filmov
tv
OpenGL 3D Game Tutorial 27: Skybox
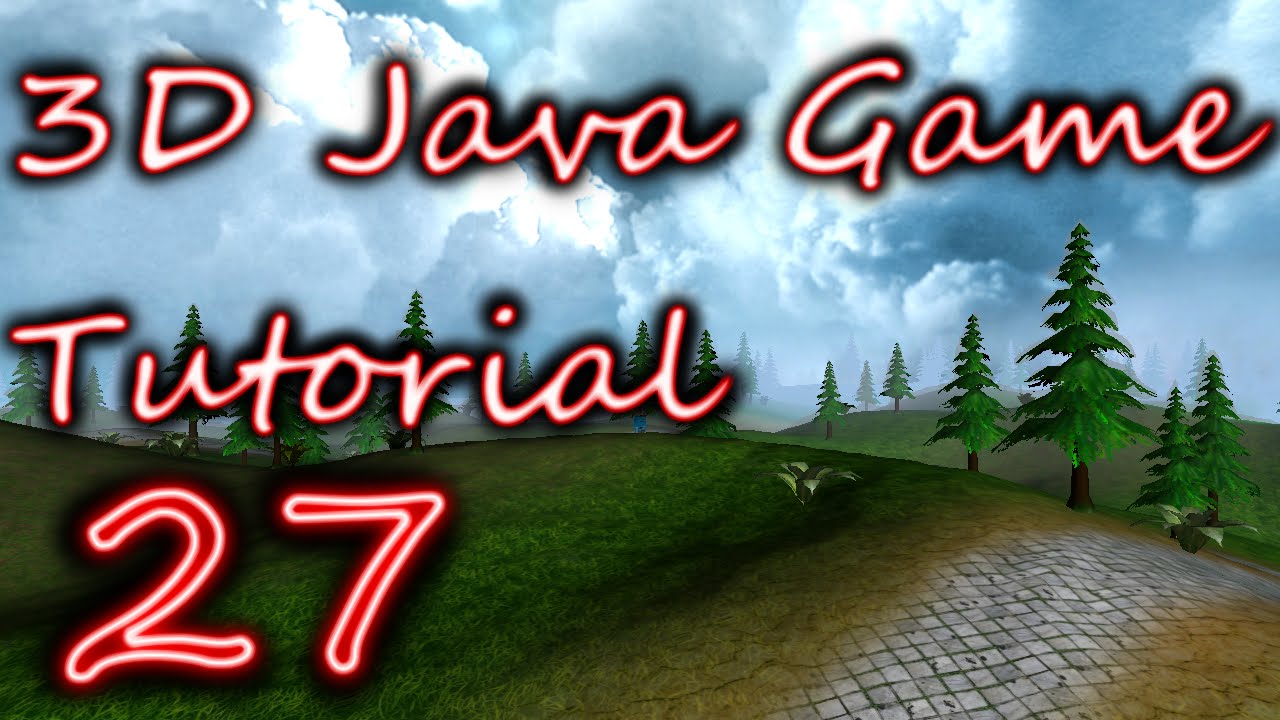
Показать описание
LWJGL tutorial series on how to create a 3D Java game with OpenGL!
Programming a skybox this week using cube map textures!
Common Problems:
-Due to hardware limitations on some computers you may see some visible seams at the edges of the skybox. If this is the case then add these two lines to the end of the loadCubeMap() method, just before returning the texID:
End of video music- Kai Engel, "Waking Stars":
Programming a skybox this week using cube map textures!
Common Problems:
-Due to hardware limitations on some computers you may see some visible seams at the edges of the skybox. If this is the case then add these two lines to the end of the loadCubeMap() method, just before returning the texID:
End of video music- Kai Engel, "Waking Stars":
OpenGL 3D Game Tutorial 27: Skybox
OpenGL Tutorial 27 - Normal Maps
OpenGL 3D Game Tutorial 29: Mouse Picking
OpenGL 3D Game Tutorial 37: Procedural Terrain
OpenGL 3D Game Tutorial 1: The Display
OpenGL/C++ 3D Tutorial 27 - Fleshing out the Mesh class and removing old code!
Creating A Modern OpenGL Game Engine - Day 27 [IMPORTANT ANNOUNCEMENT]
OpenGL 3D Game Tutorial 32: Font Rendering
OpenGL 3D Game Tutorial 8: Model, View & Projection Matrices
OpenGL 3D Game Tutorial 35: Animating Particle Textures
OpenGL 3D Game Tutorial 21: Terrain Height Maps
Framebuffers in OpenGL | Coding a 2D Game Engine in Java #27
OpenGL 3D Game Tutorial 10: Loading 3D Models
27. Computer Graphics Using OpenGL
Dog Model Created with OpenGL
The HARDEST part about programming 🤦♂️ #code #programming #technology #tech #software #developer...
Java OpenGL 3D game test
Spring Motion in 3D - OpenGL (2)
OpenGL to Vulkan #coding #gamedev #gameengine
Draw AC Milan Logo using OpenGL C++ GLUT
OpenGL 3D Game Tutorial 31: Normal Mapping
The quickest tutorial for 2d animation in #blender3d
OpenGL 3D Game Tutorial 13: Optimizing
3D Textures in OpenGL, Looped
Комментарии