filmov
tv
Frequently Asked Java Program 16: How To Check The Equality Of Two Arrays
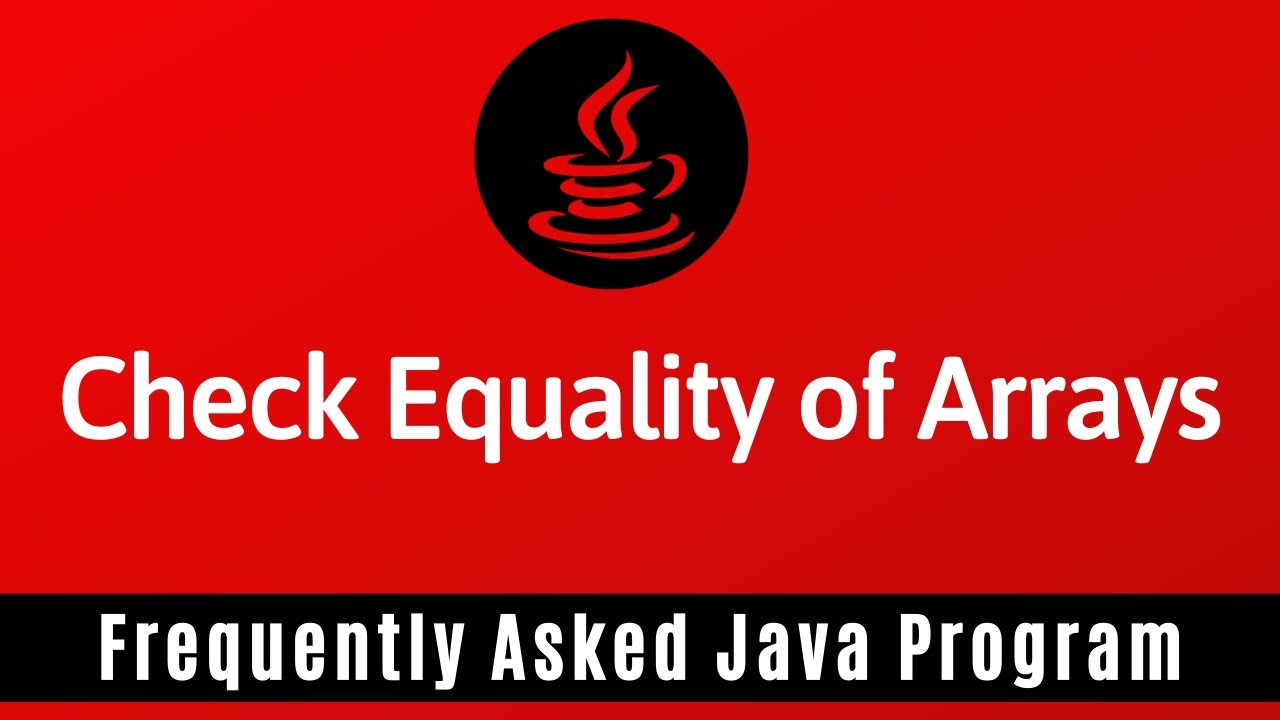
Показать описание
Topic : How To Check The Equality Of Two Arrays
#########################
Udemy Courses:
#########################
Manual Testing+Agile with Jira Tool
************************************
Selenium with Java+Cucumber
********************************
Selenium with Python & PyTest
********************************
Selenium with python using Robot framework
****************************************
API Testing(Postman, RestAssured & SoapUI)
*****************************************
Web & API Automation using Cypress with Javascript
********************************************
Playwright with Javascript
**************************
Jmeter-Performance Testing
************************
SDET Essencials(Full Stack QA)
*************************
Appium-Mobile Automation Testing
************************************
Java Collections
*****************
Python Programming
*********************
Cucumber BDD Framework
***************************
Protractor with Javascript
***************************
####################################
Youtube Playlists:
####################################
Manual Testing & Agile
***********************
SQL
*************************
linux & Shell Scripting
**********************
Java
**********************
Selenium With Java+Cucumber
********************************
Python
********************************
Selenium With Python,Pytest&Behave
***************************************
Selenium With Python Using Robert Framework
(Web&API Testing)
*************************************************
API Testing (Postman,SoapUi,&Rest Assured)
**********************************************
Mobile App Testing Appium
****************************
Performance Testing Jmeter
*******************************
Maven,Jenkins,Git,Github,CI/CD
*******************************
SQL,DB Testing&ETL,Bigdata
*******************************
JavaScript Based Automation Tools
********************************
Selector Hub Tools
********************
GraphQL
******************
Cypress API Testing
********************
Cypress Web Testing
**********************
Playwright with Javascipt
**************************
#PythonArrayEquality
#ArrayComparison
#ArrayEqualityCheck
#PythonArrays
#ArrayMatching
#CompareArrays
#ArrayEqualityProgram
#PythonCoding
#ArrayOperations
#CodeLogic
#PythonProgramming
#CodingChallenge
#ArrayLogic
#ProgrammingPractice
#EqualityCheck
#AlgorithmDesign
#PythonSolutions
#ArrayProblems
#CodingSkills
#PythonSnippets
#ArrayPuzzles
#AlgorithmAnalysis
#ProblemSolving
#ArrayAlgorithms
#PythonCodingTips
#ArrayComparisonLogic
#CodeOptimization
#PythonLearning
#ArrayPatterns
#AlgorithmApproach
#CodingCommunity
#PythonHelp
#ArrayTricks
#ProblemSolvingInPython
#AlgorithmTricks
#CodingInPython
#ArrayManipulation
#PythonTricks
#AlgorithmExplained
#CodeBreakdown
#PythonArrays101
#AlgorithmMastery
#CodingInspiration
#PythonExploration
#ArrayTips
#AlgorithmHacks
#CodingGenius
#PythonCodeSnippets
#ArrayComparisonTricks
#AlgorithmSolutions
#########################
Udemy Courses:
#########################
Manual Testing+Agile with Jira Tool
************************************
Selenium with Java+Cucumber
********************************
Selenium with Python & PyTest
********************************
Selenium with python using Robot framework
****************************************
API Testing(Postman, RestAssured & SoapUI)
*****************************************
Web & API Automation using Cypress with Javascript
********************************************
Playwright with Javascript
**************************
Jmeter-Performance Testing
************************
SDET Essencials(Full Stack QA)
*************************
Appium-Mobile Automation Testing
************************************
Java Collections
*****************
Python Programming
*********************
Cucumber BDD Framework
***************************
Protractor with Javascript
***************************
####################################
Youtube Playlists:
####################################
Manual Testing & Agile
***********************
SQL
*************************
linux & Shell Scripting
**********************
Java
**********************
Selenium With Java+Cucumber
********************************
Python
********************************
Selenium With Python,Pytest&Behave
***************************************
Selenium With Python Using Robert Framework
(Web&API Testing)
*************************************************
API Testing (Postman,SoapUi,&Rest Assured)
**********************************************
Mobile App Testing Appium
****************************
Performance Testing Jmeter
*******************************
Maven,Jenkins,Git,Github,CI/CD
*******************************
SQL,DB Testing&ETL,Bigdata
*******************************
JavaScript Based Automation Tools
********************************
Selector Hub Tools
********************
GraphQL
******************
Cypress API Testing
********************
Cypress Web Testing
**********************
Playwright with Javascipt
**************************
#PythonArrayEquality
#ArrayComparison
#ArrayEqualityCheck
#PythonArrays
#ArrayMatching
#CompareArrays
#ArrayEqualityProgram
#PythonCoding
#ArrayOperations
#CodeLogic
#PythonProgramming
#CodingChallenge
#ArrayLogic
#ProgrammingPractice
#EqualityCheck
#AlgorithmDesign
#PythonSolutions
#ArrayProblems
#CodingSkills
#PythonSnippets
#ArrayPuzzles
#AlgorithmAnalysis
#ProblemSolving
#ArrayAlgorithms
#PythonCodingTips
#ArrayComparisonLogic
#CodeOptimization
#PythonLearning
#ArrayPatterns
#AlgorithmApproach
#CodingCommunity
#PythonHelp
#ArrayTricks
#ProblemSolvingInPython
#AlgorithmTricks
#CodingInPython
#ArrayManipulation
#PythonTricks
#AlgorithmExplained
#CodeBreakdown
#PythonArrays101
#AlgorithmMastery
#CodingInspiration
#PythonExploration
#ArrayTips
#AlgorithmHacks
#CodingGenius
#PythonCodeSnippets
#ArrayComparisonTricks
#AlgorithmSolutions
Комментарии