filmov
tv
Converting XLSX File to CSV in JavaScript
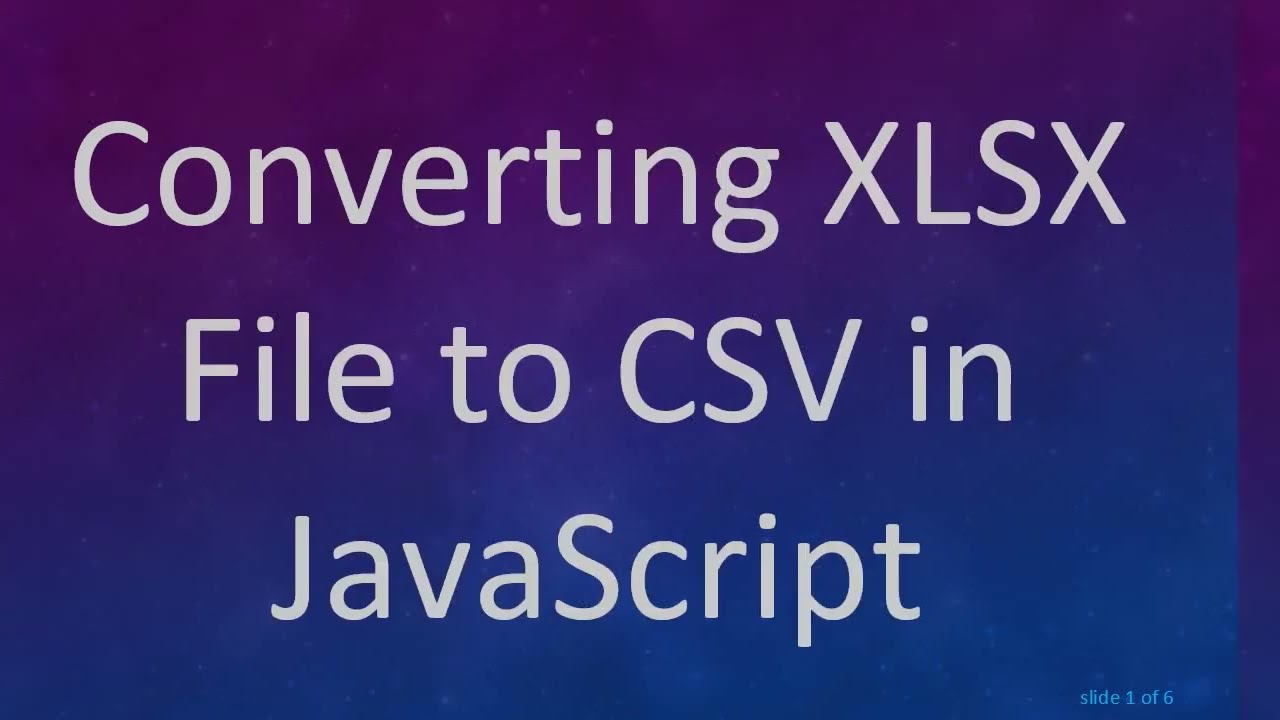
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Learn how to convert XLSX files to CSV using JavaScript. Explore examples and code snippets for effective conversion in your projects.
---
In many web development projects, handling spreadsheet data is a common requirement. Excel files in the XLSX format are widely used for storing tabular data, but there are instances where you might need to convert them to the more lightweight CSV (Comma-Separated Values) format. In this guide, we'll explore how to achieve this conversion using JavaScript, specifically leveraging the xlsx library.
Prerequisites
Before we begin, make sure you have the necessary dependencies installed. You can use a package manager like npm to install the required library.
[[See Video to Reveal this Text or Code Snippet]]
The Code
Let's delve into the code. The process involves loading the XLSX file, extracting the data, and then converting it to CSV. Here's a simple example using JavaScript:
[[See Video to Reveal this Text or Code Snippet]]
Example Walkthrough
Let's break down the example:
Importing the Library: We import the xlsx library, which provides functions for working with Excel files.
Reading the XLSX File: The readFile function is used to load the XLSX file.
Extracting the Sheet: We extract the first sheet from the workbook. You can modify this based on your sheet selection criteria.
Converting to CSV: The sheet_to_csv function converts the sheet data to CSV format.
Logging CSV Data: Finally, we log the CSV data to the console. You can choose to save it to a file or further process it based on your needs.
Handling File Input
If you are working in a browser environment and want to allow users to upload XLSX files, you can use the following HTML and JavaScript code:
[[See Video to Reveal this Text or Code Snippet]]
This example provides a simple web interface for users to upload an XLSX file, convert it to CSV, and display the result.
Conclusion
Explore further customization based on your specific requirements, and feel free to integrate error handling and additional features to enhance the user experience.
---
Summary: Learn how to convert XLSX files to CSV using JavaScript. Explore examples and code snippets for effective conversion in your projects.
---
In many web development projects, handling spreadsheet data is a common requirement. Excel files in the XLSX format are widely used for storing tabular data, but there are instances where you might need to convert them to the more lightweight CSV (Comma-Separated Values) format. In this guide, we'll explore how to achieve this conversion using JavaScript, specifically leveraging the xlsx library.
Prerequisites
Before we begin, make sure you have the necessary dependencies installed. You can use a package manager like npm to install the required library.
[[See Video to Reveal this Text or Code Snippet]]
The Code
Let's delve into the code. The process involves loading the XLSX file, extracting the data, and then converting it to CSV. Here's a simple example using JavaScript:
[[See Video to Reveal this Text or Code Snippet]]
Example Walkthrough
Let's break down the example:
Importing the Library: We import the xlsx library, which provides functions for working with Excel files.
Reading the XLSX File: The readFile function is used to load the XLSX file.
Extracting the Sheet: We extract the first sheet from the workbook. You can modify this based on your sheet selection criteria.
Converting to CSV: The sheet_to_csv function converts the sheet data to CSV format.
Logging CSV Data: Finally, we log the CSV data to the console. You can choose to save it to a file or further process it based on your needs.
Handling File Input
If you are working in a browser environment and want to allow users to upload XLSX files, you can use the following HTML and JavaScript code:
[[See Video to Reveal this Text or Code Snippet]]
This example provides a simple web interface for users to upload an XLSX file, convert it to CSV, and display the result.
Conclusion
Explore further customization based on your specific requirements, and feel free to integrate error handling and additional features to enhance the user experience.