filmov
tv
One Dimensional Array Practice (Array Operations)
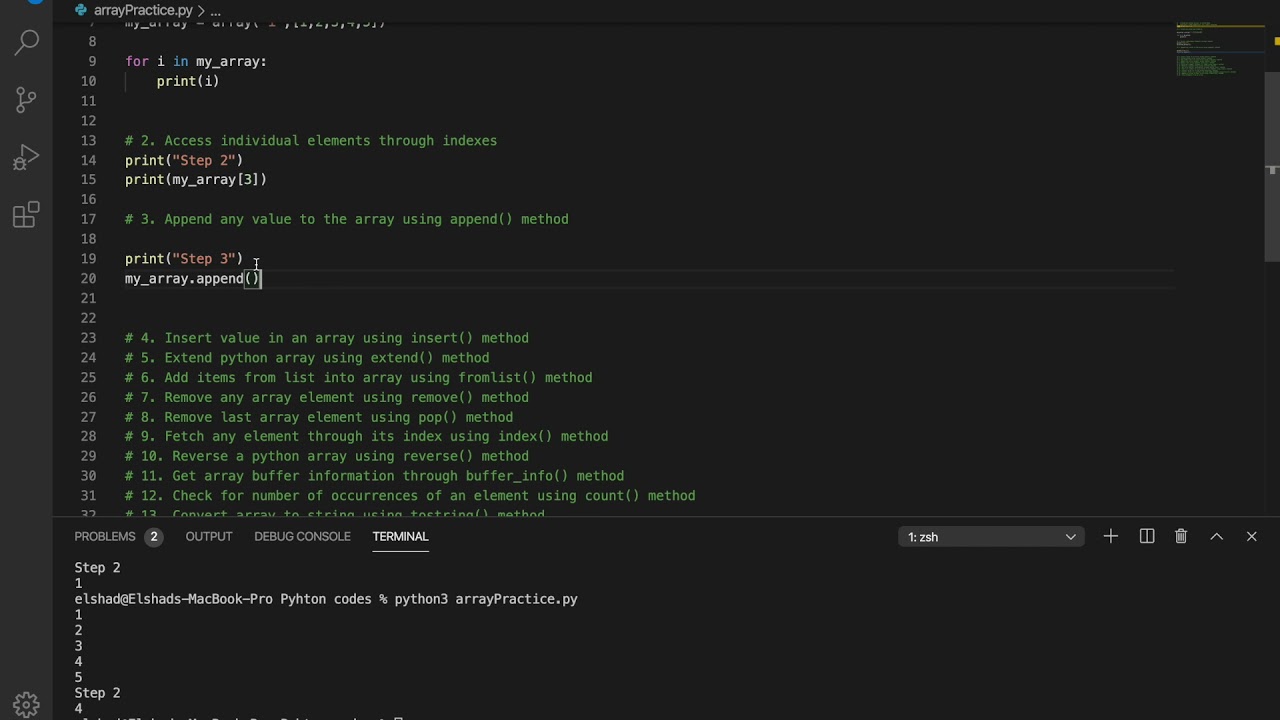
Показать описание
We're releasing a free preview (4 hours) of our 40+ hour The complete Data Structures and Algorithms course in Python on YouTube.
If you enjoyed this introduction to Data Structures and Algorithms in Python and want to continue learning, you can join the full The complete Data Structures and Algorithms course in Python from the AppMillers.
Get the best price by following the link below:
Playlist link:
Full Udemy course on Data Structure and Algorithms in Python covering the topics:
What are Data Structures?
What is an algorithm?
Why are Data Structures and Algorithms important?
Types of Data Structures
Types of Algorithms
Introduction to DS and Algorithms
Recursion
What is Recursion?
Why do we need recursion?
How Recursion works?
Recursive vs Iterative Solutions
When to use/avoid Recursion?
How to write Recursion in 3 steps?
How to find Fibonacci numbers using Recursion?
Cracking Recursion Interview Questions
Big O Notation
Analogy and Time Complexity
Big O, Big Theta and Big Omega
Time complexity examples
Space Complexity
Drop the Constants and the non dominant terms
Add vs Multiply
How to measure the codes using Big O?
How to find time complexity for Recursive calls?
How to measure Recursive Algorithms that make multiple calls?
Time Complexities
Top 10 Big O Interview Questions (Amazon, Facebook, Apple and Microsoft)
Arrays
What is an Array?
Types of Array
Arrays in Memory
Create an Array
Insertion Operation
Traversal Operation
Accessing an element of Array
Searching for an element in Array
Deleting an element from Array
Time and Space complexity of One Dimensional Array
One Dimensional Array Practice
Create Two Dimensional Array
Insertion - Two Dimensional Array
Accessing an element of Two Dimensional Array
Traversal - Two Dimensional Array
Searching for an element in Two Dimensional Array
Deletion - Two Dimensional Array
Time and Space complexity of Two Dimensional Array
When to use/avoid array
Python Lists
What is a List? How to create it?
Accessing/Traversing a list
Update/Insert a List
Slice/ from a List
Searching for an element in a List
List Operations/Functions
Lists and strings
Common List pitfalls and ways to avoid them
Lists vs Arrays
Time and Space Complexity of List
List Interview Questions
Cracking Array/List Interview Questions (Amazon, Facebook, Apple and Microsoft)
Question 1 - Missing Number
Question 2 - Pairs
Question 3 - Finding a number in an Array
Question 4 - Max product of two int
Question 5 - Is Unique
Question 6 - Permutation
Question 7 - Rotate Matrix
Dictionaries
What is a Dictionary?
Create a Dictionary
Dictionaries in memory
Insert /Update an element in a Dictionary
Traverse through a Dictionary
Search for an element in a Dictionary
Delete / Remove an element from a Dictionary
Dictionary Methods
Dictionary operations/ built in functions
Dictionary vs List
Time and Space Complexity of a Dictionary
Dictionary Interview Questions
Tuples
What is a Tuple? How to create it?
Tuples in Memory / Accessing an element of Tuple
Traversing a Tuple
Search for an element in Tuple
Tuple Operations/Functions
Tuple vs List
Time and Space complexity of Tuples
Tuple Questions
Linked List
What is a Linked List?
Linked List vs Arrays
Types of Linked List
Linked List in the Memory
Creation of Singly Linked List
Insertion in Singly Linked List in Memory
Insertion in Singly Linked List Algorithm
Insertion Method in Singly Linked List
Traversal of Singly Linked List
Search for a value in Single Linked List
Deletion of node from Singly Linked List
Deletion Method in Singly Linked List
Deletion of entire Singly Linked List
Time and Space Complexity of Singly Linked List
Creation of Circular Singly Linked List
Insertion in Circular Singly Linked List
Insertion Algorithm in Circular Singly Linked List
Insertion method in Circular Singly Linked List
Traversal of Circular Singly Linked List
Searching a node in Circular Singly Linked List
Deletion of a node from Circular Singly Linked List
Deletion Algorithm in Circular Singly Linked List
Method in Circular Singly Linked List
Deletion of entire Circular Singly Linked List
Time and Space Complexity of Circular Singly Linked List
Creation of Doubly Linked List
Insertion in Doubly Linked List
Insertion Algorithm in Doubly Linked List
Insertion Method in Doubly Linked List
Traversal of Doubly Linked List
Reverse Traversal of Doubly Linked List
Searching for a node in Doubly Linked List
Deletion of a node in Doubly Linked List
Deletion Algorithm in Doubly Linked List
Deletion Method in Doubly Linked List
Deletion of entire Doubly Linked List
Time and Space Complexity of Doubly Linked List
Creation of Circular Doubly Linked List
Insertion in Circular Doubly Linked List
Insertion Algorithm in Circular Doubly Linked List
Insertion Method in Circular Doubly Linked List
If you enjoyed this introduction to Data Structures and Algorithms in Python and want to continue learning, you can join the full The complete Data Structures and Algorithms course in Python from the AppMillers.
Get the best price by following the link below:
Playlist link:
Full Udemy course on Data Structure and Algorithms in Python covering the topics:
What are Data Structures?
What is an algorithm?
Why are Data Structures and Algorithms important?
Types of Data Structures
Types of Algorithms
Introduction to DS and Algorithms
Recursion
What is Recursion?
Why do we need recursion?
How Recursion works?
Recursive vs Iterative Solutions
When to use/avoid Recursion?
How to write Recursion in 3 steps?
How to find Fibonacci numbers using Recursion?
Cracking Recursion Interview Questions
Big O Notation
Analogy and Time Complexity
Big O, Big Theta and Big Omega
Time complexity examples
Space Complexity
Drop the Constants and the non dominant terms
Add vs Multiply
How to measure the codes using Big O?
How to find time complexity for Recursive calls?
How to measure Recursive Algorithms that make multiple calls?
Time Complexities
Top 10 Big O Interview Questions (Amazon, Facebook, Apple and Microsoft)
Arrays
What is an Array?
Types of Array
Arrays in Memory
Create an Array
Insertion Operation
Traversal Operation
Accessing an element of Array
Searching for an element in Array
Deleting an element from Array
Time and Space complexity of One Dimensional Array
One Dimensional Array Practice
Create Two Dimensional Array
Insertion - Two Dimensional Array
Accessing an element of Two Dimensional Array
Traversal - Two Dimensional Array
Searching for an element in Two Dimensional Array
Deletion - Two Dimensional Array
Time and Space complexity of Two Dimensional Array
When to use/avoid array
Python Lists
What is a List? How to create it?
Accessing/Traversing a list
Update/Insert a List
Slice/ from a List
Searching for an element in a List
List Operations/Functions
Lists and strings
Common List pitfalls and ways to avoid them
Lists vs Arrays
Time and Space Complexity of List
List Interview Questions
Cracking Array/List Interview Questions (Amazon, Facebook, Apple and Microsoft)
Question 1 - Missing Number
Question 2 - Pairs
Question 3 - Finding a number in an Array
Question 4 - Max product of two int
Question 5 - Is Unique
Question 6 - Permutation
Question 7 - Rotate Matrix
Dictionaries
What is a Dictionary?
Create a Dictionary
Dictionaries in memory
Insert /Update an element in a Dictionary
Traverse through a Dictionary
Search for an element in a Dictionary
Delete / Remove an element from a Dictionary
Dictionary Methods
Dictionary operations/ built in functions
Dictionary vs List
Time and Space Complexity of a Dictionary
Dictionary Interview Questions
Tuples
What is a Tuple? How to create it?
Tuples in Memory / Accessing an element of Tuple
Traversing a Tuple
Search for an element in Tuple
Tuple Operations/Functions
Tuple vs List
Time and Space complexity of Tuples
Tuple Questions
Linked List
What is a Linked List?
Linked List vs Arrays
Types of Linked List
Linked List in the Memory
Creation of Singly Linked List
Insertion in Singly Linked List in Memory
Insertion in Singly Linked List Algorithm
Insertion Method in Singly Linked List
Traversal of Singly Linked List
Search for a value in Single Linked List
Deletion of node from Singly Linked List
Deletion Method in Singly Linked List
Deletion of entire Singly Linked List
Time and Space Complexity of Singly Linked List
Creation of Circular Singly Linked List
Insertion in Circular Singly Linked List
Insertion Algorithm in Circular Singly Linked List
Insertion method in Circular Singly Linked List
Traversal of Circular Singly Linked List
Searching a node in Circular Singly Linked List
Deletion of a node from Circular Singly Linked List
Deletion Algorithm in Circular Singly Linked List
Method in Circular Singly Linked List
Deletion of entire Circular Singly Linked List
Time and Space Complexity of Circular Singly Linked List
Creation of Doubly Linked List
Insertion in Doubly Linked List
Insertion Algorithm in Doubly Linked List
Insertion Method in Doubly Linked List
Traversal of Doubly Linked List
Reverse Traversal of Doubly Linked List
Searching for a node in Doubly Linked List
Deletion of a node in Doubly Linked List
Deletion Algorithm in Doubly Linked List
Deletion Method in Doubly Linked List
Deletion of entire Doubly Linked List
Time and Space Complexity of Doubly Linked List
Creation of Circular Doubly Linked List
Insertion in Circular Doubly Linked List
Insertion Algorithm in Circular Doubly Linked List
Insertion Method in Circular Doubly Linked List
Комментарии