filmov
tv
Understanding the Differences Between Method Receivers and Function Parameters in Golang
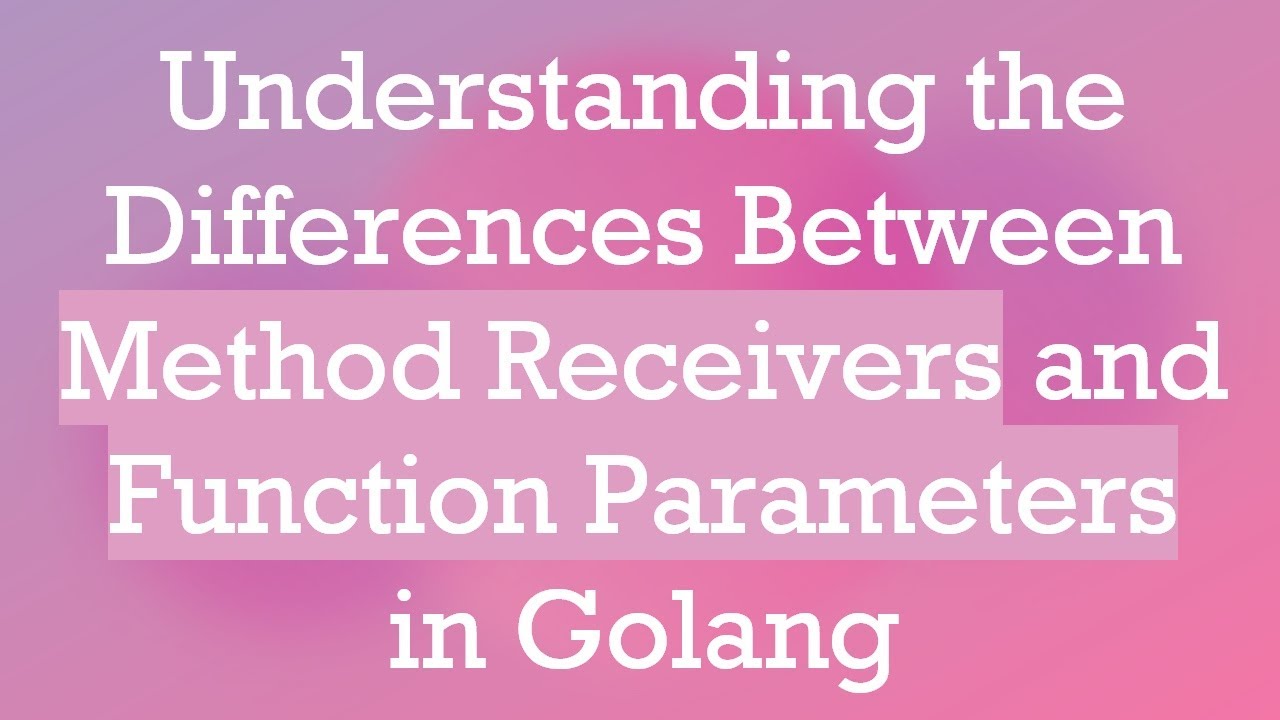
Показать описание
Summary: Uncover the key differences between method receivers and function parameters in Golang. Learn how each is used and best practices for effective Go programming.
---
Understanding the Differences Between Method Receivers and Function Parameters in Golang
Golang, or Go, is a statically typed, compiled language designed at Google that's known for its simplicity and efficiency. When you dive into Go, understanding how to structure your code using method receivers and function parameters is crucial. This guide will explore the differences between these two fundamental concepts to help you write cleaner and more idiomatic Go code.
Syntax of a Function in Go
Before diving into the differences, let’s review the syntax of a function in Go:
[[See Video to Reveal this Text or Code Snippet]]
In this example, add is a function that takes two parameters, a and b, both of type int, and returns their sum.
What are Method Receivers?
A method receiver is a special kind of parameter that allows a function to be associated with a specific type—either a struct or another custom type. Methods are akin to functions, but they include a receiver argument in their definition.
Syntax Example of a Method in Go
[[See Video to Reveal this Text or Code Snippet]]
Here, Rectangle is a struct type, and Area is a method with a receiver of type Rectangle. This means you can call Area directly on instances of Rectangle.
Key Differences
Association with Types
Function Parameters: Standalone functions are not tied to any specific type and can be called from anywhere in the code, provided the correct arguments are passed.
Method Receivers: Methods are intrinsically linked to the type of their receiver. This association allows methods to encapsulate behavior specific to the type.
Semantic Clarity
Function Parameters: Functions are generally used to execute stateless operations. They operate on supplied arguments and typically don't alter any state outside their scope.
Method Receivers: Methods are used to define behavior and often manipulate the state of their receiver type. This provides a clearer, object-oriented way to manage type-specific behavior.
Namespace and Code Organization
Function Parameters: Namespaces for functions are global. This means that function names must be unique within a package to avoid conflicts.
Method Receivers: Methods are scoped to the type of their receiver. This allows you to have methods with the same name as long as they are associated with different types, aiding in better code organization.
Mutability
Function Parameters: By default, arguments passed to functions are passed by value unless a pointer is used.
Method Receivers: Methods can have either value receivers or pointer receivers. Value receivers get a copy of the type, while pointer receivers receive a reference, allowing the method to modify the receiver’s fields directly.
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Understanding the differences between method receivers and function parameters is essential in Go programming. While both serve to modularize and organize your code, they have distinct use-cases and advantages. Method receivers are particularly useful for creating type-specific behavior and maintaining state, whereas function parameters are ideal for stateless operations. Armed with this knowledge, you'll be better equipped to write clean, idiomatic Go code.
---
Understanding the Differences Between Method Receivers and Function Parameters in Golang
Golang, or Go, is a statically typed, compiled language designed at Google that's known for its simplicity and efficiency. When you dive into Go, understanding how to structure your code using method receivers and function parameters is crucial. This guide will explore the differences between these two fundamental concepts to help you write cleaner and more idiomatic Go code.
Syntax of a Function in Go
Before diving into the differences, let’s review the syntax of a function in Go:
[[See Video to Reveal this Text or Code Snippet]]
In this example, add is a function that takes two parameters, a and b, both of type int, and returns their sum.
What are Method Receivers?
A method receiver is a special kind of parameter that allows a function to be associated with a specific type—either a struct or another custom type. Methods are akin to functions, but they include a receiver argument in their definition.
Syntax Example of a Method in Go
[[See Video to Reveal this Text or Code Snippet]]
Here, Rectangle is a struct type, and Area is a method with a receiver of type Rectangle. This means you can call Area directly on instances of Rectangle.
Key Differences
Association with Types
Function Parameters: Standalone functions are not tied to any specific type and can be called from anywhere in the code, provided the correct arguments are passed.
Method Receivers: Methods are intrinsically linked to the type of their receiver. This association allows methods to encapsulate behavior specific to the type.
Semantic Clarity
Function Parameters: Functions are generally used to execute stateless operations. They operate on supplied arguments and typically don't alter any state outside their scope.
Method Receivers: Methods are used to define behavior and often manipulate the state of their receiver type. This provides a clearer, object-oriented way to manage type-specific behavior.
Namespace and Code Organization
Function Parameters: Namespaces for functions are global. This means that function names must be unique within a package to avoid conflicts.
Method Receivers: Methods are scoped to the type of their receiver. This allows you to have methods with the same name as long as they are associated with different types, aiding in better code organization.
Mutability
Function Parameters: By default, arguments passed to functions are passed by value unless a pointer is used.
Method Receivers: Methods can have either value receivers or pointer receivers. Value receivers get a copy of the type, while pointer receivers receive a reference, allowing the method to modify the receiver’s fields directly.
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Understanding the differences between method receivers and function parameters is essential in Go programming. While both serve to modularize and organize your code, they have distinct use-cases and advantages. Method receivers are particularly useful for creating type-specific behavior and maintaining state, whereas function parameters are ideal for stateless operations. Armed with this knowledge, you'll be better equipped to write clean, idiomatic Go code.