filmov
tv
Longest Consecutive Sequence (LeetCode 128) | Full solution quick and easy explanation | Interviews
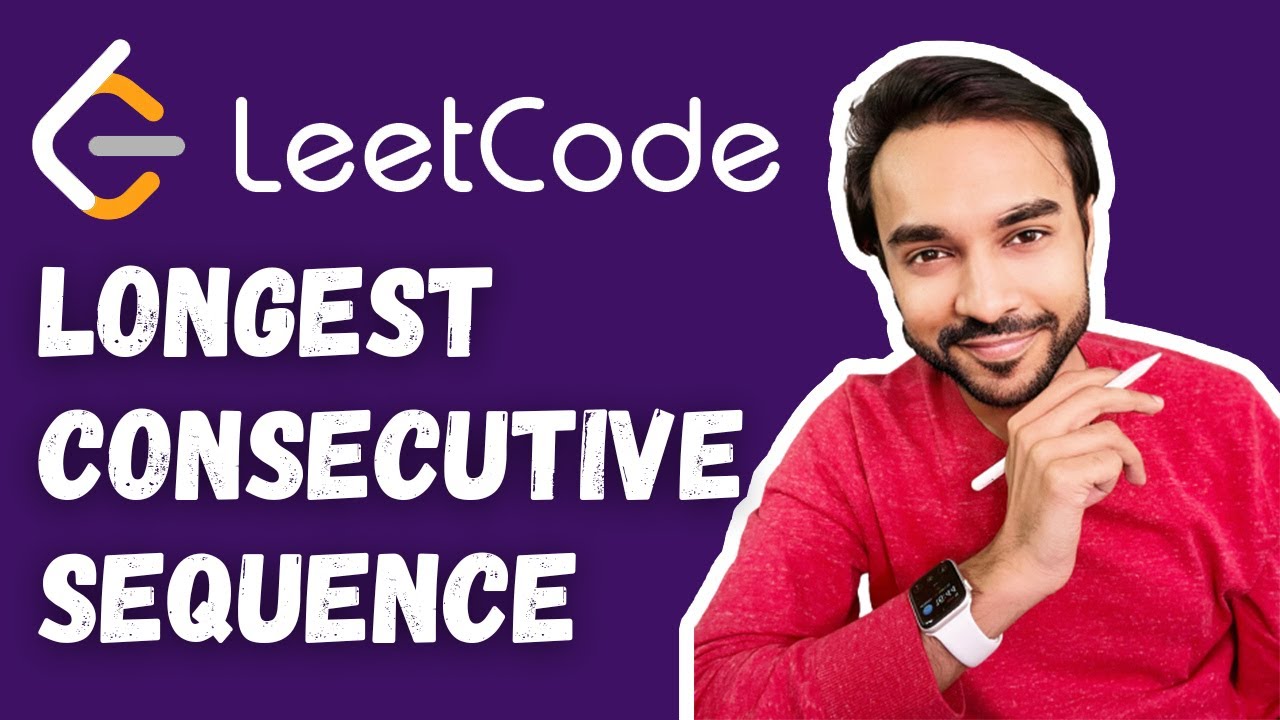
Показать описание
As direct this problem looks, the trickier it is to solve in O(n) time complexity. In this video learn how to build a better solution on top of a brute force solution and how to determine which data structure will be a good choice. This will speed up how you find the longest consecutive sequence. All along with beautiful animations and visuals.
Chapters:
00:00 - Intro
00:59 - Problem Statement and Description
03:17 - Brute Force Method
05:54 - Sorting to the rescue
08:21 - Optimizing for O(n)
14:02 - Dry-run of Code
17:21 - Final Thoughts
📚 Links to topics I talk about in the video:
📖 Reference Books:
🎥 My Recording Gear:
💻 Get Social 💻
#leetcode #programming #interview
Chapters:
00:00 - Intro
00:59 - Problem Statement and Description
03:17 - Brute Force Method
05:54 - Sorting to the rescue
08:21 - Optimizing for O(n)
14:02 - Dry-run of Code
17:21 - Final Thoughts
📚 Links to topics I talk about in the video:
📖 Reference Books:
🎥 My Recording Gear:
💻 Get Social 💻
#leetcode #programming #interview
Leetcode 128 - LONGEST CONSECUTIVE SEQUENCE
Longest Consecutive Sequence (LeetCode 128) | Full solution quick and easy explanation | Interviews
Longest Consecutive Sequence - Leetcode 128
Longest Consecutive Sequence - Leetcode 128 - Hashmaps & Sets (Python)
LeetCode 128 'HARD' - Longest Consecutive Sequence
Leetcode - Longest Consecutive Sequence (Python)
Leetcode 128. Longest Consecutive Sequence || Intutition + Code + Explanation
Leetcode Solution - 128 Longest Consecutive Sequence
Leetcode 128. Longest Consecutive Sequence [ JAVASCRIPT ].
Longest Consecutive Sequence - LeetCode 128 - Java
Longest Consecutive Sequence - Leetcode 128 - Blind 75 Explained - Graphs - Python
128. Longest Consecutive Sequence - Day 5/31 Leetcode July Challenge
Amazon Interview Question - Longest Consecutive Sequence - LeetCode 128
Longest Consecutive Sequence | LeetCode 128 | Theory + Python code
Longest Consecutive Sequence - Leetcode 128
Leetcode 128. Longest Consecutive Sequence (union find)
LEETCODE 128: LONGEST CONSECUTIVE SEQUENCE: PREV IN SET PATTERN
Amazon Interview Question! | Longest Consecutive Sequence - Leetcode 128
Longest Consecutive Sequence Leetcode 128 Javascript
LeetCode #128: Longest Consecutive Sequence | Hash Set
Longest Consecutive Sequence - Leetcode 128
Longest Consecutive Sequence | Leetcode 128 | Hashset
Longest Consecutive Sequence | LeetCode 128 | Coding Interview Tutorial
Longest Consecutive Sequence | Google Interview Question | Brute Better Optimal
Комментарии