filmov
tv
Streamline Your JavaScript Code: Using Foreach to Handle Checkboxes Efficiently
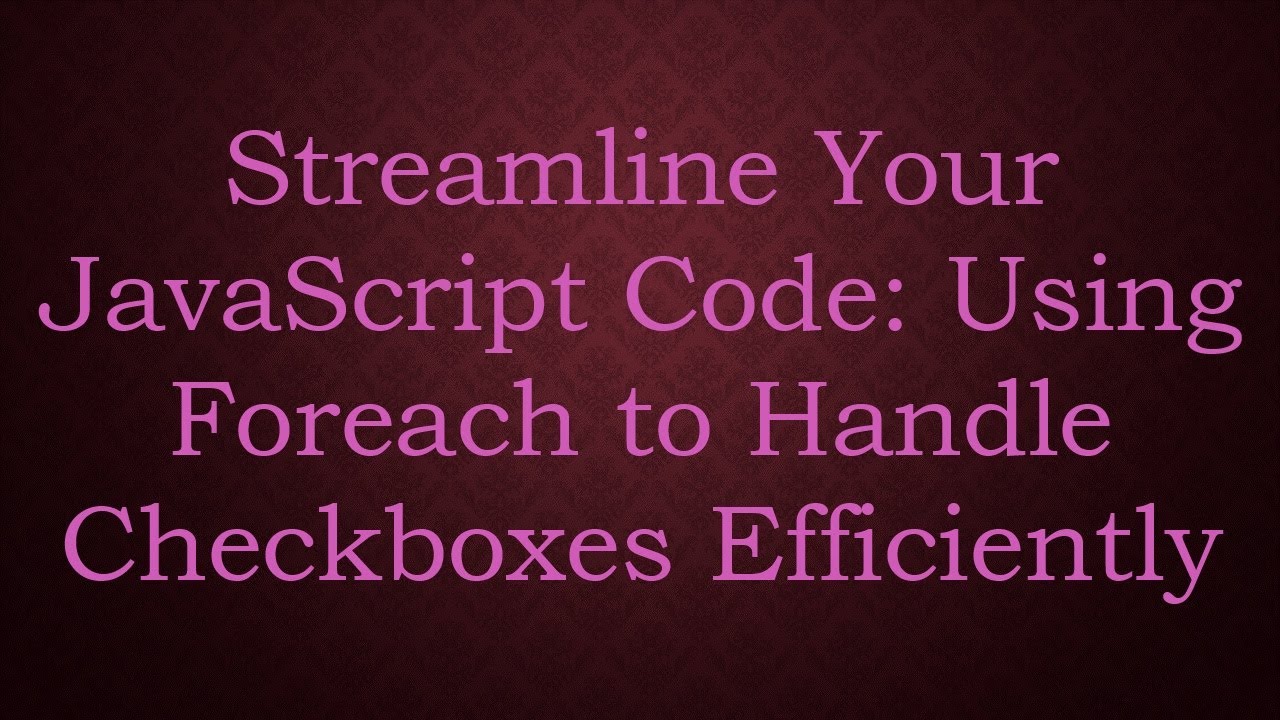
Показать описание
Discover how to use the `foreach` function in JavaScript to simplify your checkbox handling code and enhance its maintainability.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Using Foreach or another function to reduce the amount of code
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Streamline Your JavaScript Code: Using Foreach to Handle Checkboxes Efficiently
In the world of web development, writing clean and efficient code is essential. Many developers often face situations where they have repetitive code blocks that can be optimized or shortened. A common scenario is handling multiple checkboxes within a form. If you find yourself writing code that looks redundant and lengthy when checking the state of each checkbox, you’re not alone! In this guide, we will explore a more streamlined approach using the foreach function in JavaScript (with jQuery) to reduce the amount of code needed to manage checkboxes effectively.
The Problem: Repetitive Checkbox Code
Here’s a typical example of code you might find yourself writing to check if various checkboxes are selected:
[[See Video to Reveal this Text or Code Snippet]]
While this approach works, it’s clear that it can be cumbersome and challenging to maintain as the number of checkboxes grows. If you need to add or remove checkboxes, you would have to modify the code in multiple places, which increases the likelihood of errors.
The Solution: Using a forEach Loop
The good news is that you can simplify this logic by using jQuery’s each function. Instead of manually checking each checkbox, we can collect their states dynamically. Here’s a streamlined solution:
Step-by-Step Breakdown
Create an Empty Object: We will start by creating an empty object where we will store the checkbox states.
Select All Checkboxes: Use jQuery to select all the checkbox inputs on the page.
Loop Through Each Checkbox: Utilize the each function to iterate through the selected checkboxes.
Store Checkbox ID and State: For each checkbox, we will get its ID and whether it’s checked or not, then store that information in our object.
Sample Code
Here’s how the optimized code looks:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
var jsonObject = {};: This initializes an empty object to hold our checkbox data.
$('input[type=checkbox]').each(...): This jQuery selector finds all checkbox inputs and loops through them one by one.
$(this).attr('id'): Inside the loop, $(this) refers to the current checkbox being processed. We extract its ID using attr('id').
$(this).is(':checked'): This checks whether the current checkbox is checked (true) or unchecked (false).
Benefits of This Approach
Reduced Code Complexity: Minimal lines of code and reduced redundancy.
Easy Maintenance: Adding or removing checkboxes doesn’t require code changes at multiple locations, making it easier to manage.
Dynamic: Automatically collects data for all checkboxes, so no need to hard-code IDs.
Conclusion
Using the foreach loop in JavaScript for handling checkbox states not only makes your code cleaner but also enhances its functionality and maintainability. This optimization is especially beneficial in larger forms or dynamic applications where the number of inputs may frequently change. So next time you’re faced with repetitive code, consider whether a loop can save you time and improve your code quality! Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Using Foreach or another function to reduce the amount of code
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Streamline Your JavaScript Code: Using Foreach to Handle Checkboxes Efficiently
In the world of web development, writing clean and efficient code is essential. Many developers often face situations where they have repetitive code blocks that can be optimized or shortened. A common scenario is handling multiple checkboxes within a form. If you find yourself writing code that looks redundant and lengthy when checking the state of each checkbox, you’re not alone! In this guide, we will explore a more streamlined approach using the foreach function in JavaScript (with jQuery) to reduce the amount of code needed to manage checkboxes effectively.
The Problem: Repetitive Checkbox Code
Here’s a typical example of code you might find yourself writing to check if various checkboxes are selected:
[[See Video to Reveal this Text or Code Snippet]]
While this approach works, it’s clear that it can be cumbersome and challenging to maintain as the number of checkboxes grows. If you need to add or remove checkboxes, you would have to modify the code in multiple places, which increases the likelihood of errors.
The Solution: Using a forEach Loop
The good news is that you can simplify this logic by using jQuery’s each function. Instead of manually checking each checkbox, we can collect their states dynamically. Here’s a streamlined solution:
Step-by-Step Breakdown
Create an Empty Object: We will start by creating an empty object where we will store the checkbox states.
Select All Checkboxes: Use jQuery to select all the checkbox inputs on the page.
Loop Through Each Checkbox: Utilize the each function to iterate through the selected checkboxes.
Store Checkbox ID and State: For each checkbox, we will get its ID and whether it’s checked or not, then store that information in our object.
Sample Code
Here’s how the optimized code looks:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
var jsonObject = {};: This initializes an empty object to hold our checkbox data.
$('input[type=checkbox]').each(...): This jQuery selector finds all checkbox inputs and loops through them one by one.
$(this).attr('id'): Inside the loop, $(this) refers to the current checkbox being processed. We extract its ID using attr('id').
$(this).is(':checked'): This checks whether the current checkbox is checked (true) or unchecked (false).
Benefits of This Approach
Reduced Code Complexity: Minimal lines of code and reduced redundancy.
Easy Maintenance: Adding or removing checkboxes doesn’t require code changes at multiple locations, making it easier to manage.
Dynamic: Automatically collects data for all checkboxes, so no need to hard-code IDs.
Conclusion
Using the foreach loop in JavaScript for handling checkbox states not only makes your code cleaner but also enhances its functionality and maintainability. This optimization is especially beneficial in larger forms or dynamic applications where the number of inputs may frequently change. So next time you’re faced with repetitive code, consider whether a loop can save you time and improve your code quality! Happy coding!