filmov
tv
How to Fix 'IndexError: List Index Out of Range' in Python Profiler Code
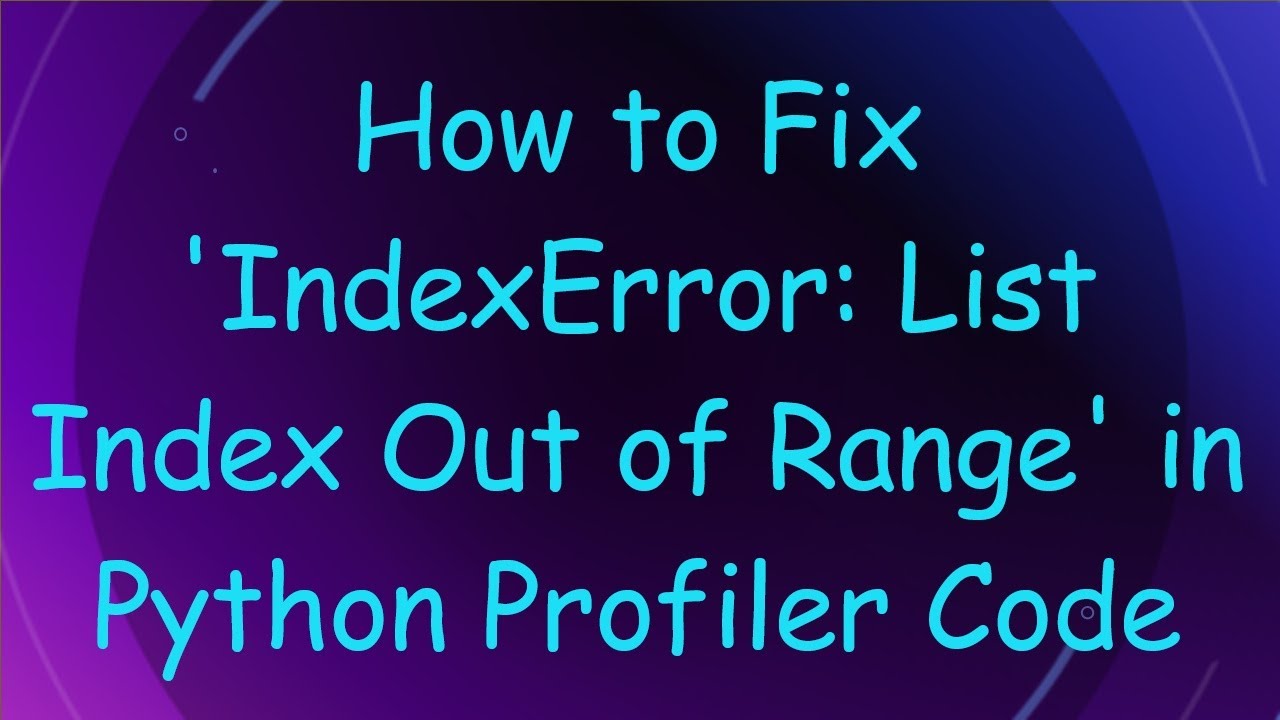
Показать описание
Learn how to resolve the 'IndexError: List Index Out of Range' error while profiling Python code, specifically in Python 2.7.
---
How to Fix 'IndexError: List Index Out of Range' in Python Profiler Code
Errors and exceptions are common when coding, especially in Python. One of the frequent ones you may encounter is the IndexError: List Index Out of Range. This error is particularly prevalent when working with the Python profiler, and it can occur in any version, including Python 2.7.
Understanding the Error
The IndexError occurs when you try to access an index that does not exist in a list. This might happen due to various reasons, such as:
Accessing an element at a negative index
Trying to loop beyond the length of the list
Miscalculations in index offsets
Here's a simple example to illustrate this error:
[[See Video to Reveal this Text or Code Snippet]]
Causes in Python Profiler Code
When you profile your code to measure performance, the profiler collects function calls and execution times. For Python 2.7, the profiler module is useful but can produce an IndexError if:
The profiling code has logic errors that affect list indexing
The profiler's internal data structures get mismanaged, leading to invalid index access
Step-by-Step Guide to Fix the Error
Check Indexes: The first thing you should do is to check the indexes that are producing the error.
Ensure your loops are not going out of range.
Debug and print index values before accessing the list.
Use len() Function: Always utilize the len() function to safeguard against out-of-range errors.
[[See Video to Reveal this Text or Code Snippet]]
Profiler Enhancements: When such an error occurs in profiling, you might need to dig into the specifics of the profiler code.
Add sanity checks before accessing lists inside your profiling functions.
Ensure that any manually written instrumentation logic doesn’t mismanage index offsets.
[[See Video to Reveal this Text or Code Snippet]]
Exception Handling: As a temporary measure or in cases where you cannot directly control the indexing, apply exception handling.
[[See Video to Reveal this Text or Code Snippet]]
Check Data Sources: Sometimes, dynamically generated lists or profiler output may cause discrepancies. Verify the integrity of data sources and collections manipulated during profiling.
Update or Patch: If using a specific profiling tool or library, check for updates or patches that address known IndexError issues.
By understanding the core reasons behind the IndexError and applying diligent indexing practices, you can significantly minimize such errors in your Python profiler code.
Conclusion
Profiling in Python offers immense insights into code performance, but it can sometimes bring along challenges like the IndexError: List Index Out of Range. By following the steps shared above, you can troubleshoot and fix these errors effectively, ensuring robust and error-free profiling in your Python projects.
---
How to Fix 'IndexError: List Index Out of Range' in Python Profiler Code
Errors and exceptions are common when coding, especially in Python. One of the frequent ones you may encounter is the IndexError: List Index Out of Range. This error is particularly prevalent when working with the Python profiler, and it can occur in any version, including Python 2.7.
Understanding the Error
The IndexError occurs when you try to access an index that does not exist in a list. This might happen due to various reasons, such as:
Accessing an element at a negative index
Trying to loop beyond the length of the list
Miscalculations in index offsets
Here's a simple example to illustrate this error:
[[See Video to Reveal this Text or Code Snippet]]
Causes in Python Profiler Code
When you profile your code to measure performance, the profiler collects function calls and execution times. For Python 2.7, the profiler module is useful but can produce an IndexError if:
The profiling code has logic errors that affect list indexing
The profiler's internal data structures get mismanaged, leading to invalid index access
Step-by-Step Guide to Fix the Error
Check Indexes: The first thing you should do is to check the indexes that are producing the error.
Ensure your loops are not going out of range.
Debug and print index values before accessing the list.
Use len() Function: Always utilize the len() function to safeguard against out-of-range errors.
[[See Video to Reveal this Text or Code Snippet]]
Profiler Enhancements: When such an error occurs in profiling, you might need to dig into the specifics of the profiler code.
Add sanity checks before accessing lists inside your profiling functions.
Ensure that any manually written instrumentation logic doesn’t mismanage index offsets.
[[See Video to Reveal this Text or Code Snippet]]
Exception Handling: As a temporary measure or in cases where you cannot directly control the indexing, apply exception handling.
[[See Video to Reveal this Text or Code Snippet]]
Check Data Sources: Sometimes, dynamically generated lists or profiler output may cause discrepancies. Verify the integrity of data sources and collections manipulated during profiling.
Update or Patch: If using a specific profiling tool or library, check for updates or patches that address known IndexError issues.
By understanding the core reasons behind the IndexError and applying diligent indexing practices, you can significantly minimize such errors in your Python profiler code.
Conclusion
Profiling in Python offers immense insights into code performance, but it can sometimes bring along challenges like the IndexError: List Index Out of Range. By following the steps shared above, you can troubleshoot and fix these errors effectively, ensuring robust and error-free profiling in your Python projects.