filmov
tv
LeetCode Longest Repeating Character Replacement Solution Explained - Java
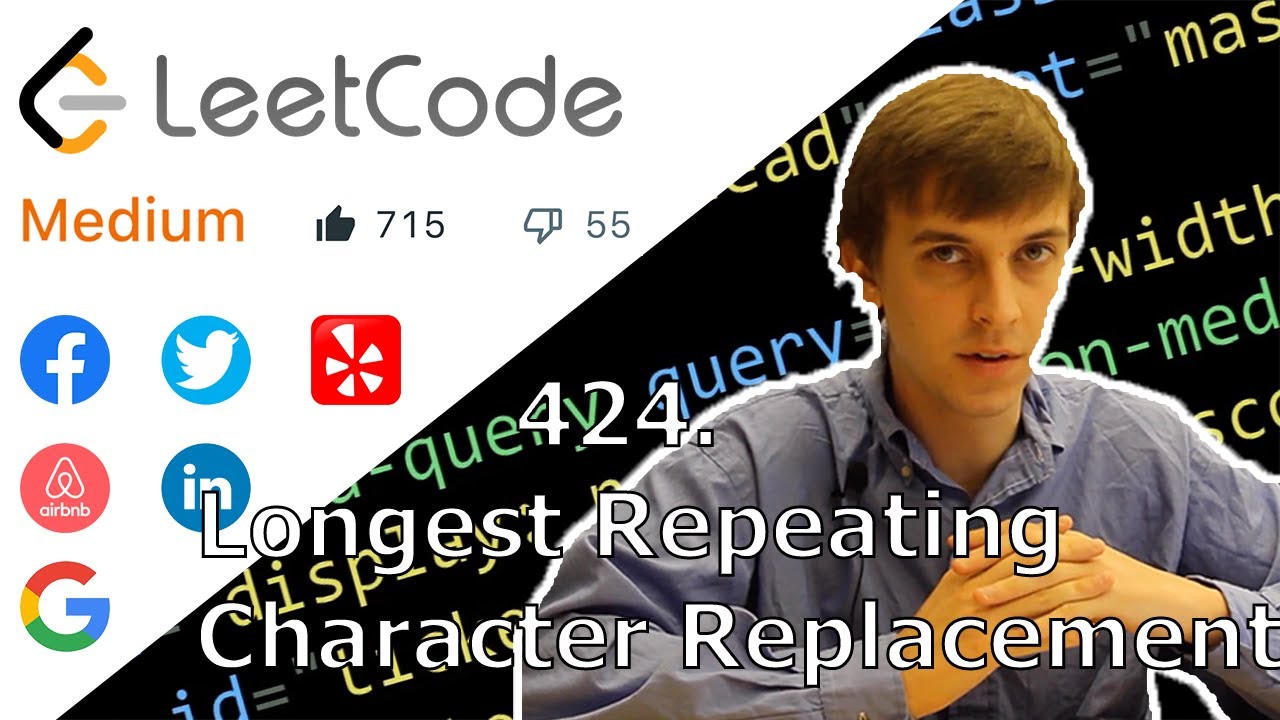
Показать описание
Preparing For Your Coding Interviews? Use These Resources
————————————————————
Other Social Media
----------------------------------------------
Show Support
------------------------------------------------------------------------------
#coding #programming #softwareengineering
Longest Repeating Character Replacement - Leetcode 424 - Python
Longest Repeating Character Replacement - Leetcode 424 - Sliding Window (Python)
LeetCode Longest Repeating Character Replacement Solution Explained - Java
Essential Coding Interview Question! | Longest Repeating Character Replacement - Leetcode 424
Longest Repeating Character Replacement (LeetCode 424) | Single iteration | Sliding Window
L8. Longest Repeating Character Replacement | 2 Pointers and Sliding Window Playlist
Longest Repeated Character Replacement - Amazon Interview Question
Longest Repeating Character Replacement - LeetCode 424 - Python #leetcode #slidingwindow #blind75
Leetcode Longest Repeating Character Replacement | Python
Longest Repeating Character Replacement - Sliding Window - Leetcode Medium - JAVA
424. Longest Repeating Character Replacement (Leetcode Medium)
[Java] Leetcode 424. Longest Repeating Character Replacement [Sliding Window #6]
Longest Repeating Character Replacement - LeetCode #424 with python programming
Отличное применение питоговского СЛОВАРЯ 📗 | LeetCode №038 - Longest Repeating Character Replacement...
Leetcode 424. Longest Repeating Character Replacement. Python (Sliding Window)
424. Longest Repeating Character Replacement | JavaScript | LeetCode 75 | Medium | Time Complexity
LeetCode | 424. Longest Repeating Character Replacement | Sliding Window | HashTable
Longest Repeating Character Replacement - LeetCode #424 - C++
LeetCode 424. Longest Repeating Character Replacement
Sliding Window Algorithm Explained Clearly | Longest Substring Without Repeating Characters Leetcode
LeetCode 424 - Longest Repeating Character Replacement
Longest Repeating Character Replacement - Leetcode 424 - Javascript
424. Longest Repeating Character Replacement- leetcode solution by ARAVIND A in java
8. Longest Repeating Character Replacement | Grokking the Coding Interview
Комментарии