filmov
tv
Maximum Subarray - Amazon Coding Interview Question - Leetcode 53 - Python
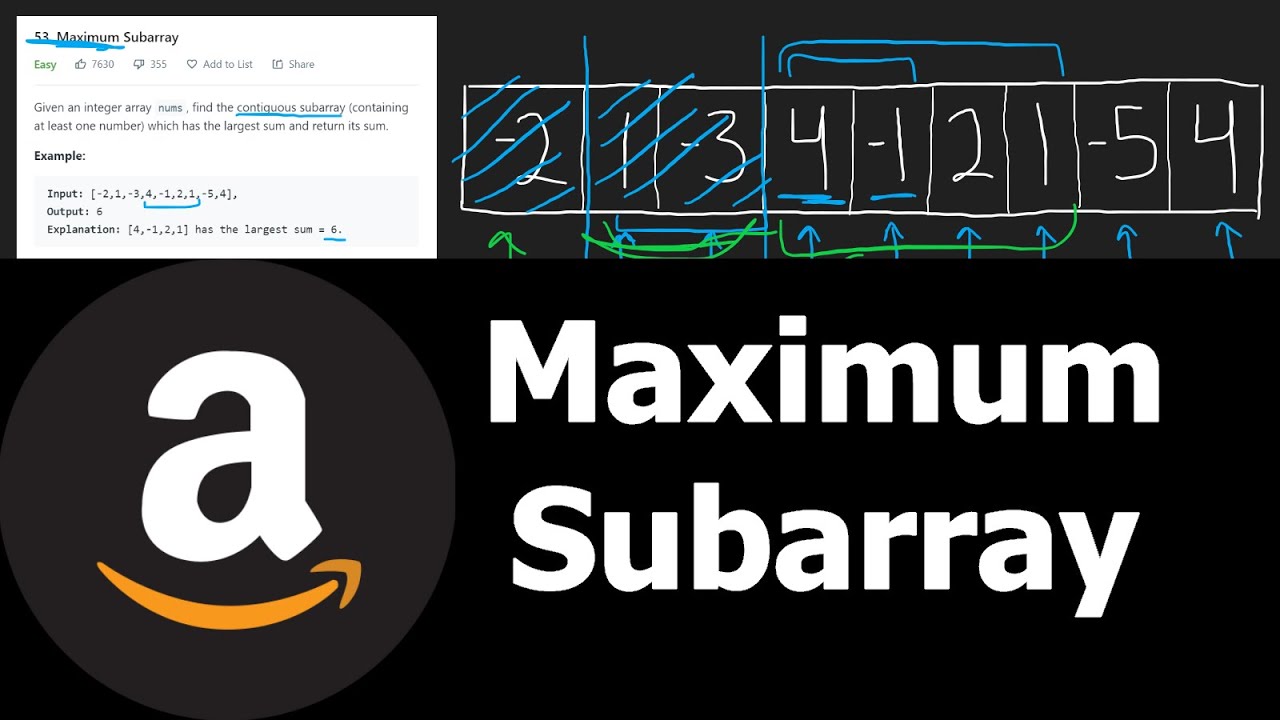
Показать описание
0:00 - Cubic solution
2:02 - Quadratic solution
3:10 - Linear solution
6:37 - Coding
#Coding #Programming #CodingInterview
Disclosure: Some of the links above may be affiliate links, from which I may earn a small commission.
Maximum Subarray - Amazon Coding Interview Question - Leetcode 53 - Python
Kadane's Algorithm - Maximum Sum Subarray (Amazon Coding Interview Question)
Kadane's maximum subarray sum #animation
Amazon Coding Interview Question - Maximum Sum Subarray
Maximum Subarray - LeetCode 53 - Coding Interview Questions
Problem Asked in Amazon Interview | Maximum Subarray Sum
Maximum Subarray Amazon, Google, Facebook Coding Interview Question - Leetcode 53
Kadane's Algorithm for Maximum subarray - Amazon coding interview question
Kadane's Algorithm - Maximum Subarray (Dynamic Programming)
Maximum Subarray Amazon Coding Interview Question
Amazon Coding Interview Question | Leetcode 53 | Maximum Subarray
Kadane's Algorithm - Maximum Sum Subarray(Amazon Coding Interview Question)|leetcode 53 |#leetc...
Maximum Subarray - Amazon Coding Interview Question - Leetcode 53 - Java
LeetCode 53: Maximum Subarray Problem | DSA | Hack Code | Amazon Question
Amazon, Microsoft, MakeMyTrip Interview Question: Find max subarray length with sum zero
MAXIMUM PRODUCT SUBARRAY dynamic programming Google, Amazon, coding interview question Leetcode 152
Interview Problem (Dynamic Programming - Max Sum Subarray) Amazon, Google, Facebook
Maximum Sum Subarray in C++ | Amazon Coding Interview Question | Brute force #dsa #faang #amazon
Maximum Subarray Min-Product - Monotonic Increasing Stack - Leetcode 1856 - Python
Leetcode 53. Maximum Subarray | 1 of Amazon's Favourite Interview Questions | Python | FAANG Co...
LeetCode 53. Maximum Subarray - Python
Maximum Subarray - Amazon Coding Interview Question - Leetcode 53 - Python
Amazon Interview Question Leetcode 53. Maximum Subarray Python solution
Largest Subarray with Zero Sum | Amazon | MMT
Комментарии