filmov
tv
Binary Tree Level Order Traversal - BFS - Leetcode 102
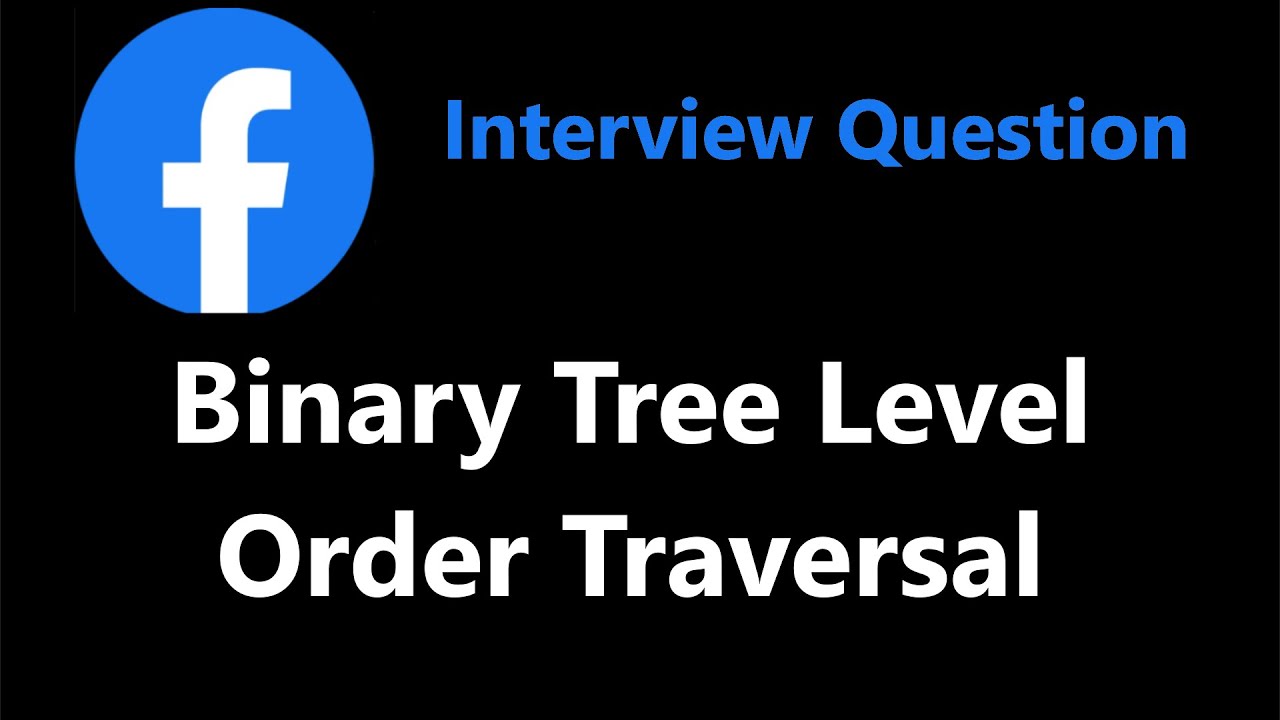
Показать описание
0:00 - Read the problem
leetcode 102
#sorted #array #python
Binary Tree Level Order Traversal - BFS - Leetcode 102
Binary tree: Level Order Traversal
Binary Tree Level Order Traversal
Binary Tree Level Order Traversal (BFS) - Leetcode 102 - Trees (Python)
Leetcode - Binary Tree Level Order Traversal (Python)
LeetCode 102. Binary Tree Level Order Traversal - Interview Prep Ep 70
L8. Level Order Traversal of Binary Tree | BFS | C++ | Java
Binary Tree Level Order Traversal | Live Coding with Explanation | Leetcode - 102
Binary Tree Level Order Traversal - MS-DSA
Level order traversal of Binary Tree | Step by step demo with example | Study Algorithms
Binary Tree Level Order Traversal - LeetCode 102 Python
Level Order Traversal Binary Tree | Binary Tree Level Order Traversal | DSAOne Course #59
Level-order tree traversal in 3 minutes
Level Order Traversal , Sum at Kth Level In Binary Tree | C++ Placement Course | Lecture 27.5
Level order traversal of a Binary Tree in Java
102. Binary Tree Level Order Traversal Java Solution
Leetcode 102. Binary Tree Level Order Traversal [Java]
Binary Tree Level Order Traversal 2 Leetcode C++
Binary Tree Level Order Traversal | LeetCode 102 | C++, Python
Reverse level order traversal binary tree
Level Order Traversal in Binary Tree | Solution | Data Structure and Algorithms | Java and C++
Level Order Traversal | Tree | HackerRank | Python
Binary Trees in Python: Level-order Traversal
Binary Tree Level Order Traversal | Leetcode 102 | Binary Tree
Комментарии