filmov
tv
Advanced C++/Graphics Tutorial 22 pt.1: SpriteBatch, VAO, std::sort
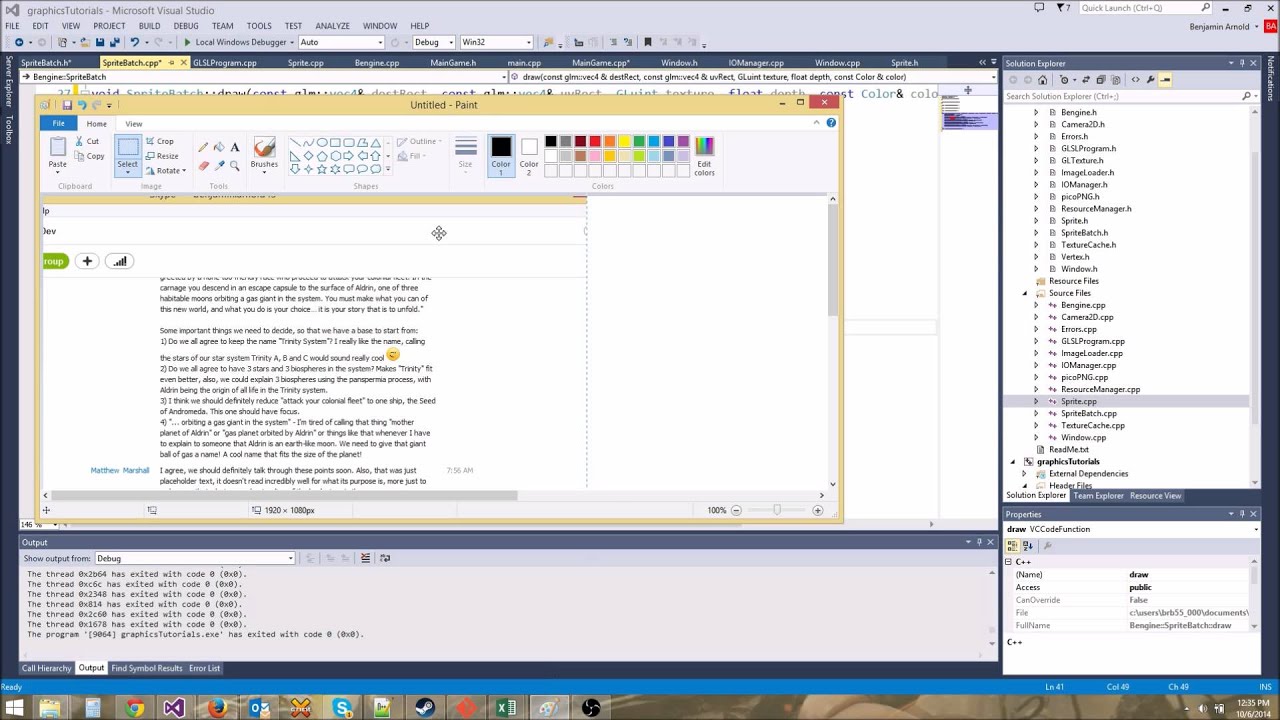
Показать описание
Source code is in part two!
Today we begin creating the SpriteBatch class, which will help us optimize our rendering! This is a rather long video so I split it into two parts! You will learn a lot today :)
Today we begin creating the SpriteBatch class, which will help us optimize our rendering! This is a rather long video so I split it into two parts! You will learn a lot today :)
Комментарии