorial #leetcode #coding #programming #computerscience #algorithm #WebStylePress #WebDevelopment
Комментарии