filmov
tv
Streamline Your JavaScript: A Better Way to Extract Values from Strings
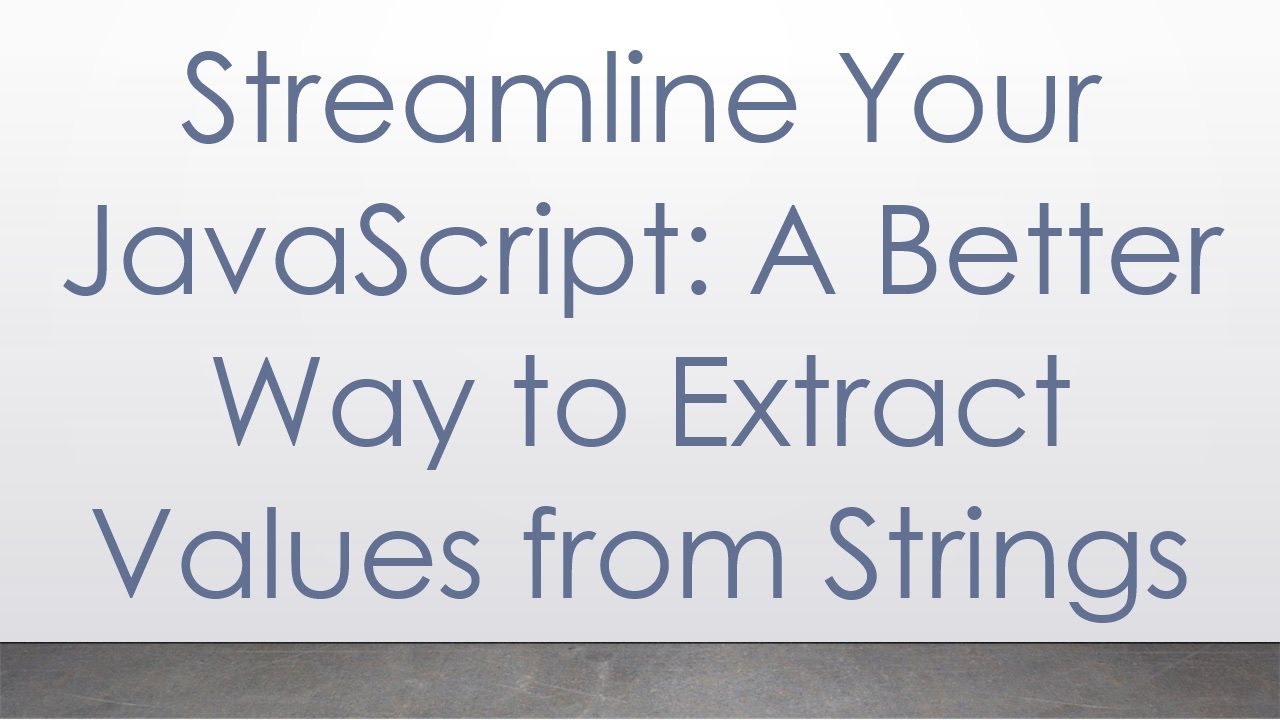
Показать описание
Discover a more efficient method to extract text after `=` in JavaScript using map, optimizing code for readability and performance.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: I need to extract text after = for each value seperated by semicolon in javascript. I have done it but wondering if there is a better approach
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Streamline Your JavaScript: A Better Way to Extract Values from Strings
When working with strings in JavaScript, you might encounter scenarios where you need to extract specific values. A common task is retrieving text that follows an equals sign (=) for each value separated by a semicolon. Many developers have their own methods for accomplishing this, but sometimes a more efficient approach can simplify the code and improve readability. In this guide, we will explore a common solution to this problem and refine it for optimal performance and clarity.
The Challenge: Extracting Values from a String
Consider this example string:
[[See Video to Reveal this Text or Code Snippet]]
Our goal is to extract everything that comes after the = sign in each segment, which results in an array containing ["*aaa", "*bbb"]. Here's the initial solution that many may use, often involving splitting the string, iterating through each element, and pushing results into an array.
Original Working Code
Here’s how the original code structure looks:
[[See Video to Reveal this Text or Code Snippet]]
While this code works, it may not be the most efficient way to achieve this objective.
Enhancing the Solution
Let’s improve this code by employing three key strategies:
1. Use const instead of let
Since we are creating an array (params) that we will fill but not reassign, we can declare it using const for better code practices.
2. Merge Statements in the Loop
The original code splits each element and saves it into a temporary variable (zz) that is not reused. Instead, we can directly use the split result.
3. Utilize the .map() Method
Rather than first creating an empty array and then populating it, we can use the .map() method to transform the initial array into the desired array with only the needed values. This leads to cleaner, more concise code.
The Refined Code
After applying these strategies, here's how the optimized code looks:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Optimized Code
const Declaration: const params ensures that the variable cannot be reassigned, maintaining clarity.
Single .map() Call: We split the string directly and immediately extract the value after = using the map() function, which makes the operation more linear and reduces the number of loops.
Conclusion
By optimizing JavaScript code in this manner, not only do we make it more concise and efficient, but we also enhance its readability and maintainability. Leveraging methods like .map() allows you to write cleaner, more functional-style code that aligns with modern JavaScript practices.
Why settle for less when you can streamline your JavaScript code? Embrace these small changes for a more effective coding experience!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: I need to extract text after = for each value seperated by semicolon in javascript. I have done it but wondering if there is a better approach
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Streamline Your JavaScript: A Better Way to Extract Values from Strings
When working with strings in JavaScript, you might encounter scenarios where you need to extract specific values. A common task is retrieving text that follows an equals sign (=) for each value separated by a semicolon. Many developers have their own methods for accomplishing this, but sometimes a more efficient approach can simplify the code and improve readability. In this guide, we will explore a common solution to this problem and refine it for optimal performance and clarity.
The Challenge: Extracting Values from a String
Consider this example string:
[[See Video to Reveal this Text or Code Snippet]]
Our goal is to extract everything that comes after the = sign in each segment, which results in an array containing ["*aaa", "*bbb"]. Here's the initial solution that many may use, often involving splitting the string, iterating through each element, and pushing results into an array.
Original Working Code
Here’s how the original code structure looks:
[[See Video to Reveal this Text or Code Snippet]]
While this code works, it may not be the most efficient way to achieve this objective.
Enhancing the Solution
Let’s improve this code by employing three key strategies:
1. Use const instead of let
Since we are creating an array (params) that we will fill but not reassign, we can declare it using const for better code practices.
2. Merge Statements in the Loop
The original code splits each element and saves it into a temporary variable (zz) that is not reused. Instead, we can directly use the split result.
3. Utilize the .map() Method
Rather than first creating an empty array and then populating it, we can use the .map() method to transform the initial array into the desired array with only the needed values. This leads to cleaner, more concise code.
The Refined Code
After applying these strategies, here's how the optimized code looks:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Optimized Code
const Declaration: const params ensures that the variable cannot be reassigned, maintaining clarity.
Single .map() Call: We split the string directly and immediately extract the value after = using the map() function, which makes the operation more linear and reduces the number of loops.
Conclusion
By optimizing JavaScript code in this manner, not only do we make it more concise and efficient, but we also enhance its readability and maintainability. Leveraging methods like .map() allows you to write cleaner, more functional-style code that aligns with modern JavaScript practices.
Why settle for less when you can streamline your JavaScript code? Embrace these small changes for a more effective coding experience!