filmov
tv
Tree traversal algorithms (BFS and DFS - Preorder, Inorder, Postorder)
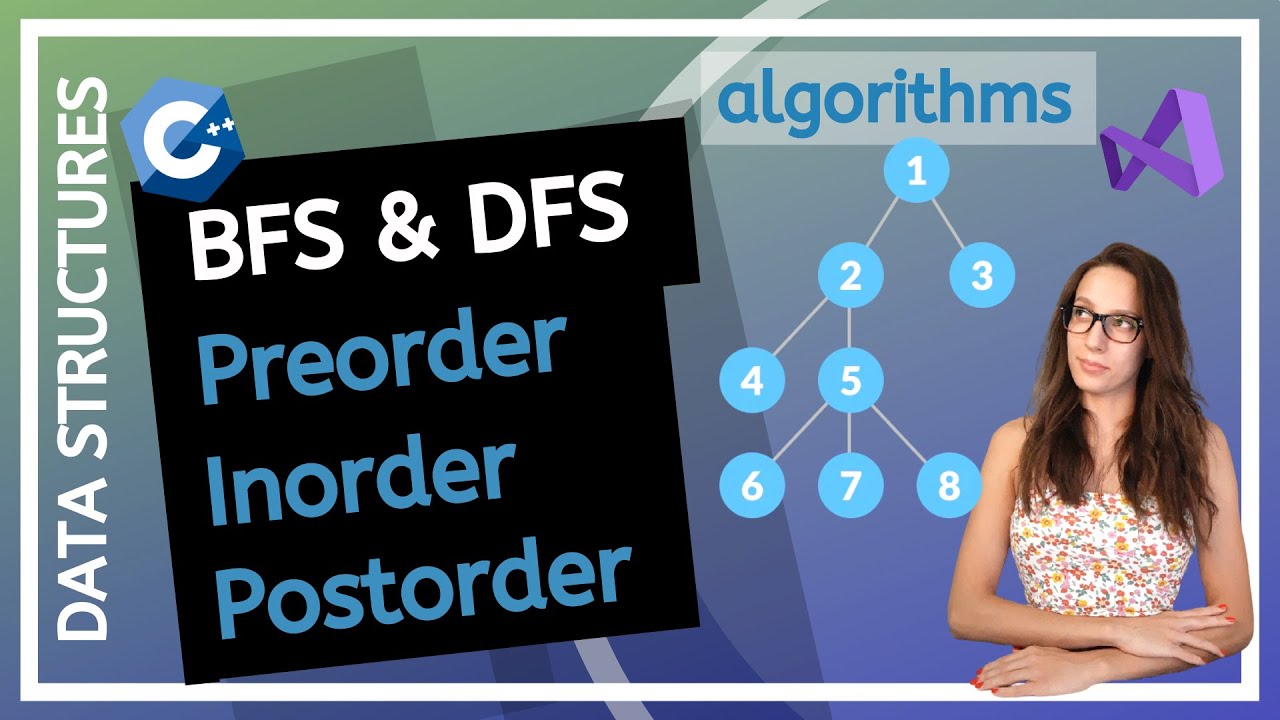
Показать описание
📚 Learn how to solve problems and build projects with these Free E-Books ⬇️
Experience the power of practical learning, gain career-ready skills, and start building real applications!
This is a step-by-step course designed to take you from beginner to expert in no time!
💰 Here is a coupon to save 10% on your first payment (CODEBEAUTY_YT10).
Use it quickly, because it will be available for a limited time.
Trees are an important data structure because of their non-linear nature, which allows for a faster response time during a search process. So in this video, I decided to teach you about the most important algorithms and ways to search and traverse a binary tree.
After watching the video you'll learn and understand the differences between BFS (breadth-first search) and DFS (depth-first search) algorithms, and also learn everything you need to know about Preorder, Inorder, and Postorder algorithms.
Contents:
00:00 - Introduction
02:38 - BFS and DFS
04:32 - Preorder algorithm
08:38 - Traversing a tree with recursion (explaining the code)
15:34 - Inorder algorithm
19:49 - Postorder algorithm
Follow me on other platforms:
Experience the power of practical learning, gain career-ready skills, and start building real applications!
This is a step-by-step course designed to take you from beginner to expert in no time!
💰 Here is a coupon to save 10% on your first payment (CODEBEAUTY_YT10).
Use it quickly, because it will be available for a limited time.
Trees are an important data structure because of their non-linear nature, which allows for a faster response time during a search process. So in this video, I decided to teach you about the most important algorithms and ways to search and traverse a binary tree.
After watching the video you'll learn and understand the differences between BFS (breadth-first search) and DFS (depth-first search) algorithms, and also learn everything you need to know about Preorder, Inorder, and Postorder algorithms.
Contents:
00:00 - Introduction
02:38 - BFS and DFS
04:32 - Preorder algorithm
08:38 - Traversing a tree with recursion (explaining the code)
15:34 - Inorder algorithm
19:49 - Postorder algorithm
Follow me on other platforms:
Комментарии