filmov
tv
Longest Repeating Character Replacement - Leetcode 424 - Sliding Window (Python)
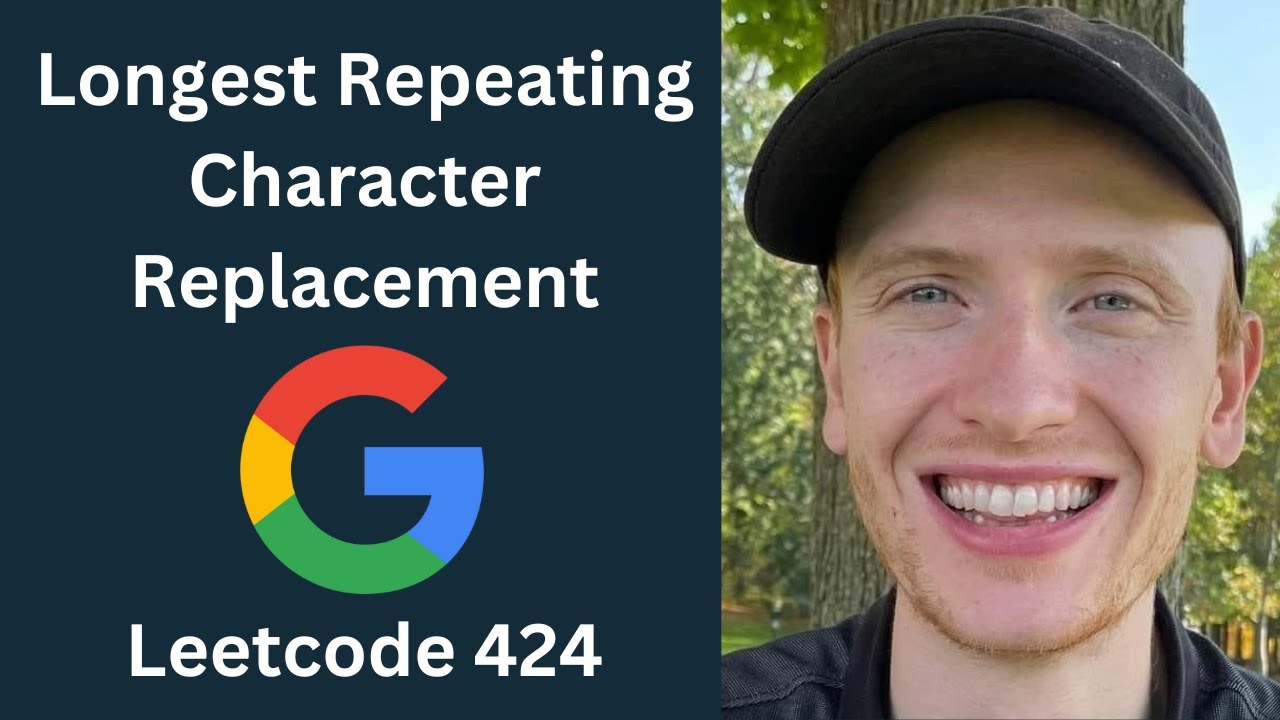
Показать описание
Please check my playlists for free DSA problem solutions:
Best Courses for Analytics:
---------------------------------------------------------------------------------------------------------
Best Courses for Programming:
---------------------------------------------------------------------------------------------------------
Best Courses for Machine Learning:
---------------------------------------------------------------------------------------------------------
Best Courses for Statistics:
---------------------------------------------------------------------------------------------------------
Best Courses for Big Data:
---------------------------------------------------------------------------------------------------------
More Courses:
---------------------------------------------------------------------------------------------------------
Full Disclosure:
Please note that I may earn a commission for purchases made at the above sites! I strongly believe in the material provided; I only recommend what I truly think is great. If you do choose to make purchases through these links; thank you for supporting the channel, it helps me make more free content like this!
Комментарии