filmov
tv
Python Tutorial: File Objects - Reading and Writing to Files
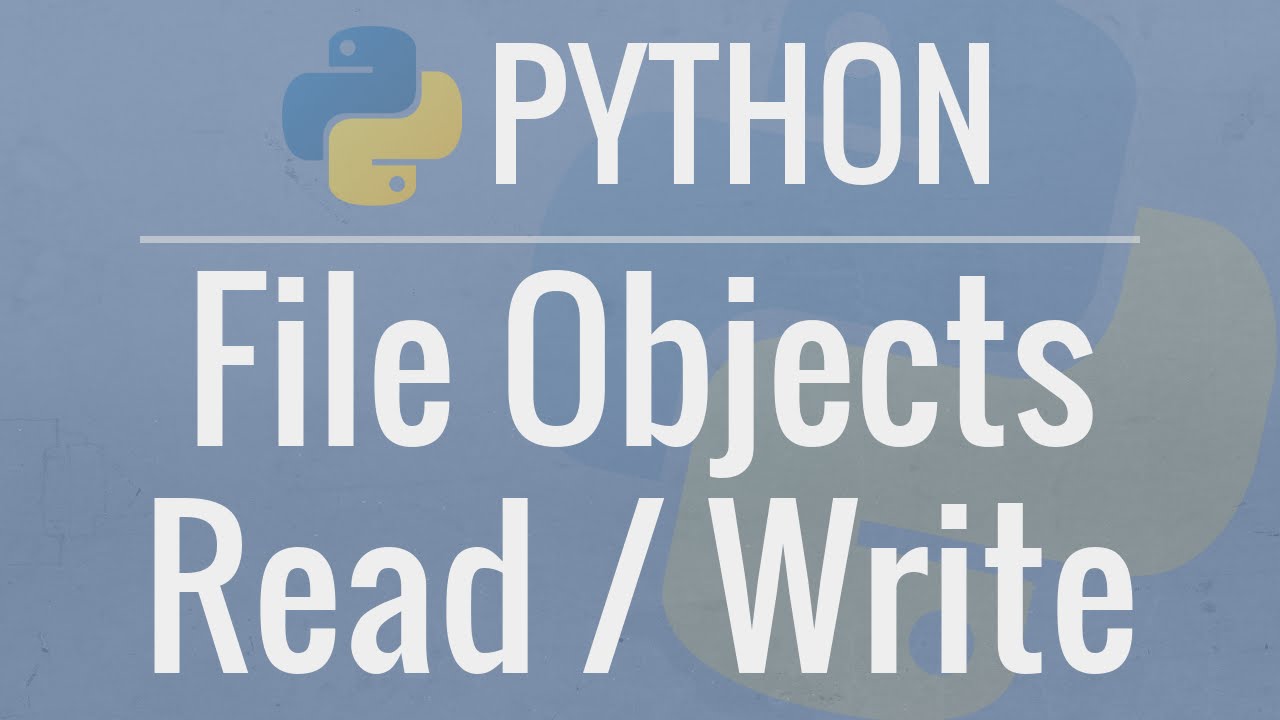
Показать описание
In this Python Tutorial, we will be learning how to read and write to files. You will likely come into contact with file objects at some point while using Python, so knowing how to read and write from them is extremely important. We will learn how to read and write from simple text files, open multiple files at once, and also how to copy image binary files. Let's get started.
The code from this video can be found at:
Read more about opening in binary mode here:
✅ Support My Channel Through Patreon:
✅ Become a Channel Member:
✅ One-Time Contribution Through PayPal:
✅ Cryptocurrency Donations:
Bitcoin Wallet - 3MPH8oY2EAgbLVy7RBMinwcBntggi7qeG3
Ethereum Wallet - 0x151649418616068fB46C3598083817101d3bCD33
Litecoin Wallet - MPvEBY5fxGkmPQgocfJbxP6EmTo5UUXMot
✅ Corey's Public Amazon Wishlist
✅ Equipment I Use and Books I Recommend:
▶️ You Can Find Me On:
#Python
The code from this video can be found at:
Read more about opening in binary mode here:
✅ Support My Channel Through Patreon:
✅ Become a Channel Member:
✅ One-Time Contribution Through PayPal:
✅ Cryptocurrency Donations:
Bitcoin Wallet - 3MPH8oY2EAgbLVy7RBMinwcBntggi7qeG3
Ethereum Wallet - 0x151649418616068fB46C3598083817101d3bCD33
Litecoin Wallet - MPvEBY5fxGkmPQgocfJbxP6EmTo5UUXMot
✅ Corey's Public Amazon Wishlist
✅ Equipment I Use and Books I Recommend:
▶️ You Can Find Me On:
#Python
Python Tutorial: File Objects - Reading and Writing to Files
File Handling In Python | Python File IO | Python Read & Write Files | Python Tutorial | Simplil...
#65 Python Tutorial for Beginners | File handling
Python read a file 🔍
Python write a file 📝
Python Tutorial - 13. Reading/Writing Files
Python Tutorial: Working with JSON Data using the json Module
Classes and Objects with Python - Part 1 (Python Tutorial #9)
PYTHON tutorials || Demo - 1 || by Mr. N. Vijay Sunder Sagar On 18-07-2024 @7PM IST
Text Files in Python || Python Tutorial || Learn Python Programming
Intermediate Python Tutorial: How to Use the __init__.py File
Python Tutorial for Beginners 9: Import Modules and Exploring The Standard Library
5 Tips To Organize Python Code
FILES (OPEN,CLOSE,ACCESS MODES) - PYTHON PROGRAMMING
Python Object Oriented Programming in 10 minutes 🐍
Python Tutorial: if __name__ == '__main__'
Python Requests Tutorial: Request Web Pages, Download Images, POST Data, Read JSON, and More
Reading Files | Python | Tutorial 28
Writing to Files | Python | Tutorial 29
Python Tutorial: OS Module - Use Underlying Operating System Functionality
Using Object/Classes in Other File with Python
Zip Function in Python
Python Tutorial for Beginners 41 - Create a Text File and Write in It Using Python
Importing Your Own Python Modules Properly
Комментарии