filmov
tv
Simplifying Your JavaScript Logic: A Streamlined Approach to If/Else Statements
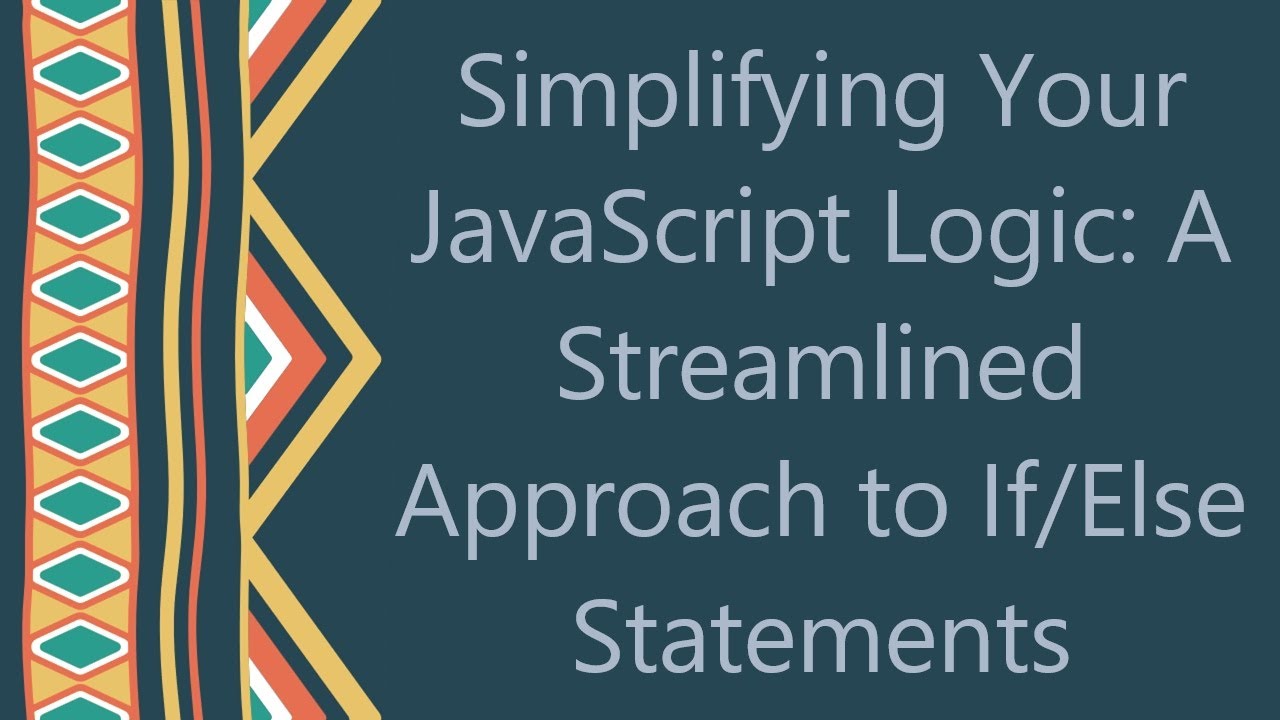
Показать описание
Discover how to simplify your JavaScript `if/else` statements by eliminating unnecessary variables. This guide explores an efficient method to enhance user interaction in a quiz application.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Is there a simpler way to write this if/else statement without a variable?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Simplifying Your JavaScript Logic: A Streamlined Approach to If/Else Statements
When writing JavaScript code, especially for interactive applications like quizzes, you may encounter scenarios where your if/else statements become cumbersome. One common challenge is handling user inputs without the need for temporary variables. In this guide, we will explore how to simplify your code and improve functionality by using classes or data attributes instead of additional variables.
The Problem: Managing User Inputs in a Quiz
Suppose you're developing an interactive quiz where users can submit their answers by pressing the "Enter" key. The first press should grade the answer, while the second press resets the quiz for a new question. Initially, you might write your code using a variable (flip in this case) to track whether the quiz is in a grading or resetting state. Here’s an example of a typical implementation:
[[See Video to Reveal this Text or Code Snippet]]
While this code works, it's not the most efficient approach. Using a variable (flip) to control the flow of logic can complicate your code.
The Solution: Using Classes to Simplify Logic
Instead of maintaining a separate variable to track the state, you can utilize CSS classes to manage the logic. This approach leverages the inherent functionality of JavaScript, making your code cleaner and easier to follow. Below, we will refactor the previous code example without the flip variable.
Code Refactoring
Here’s how you can rewrite your event listener to use a class instead:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the New Code
Event Listener: The code begins by attaching an event listener to the input element. It listens for the "keyup" event and checks if the pressed key is "Enter".
State Management with Classes:
The tgt variable stores the reference to the input element.
If it doesn’t have the class, the code calls the reset() function to prepare for a new question.
Conclusion
By utilizing CSS classes instead of a temporary variable, you can simplify your JavaScript logic significantly. This method not only reduces the amount of code but also enhances readability, making it easier for others (and your future self) to understand. Implementing classes this way can streamline your code, especially in interactive applications like quizzes. So next time you find yourself using unnecessary variables, consider using the power of class manipulation to keep your code clean and efficient.
Keep coding smart, and happy programming!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Is there a simpler way to write this if/else statement without a variable?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Simplifying Your JavaScript Logic: A Streamlined Approach to If/Else Statements
When writing JavaScript code, especially for interactive applications like quizzes, you may encounter scenarios where your if/else statements become cumbersome. One common challenge is handling user inputs without the need for temporary variables. In this guide, we will explore how to simplify your code and improve functionality by using classes or data attributes instead of additional variables.
The Problem: Managing User Inputs in a Quiz
Suppose you're developing an interactive quiz where users can submit their answers by pressing the "Enter" key. The first press should grade the answer, while the second press resets the quiz for a new question. Initially, you might write your code using a variable (flip in this case) to track whether the quiz is in a grading or resetting state. Here’s an example of a typical implementation:
[[See Video to Reveal this Text or Code Snippet]]
While this code works, it's not the most efficient approach. Using a variable (flip) to control the flow of logic can complicate your code.
The Solution: Using Classes to Simplify Logic
Instead of maintaining a separate variable to track the state, you can utilize CSS classes to manage the logic. This approach leverages the inherent functionality of JavaScript, making your code cleaner and easier to follow. Below, we will refactor the previous code example without the flip variable.
Code Refactoring
Here’s how you can rewrite your event listener to use a class instead:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the New Code
Event Listener: The code begins by attaching an event listener to the input element. It listens for the "keyup" event and checks if the pressed key is "Enter".
State Management with Classes:
The tgt variable stores the reference to the input element.
If it doesn’t have the class, the code calls the reset() function to prepare for a new question.
Conclusion
By utilizing CSS classes instead of a temporary variable, you can simplify your JavaScript logic significantly. This method not only reduces the amount of code but also enhances readability, making it easier for others (and your future self) to understand. Implementing classes this way can streamline your code, especially in interactive applications like quizzes. So next time you find yourself using unnecessary variables, consider using the power of class manipulation to keep your code clean and efficient.
Keep coding smart, and happy programming!