filmov
tv
Solving the IndexError: list index out of range in Python Salary Splitting Code
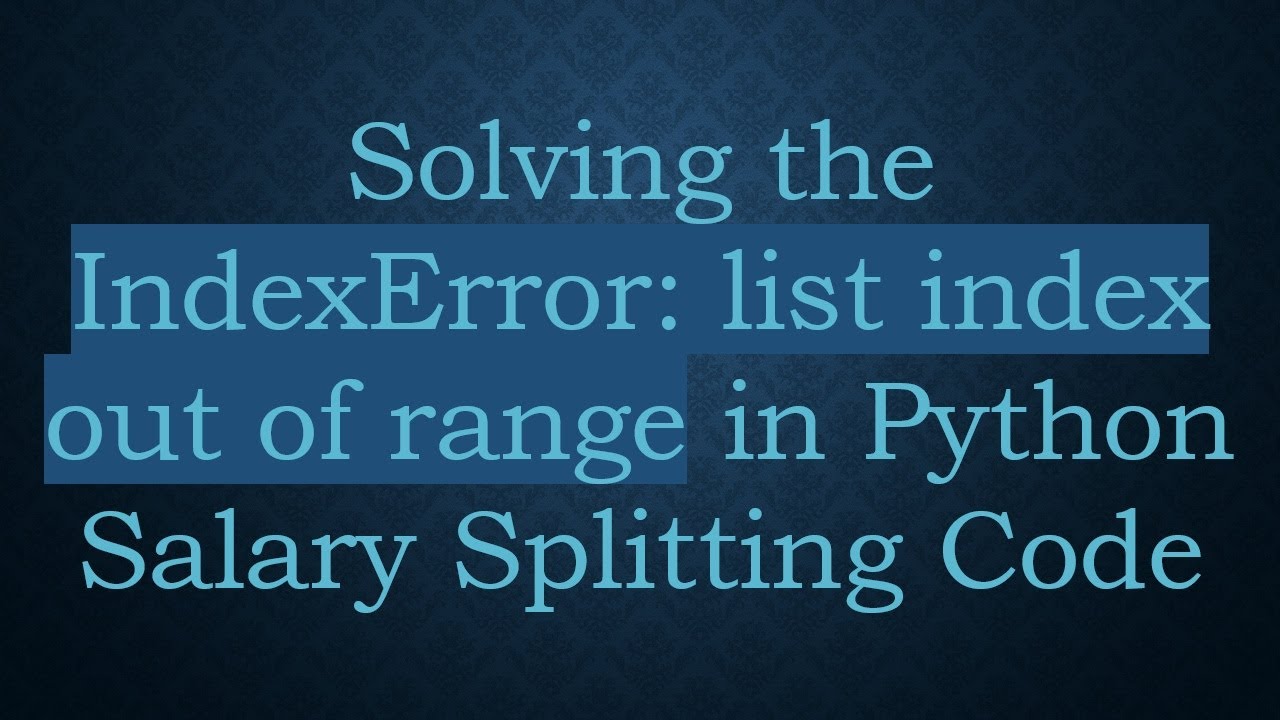
Показать описание
Learn how to tackle the common `IndexError` when trying to split salary strings in Python. Find solutions that handle varying formats in your data gracefully!
---
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the IndexError: List Index Out of Range in Salary Splitting Code
When working with data in Python, especially when dealing with strings, it's common to run into issues like the IndexError: list index out of range. This error can arise when you attempt to access an index in a list that doesn't exist. In this post, we’ll explore a specific case where this error occurs while splitting salary ranges and how to handle it efficiently.
The Problem
Imagine you have a DataFrame containing job salaries formatted as ranges, such as 80 - 100. You want to split these strings into separate minimum and maximum salaries. Here's a snippet of code that attempts to do this:
[[See Video to Reveal this Text or Code Snippet]]
While the code works fine for properly formatted entries, such as 80 - 100, it can throw an error for other formats, particularly when the string does not contain a hyphen -, resulting in an empty list when split. This leads to the dreaded IndexError when trying to access the second element of the list.
Identifying the Root Cause
The core issue here is that not all entries in your salary column follow the format you expect. For example, if some rows contain single values like 70, the split function will only create a list with one element, which cannot be accessed at index [1], thus causing the error.
To resolve this, you need a strategy that handles these inconsistencies gracefully.
Solution Approaches
Here are several approaches to handle and avoid the IndexError when splitting salary ranges in your DataFrame.
This method allows you to split the string into multiple columns smoothly and automatically handle cases where the expected format doesn't exist by filling in NaN for missing values:
[[See Video to Reveal this Text or Code Snippet]]
Example Output
After using the above code, your DataFrame might look like this:
[[See Video to Reveal this Text or Code Snippet]]
2. Filling Empty Values for Single Entries
If you want salaries that only have a single value to populate the max_salary column, you can use the following approach, which adds NaN where necessary:
[[See Video to Reveal this Text or Code Snippet]]
Example Output
This will yield the following DataFrame:
[[See Video to Reveal this Text or Code Snippet]]
3. Filling NaN Values Laterally
A potentially better approach in some cases is to forward-fill NaN values horizontally across your DataFrame, ensuring that each single salary fills in properly:
[[See Video to Reveal this Text or Code Snippet]]
Example Output
This will change your DataFrame to look like this:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By implementing these strategies, you can effectively manage the variability in your salary data and avoid the common IndexError when trying to split strings. Always remember to check for inconsistencies in your data to create robust code.
Utilizing NaN values can help you maintain a clean and organized DataFrame, ensuring that your analysis remains accurate and meaningful.
With these techniques under your belt, you'll navigate string manipulations in Python with confidence!
---
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the IndexError: List Index Out of Range in Salary Splitting Code
When working with data in Python, especially when dealing with strings, it's common to run into issues like the IndexError: list index out of range. This error can arise when you attempt to access an index in a list that doesn't exist. In this post, we’ll explore a specific case where this error occurs while splitting salary ranges and how to handle it efficiently.
The Problem
Imagine you have a DataFrame containing job salaries formatted as ranges, such as 80 - 100. You want to split these strings into separate minimum and maximum salaries. Here's a snippet of code that attempts to do this:
[[See Video to Reveal this Text or Code Snippet]]
While the code works fine for properly formatted entries, such as 80 - 100, it can throw an error for other formats, particularly when the string does not contain a hyphen -, resulting in an empty list when split. This leads to the dreaded IndexError when trying to access the second element of the list.
Identifying the Root Cause
The core issue here is that not all entries in your salary column follow the format you expect. For example, if some rows contain single values like 70, the split function will only create a list with one element, which cannot be accessed at index [1], thus causing the error.
To resolve this, you need a strategy that handles these inconsistencies gracefully.
Solution Approaches
Here are several approaches to handle and avoid the IndexError when splitting salary ranges in your DataFrame.
This method allows you to split the string into multiple columns smoothly and automatically handle cases where the expected format doesn't exist by filling in NaN for missing values:
[[See Video to Reveal this Text or Code Snippet]]
Example Output
After using the above code, your DataFrame might look like this:
[[See Video to Reveal this Text or Code Snippet]]
2. Filling Empty Values for Single Entries
If you want salaries that only have a single value to populate the max_salary column, you can use the following approach, which adds NaN where necessary:
[[See Video to Reveal this Text or Code Snippet]]
Example Output
This will yield the following DataFrame:
[[See Video to Reveal this Text or Code Snippet]]
3. Filling NaN Values Laterally
A potentially better approach in some cases is to forward-fill NaN values horizontally across your DataFrame, ensuring that each single salary fills in properly:
[[See Video to Reveal this Text or Code Snippet]]
Example Output
This will change your DataFrame to look like this:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By implementing these strategies, you can effectively manage the variability in your salary data and avoid the common IndexError when trying to split strings. Always remember to check for inconsistencies in your data to create robust code.
Utilizing NaN values can help you maintain a clean and organized DataFrame, ensuring that your analysis remains accurate and meaningful.
With these techniques under your belt, you'll navigate string manipulations in Python with confidence!