filmov
tv
Creating a Generic Wait Function in Python to Check User Status
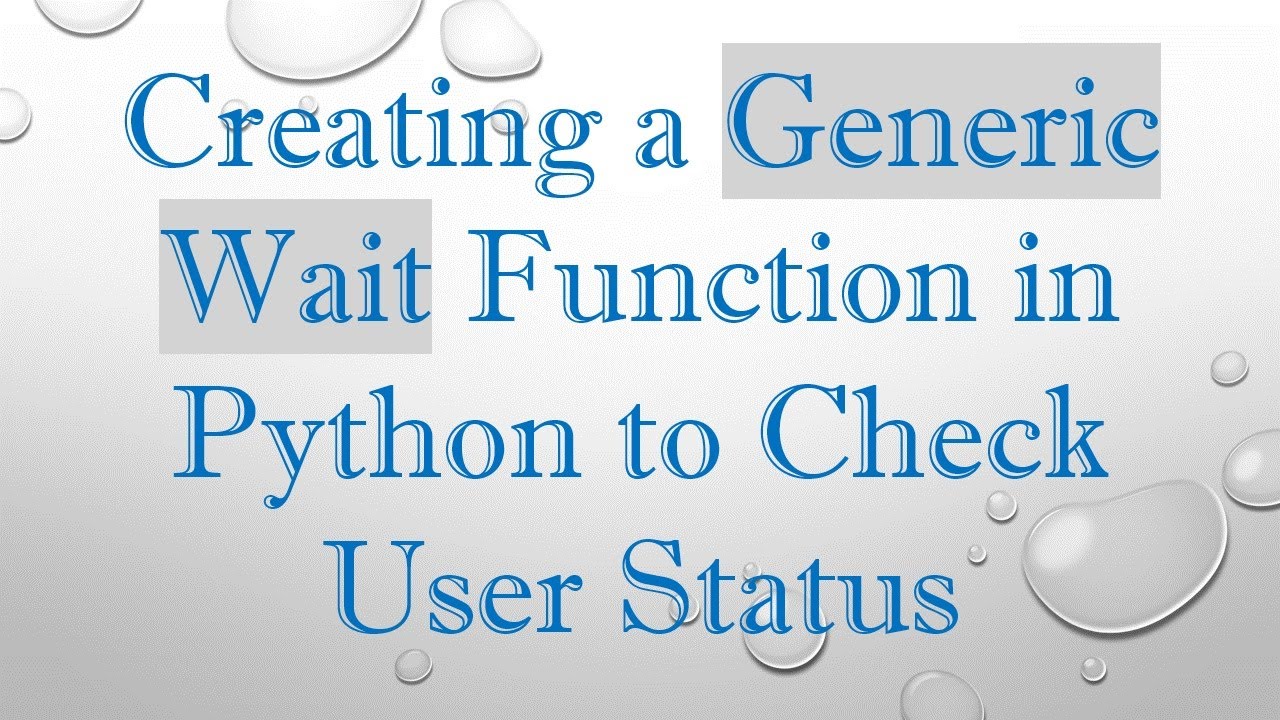
Показать описание
Learn how to create a flexible, generic function in Python that waits for a specific condition by accepting another function as a parameter.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: python - Generic function that accept another function as parameter and wait until condition
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Creating a Generic Wait Function in Python to Check User Status
In the world of programming, efficiency is key. Sometimes, however, we find ourselves needing to wait for a specific condition to be met before proceeding further in our execution flow. For example, if you are developing a system that requires checking a user’s status in a database, you might need to wait until they are connected. Let's learn how to implement a flexible solution to tackle this problem by creating a generic wait function in Python.
The Problem
Imagine you have a function called get_status which retrieves the current status of a user from a database. You want to ensure that you can continuously check this status until it reaches a specific value, such as connected. This could take some time, and you want to set a timeout to avoid waiting indefinitely.
Here’s a sample of your current implementation:
[[See Video to Reveal this Text or Code Snippet]]
The usage would look like this:
[[See Video to Reveal this Text or Code Snippet]]
But what if you have multiple functions you want to verify? How can you create a more generic solution that handles different types of status checks without writing redundant code? This brings us to our solution.
The Solution: A Generic Wait Function
To create a more reusable function, we will design a generic_wait function that accepts another function as a parameter alongside the necessary timeout. This approach will allow us to pass any function along with its parameters, making our code neater and more versatile.
Here’s how to implement this generic wait function:
[[See Video to Reveal this Text or Code Snippet]]
Usage Example
You can now easily use this in your code. Assuming you already have the get_status function defined, the usage would look like this:
[[See Video to Reveal this Text or Code Snippet]]
This way, you can replace get_status with any other function that checks different conditions and still maintain the same structure.
How It Works
Parameters: The generic_wait function takes a timeout, the function to check, and any additional arguments required by that function.
Looping with Condition: It runs a loop, continuously calling the function until either the result meets the expected condition (e.g., connected), or the timeout is reached.
Execution Control: If the timeout is reached without meeting the condition, an exception is raised, which provides an informative message.
Conclusion
Creating a generic wait function in Python allows you to maintain cleaner code by avoiding repetitive status-checking methods. With this flexible solution, you can handle various scenarios while setting consistent timeout behavior. By implementing the generic_wait function, you can enhance your program's efficiency and reliability for user status checks or similar use cases.
Feel free to adapt and expand upon this generic function to suit your unique requirements in the vast landscape of programming challenges!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: python - Generic function that accept another function as parameter and wait until condition
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Creating a Generic Wait Function in Python to Check User Status
In the world of programming, efficiency is key. Sometimes, however, we find ourselves needing to wait for a specific condition to be met before proceeding further in our execution flow. For example, if you are developing a system that requires checking a user’s status in a database, you might need to wait until they are connected. Let's learn how to implement a flexible solution to tackle this problem by creating a generic wait function in Python.
The Problem
Imagine you have a function called get_status which retrieves the current status of a user from a database. You want to ensure that you can continuously check this status until it reaches a specific value, such as connected. This could take some time, and you want to set a timeout to avoid waiting indefinitely.
Here’s a sample of your current implementation:
[[See Video to Reveal this Text or Code Snippet]]
The usage would look like this:
[[See Video to Reveal this Text or Code Snippet]]
But what if you have multiple functions you want to verify? How can you create a more generic solution that handles different types of status checks without writing redundant code? This brings us to our solution.
The Solution: A Generic Wait Function
To create a more reusable function, we will design a generic_wait function that accepts another function as a parameter alongside the necessary timeout. This approach will allow us to pass any function along with its parameters, making our code neater and more versatile.
Here’s how to implement this generic wait function:
[[See Video to Reveal this Text or Code Snippet]]
Usage Example
You can now easily use this in your code. Assuming you already have the get_status function defined, the usage would look like this:
[[See Video to Reveal this Text or Code Snippet]]
This way, you can replace get_status with any other function that checks different conditions and still maintain the same structure.
How It Works
Parameters: The generic_wait function takes a timeout, the function to check, and any additional arguments required by that function.
Looping with Condition: It runs a loop, continuously calling the function until either the result meets the expected condition (e.g., connected), or the timeout is reached.
Execution Control: If the timeout is reached without meeting the condition, an exception is raised, which provides an informative message.
Conclusion
Creating a generic wait function in Python allows you to maintain cleaner code by avoiding repetitive status-checking methods. With this flexible solution, you can handle various scenarios while setting consistent timeout behavior. By implementing the generic_wait function, you can enhance your program's efficiency and reliability for user status checks or similar use cases.
Feel free to adapt and expand upon this generic function to suit your unique requirements in the vast landscape of programming challenges!