filmov
tv
Conversion of Unicode string to ASCII in python 2 7
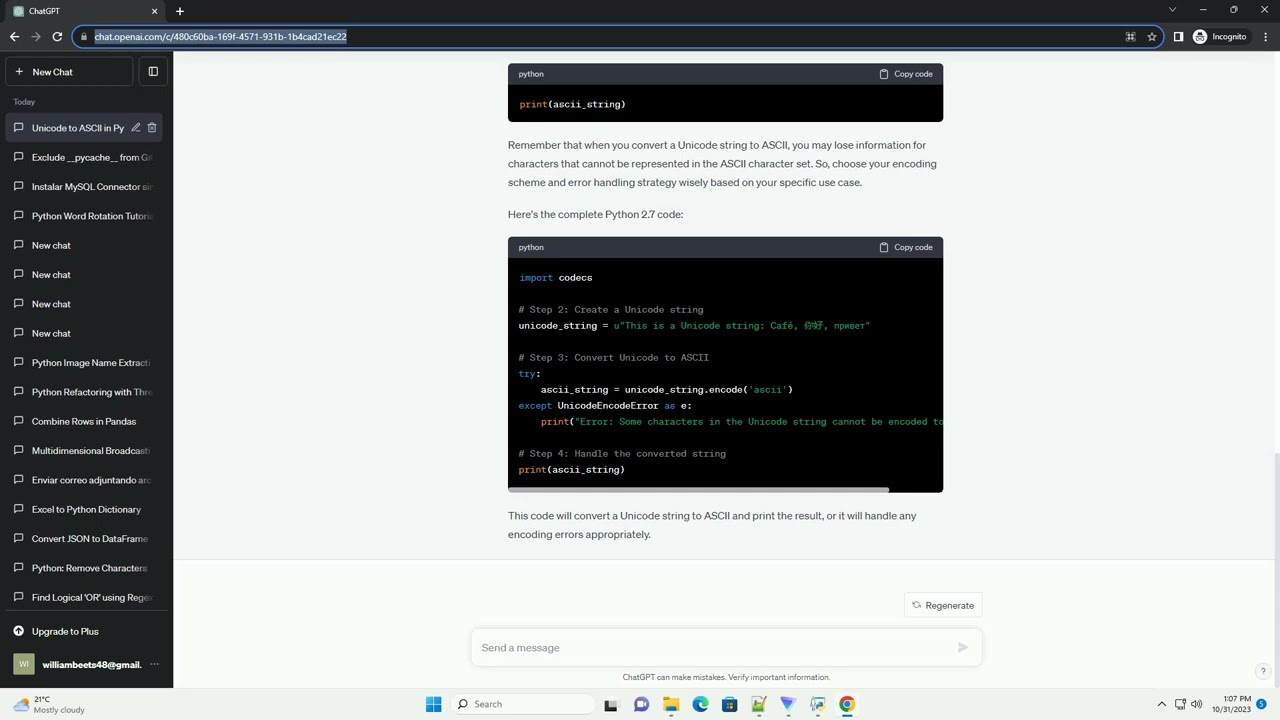
Показать описание
Converting a Unicode string to ASCII in Python 2.7 can be a useful task, especially if you need to work with legacy systems that only support ASCII characters. Unicode is a superset of ASCII, so not all Unicode characters can be directly converted to ASCII. You might lose some characters during this conversion since ASCII only supports a limited character set. Here's a step-by-step tutorial on how to do this in Python 2.7, along with code examples.
Step 1: Import the necessary module
In Python, you can use the encode method to convert a Unicode string to an ASCII-encoded string. You need to import the codecs module for this purpose.
Step 2: Create a Unicode string
You need a Unicode string that you want to convert to ASCII. Let's create a sample Unicode string for this tutorial:
Step 3: Convert Unicode to ASCII
Use the encode method to convert the Unicode string to ASCII. You can specify a specific encoding scheme to handle non-ASCII characters. The most common encoding scheme to convert Unicode to ASCII is "ascii" which will replace non-ASCII characters with a suitable replacement character or raise an exception.
Here's how to use the "ascii" encoding scheme:
In the code above, if any character in unicode_string can't be represented in ASCII, it will raise a UnicodeEncodeError. You can handle this error as needed for your specific use case.
If you want to remove non-ASCII characters instead of raising an error, you can use the "ignore" option:
This will simply remove any non-ASCII characters from the string.
Step 4: Handle the converted string
Now that you have your ASCII-encoded string, you can work with it as needed. You can print it, save it to a file, or use it in any way that suits your project.
Here's how to print the converted ASCII string:
Remember that when you convert a Unicode string to ASCII, you may lose information for characters that cannot be represented in the ASCII character set. So, choose your encoding scheme and error handling strategy wisely based on your specific use case.
Here's the complete Python 2.7 code:
This code will convert a Unicode string to ASCII and print the result, or it will handle any encoding errors appropriately.
ChatGPT
Step 1: Import the necessary module
In Python, you can use the encode method to convert a Unicode string to an ASCII-encoded string. You need to import the codecs module for this purpose.
Step 2: Create a Unicode string
You need a Unicode string that you want to convert to ASCII. Let's create a sample Unicode string for this tutorial:
Step 3: Convert Unicode to ASCII
Use the encode method to convert the Unicode string to ASCII. You can specify a specific encoding scheme to handle non-ASCII characters. The most common encoding scheme to convert Unicode to ASCII is "ascii" which will replace non-ASCII characters with a suitable replacement character or raise an exception.
Here's how to use the "ascii" encoding scheme:
In the code above, if any character in unicode_string can't be represented in ASCII, it will raise a UnicodeEncodeError. You can handle this error as needed for your specific use case.
If you want to remove non-ASCII characters instead of raising an error, you can use the "ignore" option:
This will simply remove any non-ASCII characters from the string.
Step 4: Handle the converted string
Now that you have your ASCII-encoded string, you can work with it as needed. You can print it, save it to a file, or use it in any way that suits your project.
Here's how to print the converted ASCII string:
Remember that when you convert a Unicode string to ASCII, you may lose information for characters that cannot be represented in the ASCII character set. So, choose your encoding scheme and error handling strategy wisely based on your specific use case.
Here's the complete Python 2.7 code:
This code will convert a Unicode string to ASCII and print the result, or it will handle any encoding errors appropriately.
ChatGPT