filmov
tv
Reading Binary Files and Looping Over Each Byte in Python
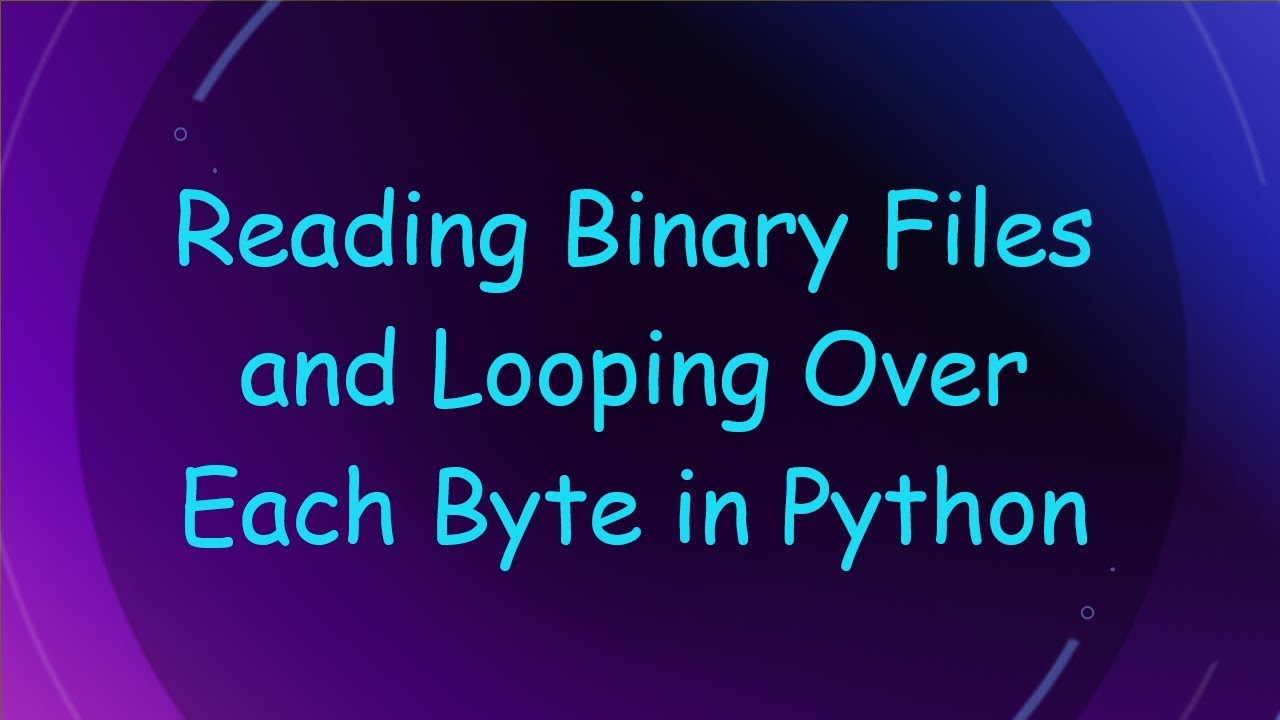
Показать описание
Summary: Learn how to read binary files and loop over each byte in Python. This guide provides step-by-step instructions to handle binary data efficiently in your Python applications.
---
Reading and processing binary files is a common task in many programming scenarios, including data analysis, file manipulation, and communication with hardware devices. In this guide, we will explore how to read a binary file and loop over each byte using Python.
Why Read Binary Files?
Binary files store data in a format that is not human-readable. Unlike text files, which store data as plain text, binary files can contain any type of data, such as images, audio, video, or any other format that requires efficient storage. Understanding how to read and process binary files allows you to work with a wider range of file types and perform low-level data manipulations.
Opening a Binary File
To read a binary file in Python, you need to open it in binary mode. This can be done using the built-in open function, specifying the mode as 'rb' (read binary).
Here is an example of how to open a binary file:
[[See Video to Reveal this Text or Code Snippet]]
Looping Over Each Byte
Once you have read the binary file, you can loop over each byte. In Python, the data read from a binary file is returned as a bytes object, which is essentially a sequence of bytes. You can iterate over this sequence using a simple for loop.
Here's how you can loop over each byte in the binary data:
[[See Video to Reveal this Text or Code Snippet]]
Working with Binary Data
In many cases, you might not just want to print each byte but perform some processing on the binary data. For example, you might need to decode the data, transform it, or extract specific information. Below are a few common operations you might perform on binary data.
Converting Bytes to Integers
If you need to work with numerical data stored in a binary file, you can convert bytes to integers. Python provides several ways to do this, but the simplest is using the built-in int function.
[[See Video to Reveal this Text or Code Snippet]]
Extracting Specific Bytes
You can extract specific bytes or ranges of bytes from the binary data using slicing.
[[See Video to Reveal this Text or Code Snippet]]
Decoding Binary Data
If the binary file contains encoded text data (e.g., UTF-8), you can decode it to a string.
[[See Video to Reveal this Text or Code Snippet]]
Example: Reading and Processing a Binary File
Let's put it all together with a complete example that reads a binary file, processes each byte, and performs some operations on the data.
[[See Video to Reveal this Text or Code Snippet]]
In this example, we read a binary file and perform a bitwise NOT operation on each byte. This is just a simple illustration; in practice, you might need to perform more complex operations depending on your specific requirements.
Conclusion
Reading and processing binary files in Python is a straightforward task that opens up many possibilities for working with different types of data. By understanding how to read binary files and loop over each byte, you can handle various file formats and perform low-level data manipulations effectively. Whether you are dealing with images, audio files, or custom binary formats, the techniques discussed in this post will help you get started.
---
Reading and processing binary files is a common task in many programming scenarios, including data analysis, file manipulation, and communication with hardware devices. In this guide, we will explore how to read a binary file and loop over each byte using Python.
Why Read Binary Files?
Binary files store data in a format that is not human-readable. Unlike text files, which store data as plain text, binary files can contain any type of data, such as images, audio, video, or any other format that requires efficient storage. Understanding how to read and process binary files allows you to work with a wider range of file types and perform low-level data manipulations.
Opening a Binary File
To read a binary file in Python, you need to open it in binary mode. This can be done using the built-in open function, specifying the mode as 'rb' (read binary).
Here is an example of how to open a binary file:
[[See Video to Reveal this Text or Code Snippet]]
Looping Over Each Byte
Once you have read the binary file, you can loop over each byte. In Python, the data read from a binary file is returned as a bytes object, which is essentially a sequence of bytes. You can iterate over this sequence using a simple for loop.
Here's how you can loop over each byte in the binary data:
[[See Video to Reveal this Text or Code Snippet]]
Working with Binary Data
In many cases, you might not just want to print each byte but perform some processing on the binary data. For example, you might need to decode the data, transform it, or extract specific information. Below are a few common operations you might perform on binary data.
Converting Bytes to Integers
If you need to work with numerical data stored in a binary file, you can convert bytes to integers. Python provides several ways to do this, but the simplest is using the built-in int function.
[[See Video to Reveal this Text or Code Snippet]]
Extracting Specific Bytes
You can extract specific bytes or ranges of bytes from the binary data using slicing.
[[See Video to Reveal this Text or Code Snippet]]
Decoding Binary Data
If the binary file contains encoded text data (e.g., UTF-8), you can decode it to a string.
[[See Video to Reveal this Text or Code Snippet]]
Example: Reading and Processing a Binary File
Let's put it all together with a complete example that reads a binary file, processes each byte, and performs some operations on the data.
[[See Video to Reveal this Text or Code Snippet]]
In this example, we read a binary file and perform a bitwise NOT operation on each byte. This is just a simple illustration; in practice, you might need to perform more complex operations depending on your specific requirements.
Conclusion
Reading and processing binary files in Python is a straightforward task that opens up many possibilities for working with different types of data. By understanding how to read binary files and loop over each byte, you can handle various file formats and perform low-level data manipulations effectively. Whether you are dealing with images, audio files, or custom binary formats, the techniques discussed in this post will help you get started.