filmov
tv
Print Binary Representation of Decimal Number in C/C++
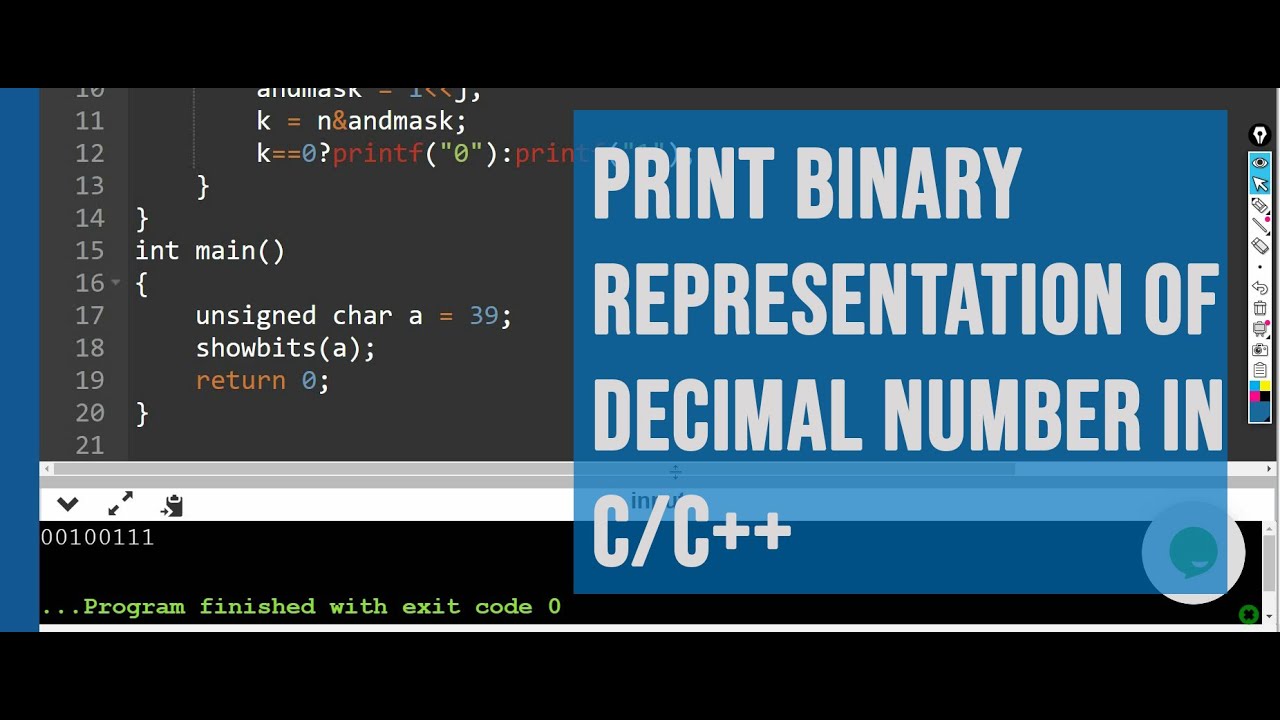
Показать описание
in this video we'll see
how
show bits function work so we're going
to print the binary representation of a
decimal number okay
so the decimal number here is 39 so if i
run the program
it runs the binary representation of
this
of this decimal number which is 39 right
this is a binary representation of 39
which has been
printed uh using this function show bits
all right so so normally when we want to
convert
a decimal number to binary
right so i will take 39 and i'll divide
it by two
and uh so
so i'll get 19 and the remainder will be
one
and then i'll again divide it by two so
two into nine is
eighteen which will throw a remainder
one
and then two into four is eight which
will again throw a remainder one
and then again i'll divide 2 into 2 is 4
and the remainder is 0. and then 2 into
1 is demeter is again 0.
okay so 1 triple 1 double 0 and then
triple 1 this is
binary okay and 39 is
yeah so so this is the binary
representation of
39 right so we can we can we can
implement this
in c but a more efficient way of
of achieving this uh of of achieving
this will be
using and mask right so what do we do
here
is we start a loop so you guys
so you can see the representation here
is eight eight bits
right so we'll start a loop from i is
equals to seven
okay and we'll take it
all the way to zero and then
uh sorry it's not less than it's greater
than equal to zero and then i minus
minus for each
um so it will it will decrement until it
reaches zero right and
and then with and then with each and
then with each iteration i'll store this
i
and then i'll create an and mask which
is
which is i'll shift one by
j bits so for the first time
so so let's say at first iteration
j is equals to seven okay uh so if i
shift one by seven bits it's one and
then
seven seven zeros all right so let's say
so one of the so one of the use of and
mask
is to check whether a bit is true or
false
right suppose i pass a bit
39 right where for which the
binary code is for which the binary code
is zero zero one zero zero
triple one zero zero one zero zero
triple one
okay that's the binary code for for 39
right
so if i um that's the binary code for
that's the binary code for 39 right
so so if i want to check that the
seventh bit
is zero or not i will simply
multiply this so let this number
so let this decimal number be n
and i'll simply simply perform a bitwise
and with and mask
so so if seventh bit is on
that means if seventh bit is one right
it will
return one or if the seventh bit is zero
it will return zero
right so zero zero one
double zero and then triple one all
right
so if i take bitwise and of
of both these uh sequences i'll get
zero right so zero for all the
so zero if i take end of both these
numbers
because the bit was actually zero right
now let's say now let's say we want to
check this
this one this is the fifth fifth bit
this is the seven bit six
with fifth bit right so if i want to
check for fifth bit so here it will be
zero and seven six
five right so rest rest all of them will
turn
zero and this one will be and
this one will be one right so
this value is something but it's not
zero it's a non-zero value
right so when it returns a non-zero
value
when we take a bitwise and of n
with uh with and mask
if it returns a non-zero value
that means this bit was on that means
the fifth bit was
on that means the fifth bit was one
right so that's exactly what we'll check
check here
if k equals to zero it should
print zero right
else it should print one that means if
it's a non-zero value
it should print one okay so
that's that's exactly what we have
implemented
implemented here in show bits right
uh i have taken the i'll pass the
unsigned
i'll pass the unsigned character inside
show bits
right so here so n will be the so n will
be
the decimal number and then the loop
will
start from seven to zero because zero to
seven is eight
eight bits here the here the
representation is using eight
eight bits and then j is equals to i and
and mask is so and and and mask
is equal to we'll shift one so we'll
shift one
by the number of bits we want to check
right so if you're checking the seventh
seventh bit one will be shifted by seven
times
and k is equals to n into n mask right
so
n n will be so n is the number which we
have passed in the function the
third decimal number and this this
and mask and if the product
if k is a non-zero value it will print
one
and if it's a zero and if k is zero then
it will print
zero right so if i so let's say if i
passed
so let's say if i pass 32 here then then
it should
print the binary sequence of 32
that's exactly what it is what it will
print
uh double zero one and then five times
zero because that's what 32 is
right so 2 to the power 0 2 to the power
1 2 to the power 2 2 to the power 3 2 to
the power 4 2 to the power 5
so um 1 0 0 0 0
0 okay so that's what 32 is
and it will and show bits will will
print the
binary representation of the of
the decimal number passed in the
function
how
show bits function work so we're going
to print the binary representation of a
decimal number okay
so the decimal number here is 39 so if i
run the program
it runs the binary representation of
this
of this decimal number which is 39 right
this is a binary representation of 39
which has been
printed uh using this function show bits
all right so so normally when we want to
convert
a decimal number to binary
right so i will take 39 and i'll divide
it by two
and uh so
so i'll get 19 and the remainder will be
one
and then i'll again divide it by two so
two into nine is
eighteen which will throw a remainder
one
and then two into four is eight which
will again throw a remainder one
and then again i'll divide 2 into 2 is 4
and the remainder is 0. and then 2 into
1 is demeter is again 0.
okay so 1 triple 1 double 0 and then
triple 1 this is
binary okay and 39 is
yeah so so this is the binary
representation of
39 right so we can we can we can
implement this
in c but a more efficient way of
of achieving this uh of of achieving
this will be
using and mask right so what do we do
here
is we start a loop so you guys
so you can see the representation here
is eight eight bits
right so we'll start a loop from i is
equals to seven
okay and we'll take it
all the way to zero and then
uh sorry it's not less than it's greater
than equal to zero and then i minus
minus for each
um so it will it will decrement until it
reaches zero right and
and then with and then with each and
then with each iteration i'll store this
i
and then i'll create an and mask which
is
which is i'll shift one by
j bits so for the first time
so so let's say at first iteration
j is equals to seven okay uh so if i
shift one by seven bits it's one and
then
seven seven zeros all right so let's say
so one of the so one of the use of and
mask
is to check whether a bit is true or
false
right suppose i pass a bit
39 right where for which the
binary code is for which the binary code
is zero zero one zero zero
triple one zero zero one zero zero
triple one
okay that's the binary code for for 39
right
so if i um that's the binary code for
that's the binary code for 39 right
so so if i want to check that the
seventh bit
is zero or not i will simply
multiply this so let this number
so let this decimal number be n
and i'll simply simply perform a bitwise
and with and mask
so so if seventh bit is on
that means if seventh bit is one right
it will
return one or if the seventh bit is zero
it will return zero
right so zero zero one
double zero and then triple one all
right
so if i take bitwise and of
of both these uh sequences i'll get
zero right so zero for all the
so zero if i take end of both these
numbers
because the bit was actually zero right
now let's say now let's say we want to
check this
this one this is the fifth fifth bit
this is the seven bit six
with fifth bit right so if i want to
check for fifth bit so here it will be
zero and seven six
five right so rest rest all of them will
turn
zero and this one will be and
this one will be one right so
this value is something but it's not
zero it's a non-zero value
right so when it returns a non-zero
value
when we take a bitwise and of n
with uh with and mask
if it returns a non-zero value
that means this bit was on that means
the fifth bit was
on that means the fifth bit was one
right so that's exactly what we'll check
check here
if k equals to zero it should
print zero right
else it should print one that means if
it's a non-zero value
it should print one okay so
that's that's exactly what we have
implemented
implemented here in show bits right
uh i have taken the i'll pass the
unsigned
i'll pass the unsigned character inside
show bits
right so here so n will be the so n will
be
the decimal number and then the loop
will
start from seven to zero because zero to
seven is eight
eight bits here the here the
representation is using eight
eight bits and then j is equals to i and
and mask is so and and and mask
is equal to we'll shift one so we'll
shift one
by the number of bits we want to check
right so if you're checking the seventh
seventh bit one will be shifted by seven
times
and k is equals to n into n mask right
so
n n will be so n is the number which we
have passed in the function the
third decimal number and this this
and mask and if the product
if k is a non-zero value it will print
one
and if it's a zero and if k is zero then
it will print
zero right so if i so let's say if i
passed
so let's say if i pass 32 here then then
it should
print the binary sequence of 32
that's exactly what it is what it will
uh double zero one and then five times
zero because that's what 32 is
right so 2 to the power 0 2 to the power
1 2 to the power 2 2 to the power 3 2 to
the power 4 2 to the power 5
so um 1 0 0 0 0
0 okay so that's what 32 is
and it will and show bits will will
print the
binary representation of the of
the decimal number passed in the
function